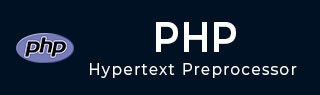
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Post-Redirect-Get (PRG)
In PHP, PRG stands for "Post/Redirect/Get". It is a commonly used technique that is designed to prevent the resubmission of a form after it's been submitted. You can easily implement this technique in PHP to avoid duplicate form submissions.
Usually a HTML form sends data to the server with the POST method. The server script fetches the data for further processing like adding a new record in a backend database, or running a query to fetch data. If the user accidentally refreshes the browser, there is a possibility of the same form data being resubmitted again, possibly leading to loss of data integrity. The PRG approach in PHP helps you avoid this pitfall.
Example
To start with, let us consider the following PHP script that renders a simple HTML form, and submits it back to itself with POST method. When the user fills the data and submits, the backend script fetches the data, renders the result, and comes back to show the blank form again.
<?php if (isset($_POST["submit"])) { if ($_SERVER["REQUEST_METHOD"] == "POST") echo "First name: " . $_REQUEST['first_name'] . " " . "Last Name: " . $_REQUEST['last_name'] . ""; } ?> <html> <body> <form action="<?php echo $_SERVER['PHP_SELF'];?>" method="post"> First Name: <input type="text" name="first_name"> <br/> Last Name: <input type="text" name="last_name" /> <button type="submit" name="submit">Submit</button> </form> </body> </html>
Assuming that the server is running, the above script is placed in the document root folder and visited in the browser.
Fill the data and submit. The browser echoes the result, and re-renders the form. Now if you try to refresh the browser page, a warning pops up as shown below −
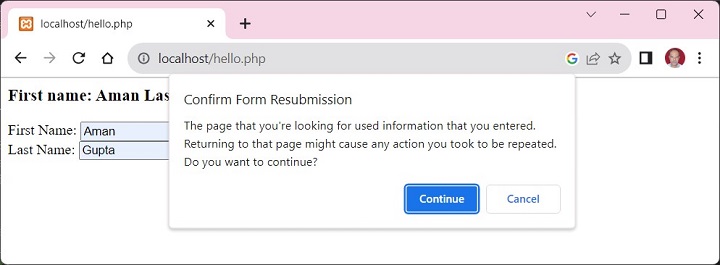
If you press Continue, the same data is posted again.
The problem can be understood with the following figure −
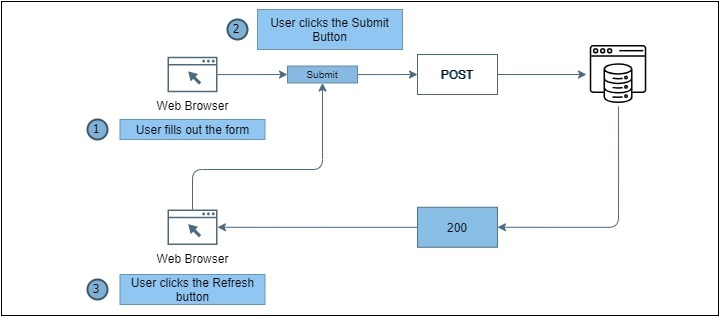
Following steps are taken in the PHP script to avoid the problem −
The PHP script before the HTML form starts a new session.
Check if the form has been submitted with POST method.
If so, store the form data in session variables
Redirect the browser to a result page. In our case, it is the same page. With the exit command, to terminate this script to make sure no more code gets executed.
If PHP finds that the REQUEST method is not POST, it checks if the session variables are set. If so, they are rendered along with the fresh copy of form.
Now even if the form is refreshed, you have successfully averted the possibility of resubmission.
Example
Here is the PHP code that uses the PRG technique −
<?php session_start(); if (isset($_POST["submit"])) { $_SESSION['fname'] = $_POST['first_name']; $_SESSION['lname'] = $_POST['last_name']; header("Location: hello.php"); exit; } if (isset($_SESSION["fname"])) { echo "First name: " . $_SESSION['fname'] . " " . "Last Name: " . $_SESSION['lname'] . ""; unset($_SESSION["fname"]); unset($_SESSION["lname"]); } ?> <html> <body> <form action="<?php echo $_SERVER['PHP_SELF'];?>" method="post"> First Name: <input type="text" name="first_name"> <br /> Last Name: <input type="text" name="last_name" /> <button type="submit" name="submit">Submit</button> </form> </body> </html>