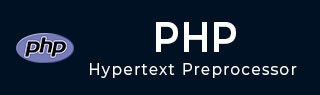
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP Deque Functions
The Deque is a sequence of values in a contiguous buffer that can grow and shrink automatically. It is a common abbreviation of “double-ended queue” and can be used internally by Ds\Queue.
Two pointers can be used to keep track of a head and tail. The pointers can wrap around the end of a buffer that avoids the need to move other values around to make room. This can make a shift and unshift very fast.
Accessing a value by index can require a translation between an index and its corresponding position in the buffer: ((head + position) % capacity).
Strengths
- Supports array syntax (square brackets).
- Uses less overall memory than an array for the same number of values.
- Automatically frees allocated memory when its size drops low enough.
- get(), set(), push(), pop(), shift(), and unshift() are all O(1).
Weaknesses
- Capacity must be a power of 2.
- insert() and remove() are O(n).
Syntax
Ds\Deque implements Ds\Sequence { /* Constants */ const int MIN_CAPACITY = 8 ; /* Methods */ public void allocate( int $capacity ) public void apply( callable $callback ) public int capacity( void ) public void clear( void ) public bool contains([ mixed $...values ] ) public Ds\Deque copy( void ) public Ds\Deque filter([ callable $callback ] ) public mixed find( mixed $value ) public mixed first( void ) public mixed get( int $index ) public void insert( int $index [, mixed $...values ] ) public bool isEmpty( void ) public string join([ string $glue ] ) public mixed last( void ) public Ds\Deque map( callable $callback ) public Ds\Deque merge( mixed $values ) public mixed pop( void ) public void push([ mixed $...values ] ) public mixed reduce( callable $callback [, mixed $initial ] ) public mixed remove( int $index ) public void reverse( void ) public Ds\Deque reversed( void ) public void rotate( int $rotations ) public void set( int $index , mixed $value ) public mixed shift( void ) public Ds\Deque slice( int $index [, int $length ] ) public void sort([ callable $comparator ] ) public Ds\Deque sorted([ callable $comparator ] ) public number sum( void ) public array toArray( void ) public void unshift([ mixed $values ] ) }
Predefined Constants
Ds\Deque::MIN_CAPACITY
Sr.No | Function & Description |
---|---|
1 |
This function can allocate enough memory for a required capacity. |
2 |
This function can update all values by applying a callback function to each value. |
3 |
This function can return the current capacity. |
4 |
This function can remove all values from the deque. |
5 |
This function can determine if the deque contains given values. |
6 | Ds\Deque::__construct() Function This function can create a new instance. |
7 |
This function can return a shallow copy of the deque. |
8 |
This function can be used to get the number of elements in the Deque. |
9 |
This function can create a new deque by using the callable to determine which values to include. |
10 |
This function can attempt to find a value's index. |
11 |
This function can return the first value in the deque. |
12 |
This function can return the value at a given index. |
13 |
This function can insert the values at a given index. |
14 |
This function can return whether the deque is empty. |
15 |
This function can join all values together as a string. |
16 | Ds\Deque::jsonSerialize() Function This function can return a representation that can be converted to JSON. |
17 |
This function can return the last value. |
18 |
This function can return the result of applying a callback to each value. |
19 |
This function can return the result of adding all given values to the deque. |
20 |
This function can remove and return the last value. |
21 |
This function can add values to an end of the deque. |
22 |
This function can reduce the deque to a single value using a callback function. |
23 |
This function can remove and return a value by index. |
24 |
This function can reverse the deque in-place. |
25 |
This function can return a reversed copy. |
26 |
This function can rotate the deque by a given number of rotations. |
27 |
This function can update the value at a given index. |
28 |
This function can remove and return the first value. |
29 |
This function can return the sub-deque of a given range. |
30 |
This function can sort the deque in-place. |
31 |
This function can return a sorted copy. |
32 |
This function can return the sum of all values in the deque. |
33 |
This function can convert the deque to an array. |
34 |
This function can add values to front of the deque. |