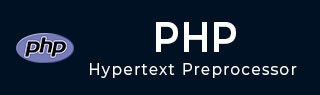
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP – Inheritance
Inheritance is one of the fundamental principles of object-oriented programming methodology. Inheritance is a software modelling approach that enables extending the capability of an existing class to build new class instead of building from scratch.
PHP provides all the functionality to implement inheritance in its object model. Incorporating inheritance in PHP software development results in code reuse, remove redundant code duplication and logical organization.
Imagine that you need to design a new class whose most of the functionality already well defined in an existing class. Inheritance lets you to extend the existing class, add or remove its features and develop a new class. In fact, PHP has the "extends" keyword to establish inheritance relationship between existing and new classes.
class newclass extends oldclass { ... ... }
Inheritance comes into picture when a new class (henceforth will be called inherited class, sub class, child class, etc.) possesses "IS A" relationship with an existing class (which will be called base class, super class, parent class, etc.).
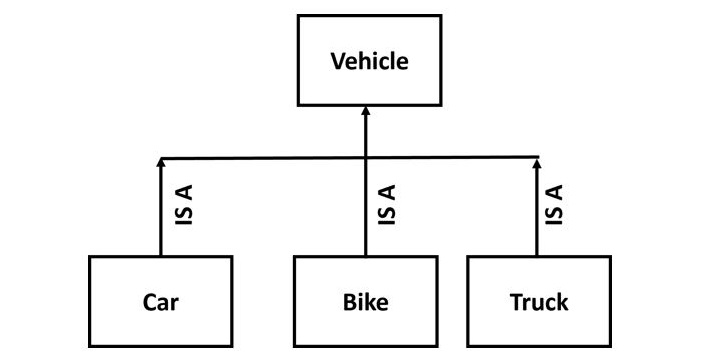
In PHP, when a new class is defined by extending another class, the subclass inherits the public and protected methods, properties and constants from the parent class. You are free to override the functionality of an inherited method, otherwise it will retain its functionality as defined in the parent class.
Example
Take a look at the following example −
<?php class myclass { public function hello() { echo "Hello from the parent class" . PHP_EOL; } public function thanks() { echo "Thank you from parent class" . PHP_EOL; } } class newclass extends myclass { public function thanks() { echo "Thank you from the child class" . PHP_EOL; } } # object of parent class $obj1 = new myclass; $obj1->hello(); $obj1->thanks(); # object of child class $obj2 = new newclass; $obj2->hello(); $obj2->thanks(); ?>
It will produce the following output −
Hello from the parent class Thank you from parent class Hello from the parent class Thank you from the child class
As mentioned before, the child class inherits public and protected members (properties and methods) of the parent. The child class may introduce additional properties or methods.
In the following example, we use the Book class as the parent class. Here, we create an ebook class that extends the Book class. The new class has an additional property – format (indicating ebook’s file format – EPUB, PDF, MOBI etc). The ebook class defines two new methods to initialize and output the ebbok data – getebook() and dispebook() respectively.
Example
The complete code of inheritance example is given below −
<?php class Book { /* Member variables */ protected int $price; protected string $title; public function getbook(string $param1, int $param2) { $this->title = $param1; $this->price = $param2; } public function dispbook() { echo "Title: $this->title Price: $this->price \n"; } } class ebook extends Book { private string $format; public function getebook(string $param1, int $param2, string $param3) { $this->title = $param1; $this->price = $param2; $this->format = $param3; } public function dispebook() { echo "Title: $this->title Price: $this->price\n"; echo "Format: $this->format \n"; } } $eb = new ebook; $eb->getebook("PHP Fundamentals", 450, "EPUB"); $eb->dispebook(); ?>
The browser output is as shown below −
Title: PHP Fundamentals Price: 450 Format: EPUB
If you take a closer look at the getebook() function, the first two assignment statements are in fact there getbook() function, which the ebook class has inherited. Hence, we can call it with parent keyword and scope resolution operator.
Change the getebook() function code with the following −
public function getebook(string $param1, int $param2, string $param3) { parent::getbook($param1, $param2); $this->format = $param3; }
Similarly, the first echo statement in dispebook() function is replaced by a call to the dispbook() function in parent class −
public function dispebook() { parent::dispbook(); echo "Format: $this->format<br/>"; }
Constructor in Inheritance
The constructor in the parent class constructor is inherited by the child class but it cannot be directly called in the child class if the child class defines a constructor.
In order to run a parent constructor, a call to parent::__construct() within the child constructor is required.
Example
Take a look at the following example −
<?php class myclass{ public function __construct(){ echo "This is parent constructor". PHP_EOL; } } class newclass extends myclass { public function __construct(){ parent::__construct(); echo "This is child class destructor" . PHP_EOL; } } $obj = new newclass(); ?>
It will produce the following output −
This is parent constructor This is child class destructor
However, if the child does not have a constructor, then it may be inherited from the parent class just like a normal class method (if it was not declared as private).
Example
Take a look at the following example −
<?php class myclass{ public function __construct(){ echo "This is parent constructor". PHP_EOL; } } class newclass extends myclass{ } $obj = new newclass(); ?>
It will produce the following output −
This is parent constructor
PHP doesn’t allow developing a class by extending more than one parents. You can have hierarchical inheritance, wherein class B extends class A, class C extends class B, and so on. But PHP doesn’t support multiple inheritance where class C tries to extend both class A and class B. We can however extend one class and implement one or more interfaces. We shall learn about interfaces in one of the subsequent chapters.