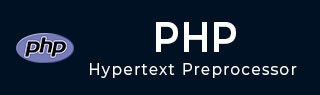
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP – Hashing
The term "hashing" represents a technique of encrypting data (specially a text) to obtain a fixed-length value. PHP library includes a number of functions that can perform hashing on data by applying different hashing algorithms such as md5, SHA2, HMAC etc. The encrypted value obtained is called as the hash of the original key.
Processing of hashing is a one-way process, in the sense, it is not possible to reverse the hash so as to obtain the original key.
Applications of Hashing
The hashing technique is effectively used for the following purposes −
Password Authentication
We often register for various online applications such as gmail, Facebook etc. You are required to fill up a form wherein you create a password for an online account. The server hashes your password and the hashed value is stored in the database. At the time of logging in, the password submitted is hashed and compared with the one in the database. This protects your password from being stolen.
Data Integrity
One of the important uses of hashing is to verify if the data has not been tampered with. When a file is downloaded from the internet, you are shown its hash value, which you can compare with the downloaded to make sure that the file has not been corrupted.
The Process of Hashing
The process of hashing can be represented by the following figure −
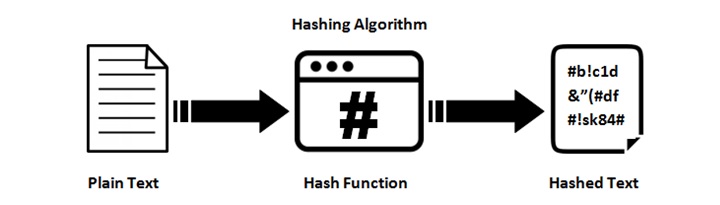
Hashing Algorithms in PHP
PHP supports a number of hashing algorithms −
MD5 − MD5 is a 128-bit hash function that is widely used in software to verify the integrity of transferred files. The 128-bit hash value is typically represented as a 32-digit hexadecimal number. For example, the word "frog" always generates the hash "8b1a9953c4611296a827abf8c47804d7"
SHA − SHA stands for Secure Hash Algorithm. It's a family of standards developed by the National Institute of Standards and Technology (NIST). SHA is a modified version of MD5 and is used for hashing data and certificates. SHA-1 and SHA-2 are two different versions of that algorithm. SHA-1 is a 160-bit hash. SHA-2 is actually a “family” of hashes and comes in a variety of lengths, the most popular being 256-bit.
HMAC − HMAC (Hash-Based Message Authentication Code) is a cryptographic authentication technique that uses a hash function and a secret key.
HKDF − HKDF is a simple Key Derivation Function (KDF) based on the HMAC message authentication code.
PBKDF2 − PBKDF2 (Password-Based Key Derivation Function 2) is a hashing algorithm that creates cryptographic keys from passwords.
Hash Functions in PHP
The PHP library includes several hash functions −
The hash_algos Function
This function returns a numerically indexed array containing the list of supported hashing algorithms.
hash_algos(): array
The hash_file Function
The function returns a string containing the calculated message digest as lowercase hexits.
hash_file( string $algo, string $filename, bool $binary = false, array $options = [] ): string|false
The algo parameter is the type of selected hashing algorithm (i.e. "md5", "sha256", "haval160,4", etc.). The filename is the URL describing location of file to be hashed; supports fopen wrappers.
Example
Take a look at the following example −
<?php /* Create a file to calculate hash of */ $fp=fopen("Hello.txt", "w"); $bytes = fputs($fp, "The quick brown fox jumped over the lazy dog."); fclose($fp); echo hash_file('md5', "Hello.txt"); ?>
It will produce the following output −
5c6ffbdd40d9556b73a21e63c3e0e904
The hash() Function
The hash() function generates a hash value (message digest) −
hash( string $algo, string $data, bool $binary = false, array $options = [] ): string
The algo parameter is the type of selected hashing algorithm (i.e. "md5", "sha256", "haval160,4", etc..). The data parameter is the message to be hashed. If the binary parameter is "true", it outputs raw binary data; "false" outputs lowercase hexits.
Example
The function returns a string containing the calculated message digest as lowercase hexits.
<?php echo "Using SHA256 algorithm:" . hash('sha256', 'The quick brown fox jumped over the lazy dog.'). PHP_EOL; echo "Using MD5 algorithm:",hash('md5', 'The quick brown fox jumped over the lazy dog.'), PHP_EOL; echo "Using SHA1 algorithm:" . hash('sha1', 'The quick brown fox jumped over the lazy dog.'); ?>
It will produce the following output −
Using SHA256 algorithm:68b1282b91de2c054c36629cb8dd447f12f096d3e3c587978dc2248444633483 Using MD5 algorithm:5c6ffbdd40d9556b73a21e63c3e0e904 Using SHA1 algorithm:c0854fb9fb03c41cce3802cb0d220529e6eef94e