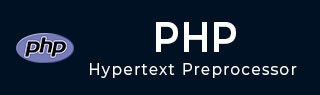
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP – Constructor and Destructor
As in most of the object-oriented languages, you can define a constructor function in a class in PHP also. When you declare an object with the new operator, its member variables are not assigned any value. The constructor function is used to initialize every new object at the time of declaration. PHP also supports having a destructor function that destroys the object from the memory as it no longer has any reference.
The __construct() Function
PHP provides a __construct() function that initializes an object.
__construct(mixed ...$values = ""): void
The constructor method inside a class is called automatically on each newly created object. Note that defining a constructor is not mandatory. However, if present, it is suitable for any initialization that the object may need before it is used.
You can pass as many as arguments you like into the constructor function. The __construct() function doesn’t have any return value.
Let us define a constructor in the Book class used in the previous chapter
<?php class Book { /* Member variables */ var $price; var $title; /*Constructor*/ function __construct(){ $this->title = "PHP Fundamentals"; $this->price = 275; } /* Member functions */ function getPrice() { echo "Price: $this->price \n"; } function getTitle(){ echo "Title: $this->title \n"; } } $b1 = new Book; $b1->getTitle(); $b1->getPrice(); ?>
It will produce the following output −
Title: PHP Fundamentals Price: 275
Parameterized Constructor
The member variables of $b1 have been initialized without having to call setTitle() and setPrice() methods, because the constructor was called as soon as the object was declared. However, this constructor will be called for each object, and hence each object has the same values of title and price properties.
To initialize each object with different values, define the __construct() function with parameters.
Change the definition of __construct() function to the following −
function __construct($param1, $param2) { $this->title = $param1; $this->price = $param2; }
To initialize the object, pass values to the parameters inside a parenthesis in the declaration.
$b1 = new Book("PHP Fundamentals", 375);
Example
Now, you can have each object with different values to the member variables.
<?php class Book { /* Member variables */ var $price; var $title; /*Constructor*/ function __construct($param1, $param2) { $this->title = $param1; $this->price = $param2; } /* Member functions */ function getPrice(){ echo "Price: $this->price \n"; } function getTitle(){ echo "Title: $this->title \n"; } } $b1 = new Book("PHP Fundamentals", 375); $b2 = new Book("PHP Programming", 450); $b1->getTitle(); $b1->getPrice(); $b2->getTitle(); $b2->getPrice(); ?>
It will produce the following output −
Title: PHP Fundamentals Price: 375 Title: PHP Programming Price: 450
Constructor Overloading
Method overloading is an important concept in object-oriented programming, where a class may have more than one definitions of constructor, each having different number of arguments. However, PHP doesn’t support method overloading. This limitation may be overcome by using arguments with default values in the constructor function.
Change the __construct() function to the following −
function __construct($param1="PHP Basics", $param2=380) { $this->title = $param1; $this->price = $param2; }
Now, declare an object without passing parameters, and the other with parameters. One without parameters will be initialized with default arguments, the other with the values passed.
$b1 = new Book(); $b2 = new Book("PHP Programming", 450);
It will produce the following output −
Title: PHP Basics Price: 380 Title: PHP Programming Price: 450
Type Declaration in Constructor
Since PHP (version 7.0 onwards) allows scalar type declarations for function arguments, the __construct() function may be defined as −
function __construct(string $param1="PHP Basics", int $param2=380) { $this->title = $param1; $this->price = $param2; }
In the earlier versions of PHP, using the name of class to define a constructor function was allowed, but this feature has been deprecated since PHP version 8.0.
The __destruct() Function
PHP also has a __destructor() function. It implements a destructor concept similar to that of other object-oriented languages, as in C++. The destructor method will be called as soon as there are no other references to a particular object.
__destruct(): void
The __destruct() function doesn’t have any parameters, neither does it have any return value. The fact that the __destruct() function is automatically called when any object goes out of scope, can be verified by putting var_dump($this) inside the function.
As mentioned above, $this carries the reference to the calling object, the dump shows that the member variables are set to NULL
Add destructor function in the Book class as follows −
function __destruct() { var_dump($this); echo "object destroyed"; }
As the program exits, the following output will be displayed −
object(Book)#1 (2) { ["price"]=> NULL ["title"]=> NULL } object destroyed