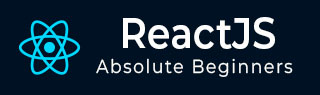
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - isElementOfType()
React JS is a tool for making computer applications. It breaks down the program into different parts called components. Each component has its own timeline. React gives us some special tools that we can use at specific points in the timeline of a component.
So we will learn about the "isElementOfType()" tool. This tool checks if something is a React component. If it is, it says "true."
Syntax
isElementOfType( element, componentClass )
Parameters
element − It is a React element to which we have to check that it is a React element or not.
componentClass − This is the kind of React component we are checking for. It is like a description of what the component should be like.
Return Value
It returns a boolean value. It returns true if the element is a React element with a React componentClass type. Otherwise it returns false.
Examples
Example − Simple React App
Now let us create a React app using the isElementOfType function. We have a React component named "App" with a button. The "checkElement" method is called when we click the button. This function verifies that an element with the ID 'myElement' is a React component of type "MyComponent" and the console logs the message.
import React from 'react'; import { isElementOfType } from 'react-dom/test-utils'; // Define App Component const App = () => { // Function to show isElementOfType() function checkElement() { // Get an HTML element by its ID var element = document.getElementById('myElement'); // Check if the element is a React component var isReactComponent = isElementOfType(element, MyComponent); // Console the result console.log("Is the element a React component?", isReactComponent); } // Returning our JSX code return ( <div> <h1>Simple React Example</h1> <button onClick={checkElement}>Check Element</button> </div> ); } // Define a custom React component function MyComponent() { return <p>This is a custom React component.</p>; } export default App;
Output
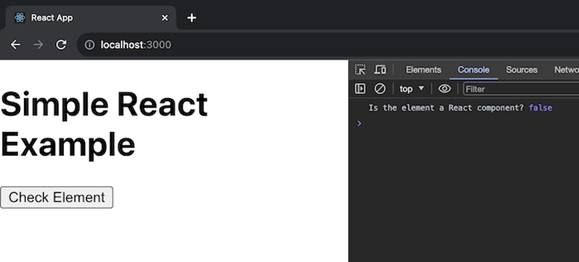
Example − Check Header element
In this example, we will use the isElementOfType function to create another React app. We have a "App" React component with a button. When we click the button, the "checkElement" method is called. This function verifies that an element with the ID 'headerElement' is a React component of type "Header" and logs the message to the console.
import React from 'react'; import { isElementOfType } from 'react-dom/test-utils'; // Define a React component function Header() { return <h2>This is a header component.</h2>; } // Define App Component const App = () => { // Function to show isElementOfType() function checkElement() { var element = document.getElementById('headerElement'); var isHeaderComponent = isElementOfType(element, Header); // Console the result console.log("Is the element a Header component?", isHeaderComponent); } // Returning our JSX code return ( <div> <h1>React Component Example</h1> <button onClick={checkElement}>Check Header Element</button> <div id="headerElement"> <Header /> </div> </div> ); } export default App;
Output
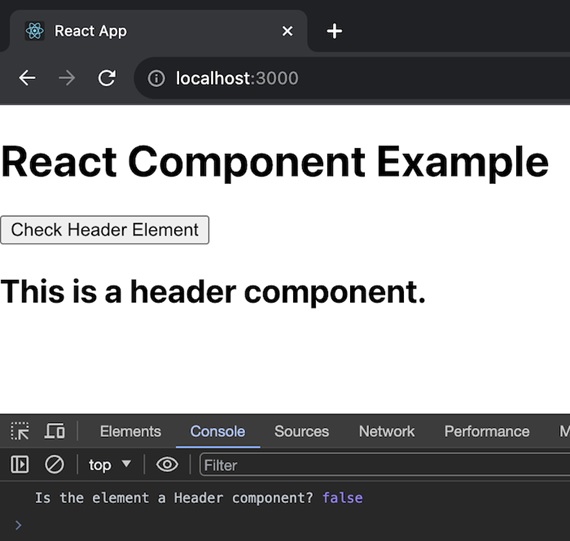
Example − Check Paragraph Element
In this example, we have developed a basic Paragraph component, and the checkElement function checks that the element with the id "paragraphElement" is of the type Paragraph and returns a boolean value. So the code for this app is mentioned below −
import React from 'react'; import { isElementOfType } from 'react-dom/test-utils'; // Define a React component function Paragraph() { return <p>This is a paragraph component.</p>; } // Define App Component const App = () => { // Function to show isElementOfType() function checkElement() { var element = document.getElementById('paragraphElement'); var isParagraphComponent = isElementOfType(element, Paragraph); // Console the result console.log("Is the element a Paragraph component?", isParagraphComponent); } // Returning our JSX code return ( <div> <h1>React Component Example</h1> <button onClick={checkElement}>Check Paragraph Element</button> <div id="paragraphElement"> <Paragraph /> </div> </div> ); } export default App;
Output
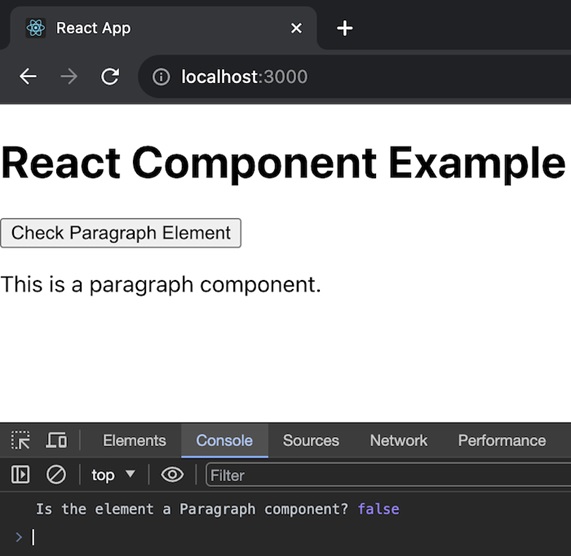
Summary
The "isElementOfType()" method determines that a given element is a React component of a particular type. In a simple example, a React component named "App" with a button is defined. When we click the button, it analyzes that an HTML element with a specific ID is a React component of a particular type and records its result.
To Continue Learning Please Login