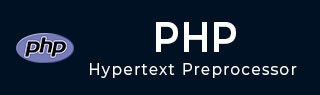
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Heredoc & Nowdoc
PHP provides two alternatives for declaring single or double quoted strings in the form of heredoc and newdoc syntax.
The single quoted string doesn’t interpret the escape characters and doesn’t expand the variables.
On the other hand, if you declare a double quoted string that contains a double quote character itself, you need to escape it by the "\" symbol. The heredoc syntax provides a convenient method.
Heredoc Strings in PHP
The heredoc strings in PHP are much like double-quoted strings, without the double-quotes. It means that they don’t need to escape quotes and expand variables.
Heredoc Syntax
$str = <<<IDENTIFIER place a string here it can span multiple lines and include single quote ' and double quotes " IDENTIFIER;
First, start with the "<<<" operator. After this operator, an identifier is provided, then a newline. The string itself follows, and then the same identifier again to close the quotation. The string can span multiple lines and includes single quotes (‘) or double quotes (").
The closing identifier may be indented by space or tab, in which case the indentation will be stripped from all lines in the doc string.
Example
The identifier must contain only alphanumeric characters and underscores and start with an underscore or a non-digit character. The closing identifier should not contain any other characters except a semicolon (;). Furthermore, the character before and after the closing identifier must be a newline character only.
Take a look at the following example −
<?php $str1 = <<<STRING Hello World PHP Tutorial by TutorialsPoint STRING; echo $str1; ?>
It will produce the following output −
Hello World PHP Tutorial by TutorialsPoint
Example
The closing identifier may or may not contain indentation after the first column in the editor. Indentation, if any, will be stripped off. However, the closing identifier must not be indented further than any lines of the body. Otherwise, a ParseError will be raised. Take a look at the following example and its output −
<?php $str1 = <<<STRING Hello World PHP Tutorial by TutorialsPoint STRING; echo $str1; ?>
It will produce the following output −
PHP Parse error: Invalid body indentation level (expecting an indentation level of at least 16) in hello.php on line 3
Example
The quotes in a heredoc do not need to be escaped, but the PHP escape sequences can still be used. Heredoc syntax also expands the variables.
<?php $lang="PHP"; echo <<<EOS Heredoc strings in $lang expand vriables. The escape sequences are also interpreted. Here, the hexdecimal ASCII characters produce \x50\x48\x50 EOS; ?>
It will produce the following output −
Heredoc strings in PHP expand vriables. The escape sequences are also interpreted. Here, the hexdecimal ASCII characters produce PHP
Nowdoc Strings in PHP
A nowdoc string in PHP is similar to a heredoc string except that it doesn’t expand the variables, neither does it interpret the escape sequences.
<?php $lang="PHP"; $str = <<<'IDENTIFIER' This is an example of Nowdoc string. it can span multiple lines and include single quote ' and double quotes " IT doesn't expand the value of $lang variable IDENTIFIER; echo $str; ?>
It will produce the following output −
This is an example of Nowdoc string. it can span multiple lines and include single quote ' and double quotes " IT doesn't expand the value of $lang variable
The nowdoc’s syntax is similar to the heredoc’s syntax except that the identifier which follows the "<<<" operator needs to be enclosed in single quotes. The nowdoc’s identifier also follows the rules for the heredoc identifier.
Heredoc strings are like double-quoted strings without escaping. Nowdoc strings are like single-quoted strings without escaping.