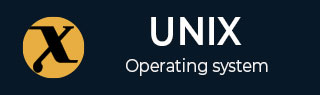
- Unix Commands Reference
- Unix - Tutorial Home
bc - Unix, Linux Command
NAME
bc - An arbitrary precision calculator language.
SYNOPSIS
bc options file...
DESCRIPTION
bc is a language to supports arbitrary precision numbers with interactive execution of statements. It starts by processing code from all the files listed on the command line in the order they are listed. After all files have been processed, bc starts reading from the standard input. All code is executed as it is read.
bc is usually used within a shell script, using a "here" document to pass the program details to bc.
OPTIONS
Tag | Description |
---|---|
--help | Display help |
-h, --help | Print the usage and exit. |
file | A file containing the calculations/functions to perform. This can be piped from standard input. |
-i, --interactive | Force interactive mode. |
-l, --mathlib | Define the standard math library. |
-w, --warn | Give warnings for extensions to POSIX bc. |
-s, --standard | Process exactly the POSIX bc language. |
-q, --quiet | Do not print the normal GNU bc welcome. |
-v, --version | Print the version number and copyright and quit. |
EXAMPLES
Create a Utility Class to use bc.
#bcsample if [ $# != 1 ] then echo "A number argument is required" exit fi bc <<END-OF-INPUT scale=6 /* first we define the function */ define myfunc(x){ return(sqrt(x) + 10); } /* then use the function to do the calculation*/ x=$1 "Processing";x;" result is ";myfunc(x) quit END-OF-INPUT echo "(to 6 decimal places)"
Run the utility
$ chmod a+x bcsample $ ./bcsample 25 Processing 25 result is 15
Standard functions supported by bc
Function | Description |
---|---|
length ( expression ) | The value of the length function is the number of significant digits in the expression. |
read ( ) | Read a number from the standard input, regardless of where the function occurs. Beware, this can cause problems with the mixing of data and program in the standard input. The best use for this function is in a previously written program that needs input from the user, but never allows program code to be input from the user. |
scale ( expression ) | The number of digits after the decimal point in the expression. |
sqrt ( expression ) | The number of digits after the decimal point in the expression. |
++ var | increment the variable by one and set the new value as the result of the expression. |
-var ++ | The result of the expression is the value of the variable and the variable is then incremented by one. |
-- var | decrement the variable by one and set the new value as the result of the expression. |
var -- | The result of the expression is the value of the variable and the variable is then decremented by one. |
( expr ) | Parenthesis alter the standard precedence to force the evaluation of an expression. |
var = expr | The variable var is assigned the value of the expression. |
Most standard math expressions like + - / * % ^, relational expressions and boolean operations are supported.
Comments
/* In-line comments */ - Used for inline comments
# single line comment. - Used for single line comments. The end of line character is not considered as a part of the comment and is processed normally.
Print