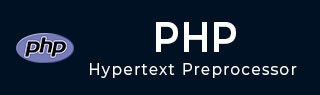
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP – Swapping Variables
PHP doesn’t provide any built-in function with which you can swap or interchange values of two variables. However, there are a few techniques which you can use to perform the swap.
One of the most straightforward approaches is to use a third variable as a temporary place holder to facilitate swapping. Using the arithmetic operators in a specific order also is very effective. You can also use the binary XOR operator for swapping purpose. In this chapter, we shall implement these swapping techniques in PHP
Temporary Variable
This is logically the most obvious and the simplest approach. To swap values of "a" and "b", use a third variable "c". Assign the value of "a" to "c", overwrite "a" with existing value of "b" and then set "b" to the earlier value of "a" that was stored in "c".
Example
Take a look at the following example −
<?php $a = 10; $b = 20; echo "Before swapping - \$a = $a, \$b = $b". PHP_EOL; $c = $a; $a = $b; $b = $c; echo "After swapping - \$a = $a, \$b = $b". PHP_EOL; ?>
It will produce the following output −
Before swapping - $a = 10, $b = 20 After swapping - $a = 20, $b = 10
Using addition (+) Operator
This solution takes the advantage of the fact that subtracting a number from the sum of two numbers gives back the second number. In other words, "sum(a+b) – a" is equal to "b" and vice versa.
Example
Let us take advantage of this property to swap "a" and "b" −
<?php $a = 10; $b = 20; echo "Before swapping - \$a = $a, \$b = $b". PHP_EOL; $a = $a + $b; $b = $a - $b; $a = $a - $b; echo "After swapping - \$a = $a, \$b = $b". PHP_EOL; ?>
It will produce the following output −
Before swapping - $a = 10, $b = 20 After swapping - $a = 20, $b = 10
You can also use the other arithmetic operators – subtraction (-), multiplication (*) and division (/) in a similar manner to perform swapping.
Using list() Function
The list() function in PHP unpacks the array in separate variables. This helps in our objective of performing swap between two variables. To do that, build an array of "a" and "b", and then unpack it to "b" and "a" variables to obtain "a" and "b" with interchanged values.
Example
Take a look at the following example −
<?php $a = 10; $b = 20; echo "Before swapping - \$a = $a, \$b = $b". PHP_EOL; $arr = [$a, $b]; list($b, $a) = $arr; echo "After swapping - \$a = $a, \$b = $b". PHP_EOL; ?>
It will produce the following output −
Before swapping - $a = 10, $b = 20 After swapping - $a = 20, $b = 10
Bitwise XOR
The bitwise XOR (^) operator can also be used to swap the value of two variables "x" and "y". It returns 1 when one of two bits at same position in both operands is 1, otherwise returns 0.
Example
Take a look at the following example −
<?php $a = 10; $b = 20; echo "Before swapping - \$a = $a, \$b = $b". PHP_EOL; $a = $a ^ $b; $b = $a ^ $b; $a = $a ^ $b; echo "After swapping - \$a = $a, \$b = $b". PHP_EOL; ?>
It will produce the following output −
Before swapping - $a = 10, $b = 20 After swapping - $a = 20, $b = 10