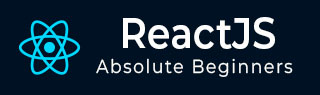
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - Context
Context is one of the important concept in React. It provides the ability to pass a information from the parent component to all its children to any nested level without passing the information through props in each level. Context will make the code more readable and simple to understand. Context can be used to store information which does not change or have minimal change. Some of the use cases of context are as follows −
Application configuration
Current authenticated user information
Current user setting
Language setting
Theme / Design configuration by application / users
Let us learn how to create context and its usage in this chapter.
How context works?
Let us learn the basic concept of context and how it works. Context has four parts,
Creating a new context
Setting context provider in the root component
Setting context consumer in the component where we need the context information
Accessing context information and using it in render method
Let us create an application to better understand context and its usage. Let us create a global context for maintaining theme information in the application root component and use it in our child component.
First of all, create and start an application using below command,
create-react-app myapp cd myapp npm start
Next, create a component, HelloWorld under components folder (src/components/HelloWorld.js)
import React from "react"; import ThemeContext from "../ThemeContext"; class HelloWorld extends React.Component { render() { return <div>Hello World</div> } } export default HelloWorld
Next, create with a new context (src/ThemeContext.js) for maintaining theme information.
import React from 'react' const ThemeContext = React.createContext({ color: 'black', backgroundColor: 'white' }) export default ThemeContext
Here,
A new context is created using React.createContext.
Context is modeled as an object having style information.
Set initial value for color and background of the text.
Next, update the root component, App.js by including HelloWorld component and the theme provider with initial value for the theme context.
import './App.css'; import HelloWorld from './components/HelloWorld'; import ThemeContext from './ThemeContext' function App() { return ( <ThemeContext.Provider value={{ color: 'white', backgroundColor: 'green' }}> <HelloWorld /> </ThemeContext.Provider> ); } export default App;
Here, the ThemeContext.Provider is used, which is a non-visual component to set the value of the theme context to be used in all its children component.
Next, include a context consumer in HelloWorld component and style the hello world message using theme information in HelloWorld component.
import React from "react"; import ThemeContext from "../ThemeContext"; class HelloWorld extends React.Component { render() { return ( <ThemeContext.Consumer> {({color, backgroundColor} ) => (<div style={{ color: color, backgroundColor: backgroundColor }}> Hello World </div>) } </ThemeContext.Consumer> ) } } export default HelloWorld
Here,
Used ThemeContext.Consumer, which is a non-visual component providing access to the current theme context details
Used a function expression to get the current context information inside ThemeContext.Consumer
Used object deconstruction syntax to get the theme information and set the value in color and backgroundColor variable.
Used the theme information to style the component using style props.
Finally, open the browser and check the output of the application
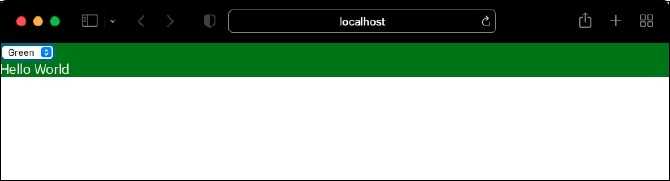
Summary
Context reduces the complexity of maintaining global data in a react application. It provides a clean concept of provider and consumer and simplifies the implementation of context.