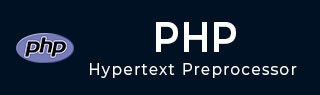
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP date_parse_from_format() Function
Definition and Usage
The date_parse_from_format() function accepts a format string and a date string as parameters and, returns information about the given date in the specified format.
Syntax
date_parse($date)
Parameters
Sr.No | Parameter & Description |
---|---|
1 |
format(Mandatory) This is a string value representing the format in which you need to format the info about the date. |
2 |
date(Mandatory) This is a string value representing the date for which you need the information about. |
Return Values
PHP date_create_from_format() function returns array holding the information about the given date in the specified format.
PHP Version
This function was first introduced in PHP Version 5.3.0 and, works with all the later versions.
Example
Following example demonstrates the usage of the date_parse_from_format() function −
<?php //Creating a DateTime object $date = "25-Mar-1989"; $format = "d-M-Y"; $res = date_parse_from_format($format, $date); print_r($res); ?>
This will produce following result −
Array ( [year] => 1989 [month] => 3 [day] => 25 [hour] => [minute] => [second] => [fraction] => [warning_count] => 0 [warnings] => Array ( ) [error_count] => 0 [errors] => Array ( ) [is_localtime] => )
Example
Let us see different formats to parse a date −
<?php $res1 = date_parse_from_format("j.n.Y", "25.8.2014"); print_r($res1); $res2 = date_parse_from_format("y-d-m", "2014-25-8"); print_r($res2); $res3 = date_parse_from_format("n/j/y", "8/25/2014"); print_r($res3); $res4 = date_parse_from_format("D.M.Y", "25.8.2014"); print_r($res4); $res5 = date_parse_from_format("H/i/s", "12/32/25"); print_r($res5); ?>
This will produce following result −
Array ( [year] => 2014 [month] => 8 [day] => 25 [hour] => [minute] => [second] => [fraction] => [warning_count] => 0 [warnings] => Array ( ) [error_count] => 0 [errors] => Array ( ) [is_localtime] => ) Array ( [year] => 2020 [month] => 25 [day] => 14 [hour] => [minute] => [second] => [fraction] => [warning_count] => 1 [warnings] => Array ( [7] => The parsed date was invalid ) [error_count] => 2 [errors] => Array ( [2] => The separation symbol could not be found [7] => Trailing data ) [is_localtime] => ) Array ( [year] => 2020 [month] => 8 [day] => 25 [hour] => [minute] => [second] => [fraction] => [warning_count] => 0 [warnings] => Array ( ) [error_count] => 1 [errors] => Array ( [7] => Trailing data ) [is_localtime] => ) Array ( [year] => 8 [month] => [day] => [hour] => [minute] => [second] => [fraction] => [warning_count] => 0 [warnings] => Array ( ) [error_count] => 4 [errors] => Array ( [0] => A textual day could not be found [3] => The separation symbol could not be found [4] => Trailing data ) [is_localtime] => ) Array ( [year] => [month] => [day] => [hour] => 12 [minute] => 32 [second] => 25 [fraction] => 0 [warning_count] => 0 [warnings] => Array ( ) [error_count] => 0 [errors] => Array ( ) [is_localtime] => )
Example
Following example demonstrates date_parse_from_format() with relative formats −
<?php print_r(date_parse_from_format("Y-m-d", "2009-18-18-+52 week +25 hour")); print("\n"); print_r(date_parse_from_format("Y-m-d", "1990-06-06 +52 week +25 hour")); ?>
This will produce the following output −
Array ( [year] => 2009 [month] => 18 [day] => 18 [hour] => [minute] => [second] => [fraction] => [warning_count] => 1 [warnings] => Array ( [10] => The parsed date was invalid ) [error_count] => 1 [errors] => Array ( [10] => Trailing data ) [is_localtime] => ) Array ( [year] => 1990 [month] => 6 [day] => 6 [hour] => [minute] => [second] => [fraction] => [warning_count] => 0 [warnings] => Array ( ) [error_count] => 1 [errors] => Array ( [10] => Trailing data ) [is_localtime] => )