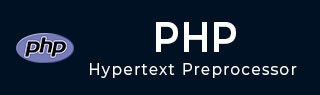
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP mysqli_stmt_affected_rows() Function
Definition and Usage
The mysqli_stmt_affected_rows() function returns the number of rows affected (changed, deleted, inserted) by the recently executed statement.
This function works fine only if invoked after INSERT, UPDATE, or DELETE statements. If you need to know the number of rows affected by the SELECT query you need to use the mysqli_stmt_num_rows() function.
Syntax
mysqli_stmt_affected_rows($stmt)
Parameters
Sr.No | Parameter & Description |
---|---|
1 |
stmt(Mandatory) This is an object representing a statement executing a SQL query. |
Return Values
PHP mysqli_stmt_affected_rows() function returns an integer value indicating the number of rows affected by the previous (INSERT, UPDATE, REPLACE or DELETE) operation.
If the statement has an error this function returns -1. If there are no affected rows, this function returns 0.
PHP Version
This function was first introduced in PHP Version 5 and works works in all the later versions.
Example
Assume we have created a table named employee in the MySQL database with the following contents $minus;
mysql> select * from employee; +------------+--------------+------+------+--------+ | FIRST_NAME | LAST_NAME | AGE | SEX | INCOME | +------------+--------------+------+------+--------+ | Vinay | Bhattacharya | 20 | M | 21000 | | Sharukh | Sheik | 25 | M | 23300 | | Trupthi | Mishra | 24 | F | 51000 | | Sheldon | Cooper | 25 | M | 2256 | | Sarmista | Sharma | 28 | F | 15000 | +------------+--------------+------+------+--------+ 5 rows in set (0.00 sec)
Following example demonstrates the usage of the mysqli_stmt_affected_rows() function (in procedural style) −
<?php $con = mysqli_connect("localhost", "root", "password", "mydb"); $stmt = mysqli_prepare($con, "UPDATE employee set INCOME=INCOME-? where INCOME>=?"); mysqli_stmt_bind_param($stmt, "si", $reduct, $limit); $limit = 20000; $reduct = 5000; //Executing the statement mysqli_stmt_execute($stmt); print("Records Updated......\n"); //Affected rows $count = mysqli_stmt_affected_rows($stmt); //Closing the statement mysqli_stmt_close($stmt); //Closing the connection mysqli_close($con); print("Rows affected ".$count); ?>
This will produce following result −
Records Updated...... Rows affected 3
Example
In object oriented style the syntax of this function is $con->affected_rows; Following is the example of this function in object oriented style $minus;
<?php //Creating a connection $con = new mysqli("localhost", "root", "password", "mydb"); $con -> query("CREATE TABLE Test(Name VARCHAR(255), AGE INT)"); print("Table Created.....\n"); $con -> query("insert into Test values('Raju', 25),('Rahman', 30),('Sarmista', 27)"); print("Records Inserted.....\n"); $stmt = $con -> prepare( "DELETE FROM Test WHERE Name in(?, ?)"); $stmt -> bind_param("ss", $name1, $name2); $name1 = 'Raju'; $name2 = 'Rahman'; print("Records Deleted.....\n"); //Executing the statement $stmt->execute(); //Affected rows $count = $stmt ->affected_rows; print("Rows affected ".$count); //Closing the statement $stmt->close(); //Closing the connection $con->close(); ?>
This will produce following result −
Table Created..... Records Inserted..... Records Deleted..... Rows affected 2
Example
Let's check the return values of this if the query does not effect any rows −
<?php $con = @mysqli_connect("localhost", "root", "password", "mydb"); mysqli_query($con, "CREATE TABLE Test(Name VARCHAR(255), AGE INT)"); print("Table Created.....\n"); mysqli_query($con, "insert into Test values('Raju', 25),('Rahman', 30),('Sarmista', 27)"); print("Records Inserted.....\n"); $stmt = mysqli_prepare($con, "DELETE FROM test where Age<?"); mysqli_stmt_bind_param($stmt, "i", $num); $num = 8; //Executing the statement mysqli_stmt_execute($stmt); //Affected rows $count = mysqli_stmt_affected_rows($stmt); print("Rows affected (when query does nothing): ".$count); //Closing the statement mysqli_stmt_close($stmt); //Closing the connection mysqli_close($con); ?>
This will produce following result −
Table Created..... Records Inserted..... Rows affected (when query does nothing): 0