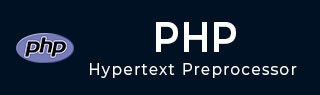
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP mysqli_rollback() Function
Definition and Usage
MySQL database have a feature named auto-commit if you turn it on, the changes done in the databases are saved automatically and, If you turn it off, you need to save the changes explicitly using the mysqli_commit() function.
The mysqli_rollback() function rolls the current transaction to the last save point (or the specified save point).
Syntax
mysqli_rollback($con, [$flags, $name]);
Parameters
Sr.No | Parameter & Description |
---|---|
1 |
con(Mandatory) This is an object representing a connection to MySQL Server. |
2 |
flags(Optional) A constant which can be on of the following :
|
3 |
name(Optional) This is a name value which when given, executes as ROLLBACK/*name*/ . |
Return Values
The PHP mysqli_rollback() function returns a boolean value which is, true if the operation is successful and, false if not.
PHP Version
This function was first introduced in PHP Version 5 and works in all the later versions.
Example
Following example demonstrates the usage of the mysqli_rollback() function (in procedural style) −
<?php //Creating a connection $con = mysqli_connect("localhost", "root", "password", "mydb"); //Setting auto commit to false mysqli_autocommit($con, False); mysqli_query($con, "CREATE TABLE IF NOT EXISTS my_team(ID INT, First_Name VARCHAR(255), Last_Name VARCHAR(255), Place_Of_Birth VARCHAR(255), Country VARCHAR(255))"); //Inserting a records into the my_team table mysqli_query($con, "insert into my_team values(1, 'Shikhar', 'Dhawan', 'Delhi', 'India')"); mysqli_query($con, "insert into my_team values(2, 'Jonathan', 'Trott', 'CapeTown', 'SouthAfrica')"); mysqli_query($con, "insert into my_team values(3, 'Kumara', 'Sangakkara', 'Matale', 'Srilanka')"); mysqli_query($con, "insert into my_team values(4, 'Virat', 'Kohli', 'Delhi', 'India')"); $res = mysqli_query($con, "SELECT * FROM my_team"); print("No.of rows (at the time of commit): ".mysqli_affected_rows($con)."\n"); //Saving the changes mysqli_commit($con); //Truncating the table mysqli_query($con, "DELETE FROM my_team where id in(3,4)"); $res = mysqli_query($con, "SELECT * FROM my_team"); print("No.of rows (before roll back): ".mysqli_affected_rows($con)."\n"); //Roll back mysqli_rollback($con); //Contents of the table $res = mysqli_query($con, "SELECT * FROM my_team"); print("No.of rows (after roll back): ".mysqli_affected_rows($con)); //Closing the connection mysqli_close($con); ?>
This will produce following result −
No.of rows (at the time of commit): 4 No.of rows (before roll back): 2 No.of rows (after roll back): 4
Example
The syntax of this method in object oriented style is $con->rollback(). Following is an example of this function in object oriented mode $minus;
//Creating a connection $con = new mysqli("localhost", "root", "password", "mydb"); //Setting auto commit to false $con->autocommit(FALSE); //Inserting a records into the players table $con->query("CREATE TABLE IF NOT EXISTS players(First_Name VARCHAR(255), Last_Name VARCHAR(255), Country VARCHAR(255))"); $con->query("insert into players values('Shikhar', 'Dhawan', 'India')"); $con->query("insert into players values('Jonathan', 'Trott', 'SouthAfrica')"); //Saving the results $con->commit(); $con->query( "insert into players values('Kumara', 'Sangakkara', 'Srilanka')"); $con->query( "insert into players values('Virat', 'Kohli', 'India')"); //Roll back $con->rollback(); $res = $con->query("SELECT * FROM players"); print_r($res); //Closing the connection $res = $con -> close(); ?>
This will produce following result −
mysqli_result Object ( [current_field] => 0 [field_count] => 3 [lengths] => [num_rows] => 2 [type] => 0 )
Example
<?php $connection = mysqli_connect("localhost", "root", "password", "mydb"); if (mysqli_connect_errno($connection)){ echo "Failed to connect to MySQL: " . mysqli_connect_error(); } mysqli_autocommit($connection,FALSE); mysqli_query($connection, "create table test(Name VARCHAR(255), Age INT)"); mysqli_query($connection, "INSERT INTO test VALUES ('Sharukh', 25)"); mysqli_commit($connection); mysqli_query($connection, "INSERT INTO test VALUES ('Kalyan', 30)"); mysqli_rollback($connection); mysqli_close($connection); ?>
After executing the above program if you verify, the contents of the table test, you can see the inserted records as −
mysql> select * from test; +---------+------+ | Name | Age | +---------+------+ | Sharukh | 25 | +---------+------+ 1 row in set (0.00 sec)