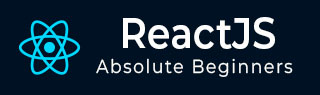
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - Forwarding Refs
Ref is an escape hatch to manipulate the DOM directly without the effect of state changes updating the component. Ref can be applied to DOM element but to apply the Ref to a React component and get the DOM element deep inside the component, Forwarding Ref is the choice. Forwarding Ref lets a component to receive a ref from the top level component and pass it further down into the next level component for the purpose of getting the DOM element.
Let us learn how to use Forwarding ref in this chapter.
Signature of the forwardRef method
The signature of forwardRef is as follows −
const new-component = React.forwardRef(fn)
Where the signature of the fn is as follows −
(props, ref) => { // renders a react component by attaching the ref and returns it }
A simple example of using forwardRef is as follows −
const MyInput = React.forwardRef((props, ref) => ( <input type="text" ref={ref} value={props.value} /> )); const myRef = React.createRef(); <MyInput ref={myRef} value="Hi" />
Here,
MyInput gets the ref from the top level and pass it to the underlying input element.
myRef is is assigned to MyInput component.
MyInput component passes myRef to underlying input element.
Finally, myRef points to the input element.
Applying forwardRef in a component
Let us learn the concept of forwardRef by developing an application.
First of all, create a new react application and start it using below command.
create-react-app myapp cd myapp npm start
Next, open App.css (src/App.css) and remove all the CSS classes. Then, create a simple component, SimpleForwardRef (src/Components/SimpleForwardRef.js) as shown below −
import React from "react"; const SimpleForwardRef = React.forwardRef((props, ref) => ( <input type="text" ref={ref} value={props.value} /> )); export default SimpleForwardRef
Here we have,
Used forwardRef to pass the ref to input element.
input element used ref props to set the ref value.
Next, open App component (src/App.js) and update the content with SimpleForwardRef component as shown below −
import './App.css' import React, { useEffect } from 'react'; import SimpleForwardRef from './Components/SimpleForwardRef' function App() { const myRef = React.createRef(); useEffect(() => { setTimeout(() => { myRef.current.value = "Hello" }, 5000) }) return ( <div className="container"> <div style={{ padding: "10px" }}> <div> <SimpleForwardRef ref={myRef} value="Hi" /> </div> </div> </div> ); } export default App;
Here,
myRef is created using createRef method and passed it into SimpleForwardRef component.
myRef represent the input element rendered by SimpleForwardRef component.
useEffect will access the input element through myRef and try to change the value of input from hi to Hello.
Finally, open the application in the browser. The value of the input will be get changed to Hello after 5 seconds as shown below −
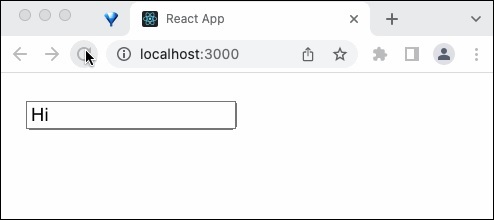
Summary
Forward ref enhances the ref concept to be used anywhere in the react application. Any DOM element, which may be any level deep inside the component hierarchy can be accessed and manipulated using forwarding ref concept.