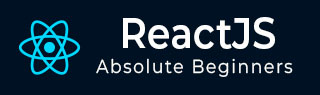
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - mockComponent()
When we use a library like React in our apps, we should regularly test our components. However, checking them out can be difficult at times because components may rely on other components. This is wherein the mockComponent() function comes in.
Assume we have a code component that has a button or a form that we want to check, but it is dependent on other components. We can use mockComponent() to create a fake model of those other components so that we can test our code without actually using them.
Syntax
mockComponent( componentClass, [mockTagName] )
Parameters
componentClass − This is the component we want to mock. It is the class or module that we want to create a fake version of for testing purposes.
[mockTagName] (Optional) − This parameter is in square brackets, which means it is not required. If we provide this parameter, it specifies the HTML tag name that the fake component will mimic. If we do not provide it, the default tag name is <div>.
Return Value
The mockComponent function returns a mock or fake version of the given component class. This allows us to isolate and test our component without actually using its dependencies.
How to use the mockComponent() function?
We call mockComponent() and give it the component we want to test. We can also tell it what kind of HTML tag the fake component should look like (usually a <div>). Any content that would normally be inside the real component will be put inside the fake one.
So, mockComponent() is like making a pretend version of a component to help us test our code. It is important to note that there are newer and better ways to do this in React, like using jest.mock(), but mockComponent() is an older method that we might still come across in some code.
Examples
Example − Mocking Button Component
Here is an example of how we can use mockComponent() in React −
Let us say we have a React component named Button.js, and we want to test it. This component depends on another component called Icon.js, which we want to mock using mockComponent().
Button.js
import React from 'react'; import Icon from './Icon'; function Button() { return ( <button> <Icon name="star" /> Click me </button> ); } export default Button;
Now, we can create a test for the Button component with the help of mockComponent() to mock the Icon component. So we can do it with a test file, let us call it Button.test.js −
Button.test.js
import React from 'react'; import { shallow } from 'enzyme'; // Use Enzyme for testing import { mockComponent } from 'react-dom/test-utils'; // Importing mockComponent import Button from './Button'; // Mock the Icon component const MockedIcon = mockComponent(Icon); test('Button renders with a mocked Icon', () => { const wrapper = shallow(<Button />); expect(wrapper.contains(<MockedIcon name="star" />)).toBeTruthy(); });
In this example, we import mockComponent from react-dom/test-utils and use mockComponent(Icon) to create a mocked version of the Icon component. The mocked version is then used in our test for the Button component.
Example − Mocking a Form Component
We will define a simple React app where a Form component is rendered, and we will use mockComponent to create a mock version of the Form component for testing. The Form component takes a prop onSubmit for handling form submissions.
And our testing app tests that the form is rendered, and it can simulate form submission by interacting with the input field and the submit button. Here the mockComponent function is used to create a mock version of the Form component for testing purposes.
Form.js
import React from 'react'; const Form = ({ onSubmit }) => ( <form onSubmit={onSubmit}> <input type="text" /> <button type="submit">Submit</button> </form> ); export default Form;
App.js
import React from 'react'; import { render } from '@testing-library/react'; import { mockComponent } from 'your-testing-library'; // Replace with testing library import Form from './Form'; const MockedForm = mockComponent(Form, 'div'); test('renders a form', () => { const { getByText } = render(<MockedForm />); const submitButton = getByText('Submit'); expect(submitButton).toBeInTheDocument(); });
Example − Mocking Parent Component with Child Component
This React app consists of a ParentComponent that renders a child component called ChildComponent. For testing purposes, the ChildComponent is replaced with a mocked version using the mockComponent function. Let's see the app below −
ParentComponent.js
import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => ( <div> <p>This is a parent component</p> <ChildComponent /> </div> ); export default ParentComponent;
App.js
import React from 'react'; import { render } from '@testing-library/react'; import { mockComponent } from 'your-testing-library'; // Replace with testing library import ParentComponent from './ParentComponent'; const MockedChildComponent = mockComponent(() => <div>Mocked Child</div>, 'div'); const MockedParentComponent = mockComponent(ParentComponent, 'div'); test('renders a parent component with a mocked child', () => { const { getByText } = render(<MockedParentComponent />); const parentText = getByText('This is a parent component'); const mockedChildText = getByText('Mocked Child'); expect(parentText).toBeInTheDocument(); expect(mockedChildText).toBeInTheDocument(); });
Summary
mockComponent() is a useful tool for simplifying React component testing by producing mock versions of dependent components. It allows effective testing by making sure the tests target the single component's functionality rather than its dependencies. There are alternatives to mockComponent(), like jest.mock(), mockComponent() remains a valuable legacy API for testing React components.
To Continue Learning Please Login