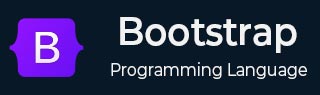
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Tooltip Plug-in
Tooltips are useful when you need to describe a link. The plugin was inspired by jQuery.tipsy plugin written by Jason Frame. Tooltips have since been updated to work without images, animate with a CSS animation, and data-attributes for local title storage.
If you want to include this plugin functionality individually, then you will need tooltip.js. Else, as mentioned in the chapter Bootstrap Plugins Overview, you can include bootstrap.js or the minified bootstrap.min.js.
Usage
The tooltip plugin generates content and markup on demand, and by default places tooltips after their trigger element. You can add tooltips in the following two ways −
Via data attributes − To add a tooltip, add data-toggle = "tooltip" to an anchor tag. The title of the anchor will be the text of a tooltip. By default, tooltip is set to top by the plugin.
<a href = "#" data-toggle = "tooltip" title = "Example tooltip">Hover over me</a>
Via JavaScript − Trigger the tooltip via JavaScript −
$('#identifier').tooltip(options)
Tooltip plugin is NOT only-css plugins like dropdown or other plugins discussed in previous chapters. To use this plugin you MUST activate it using jquery (read javascript). To enable all the tooltips on your page just use this script −
$(function () { $("[data-toggle = 'tooltip']").tooltip(); });
Example
The following example demonstrates the use of tooltip plugin via data attributes.
<h4>Tooltip examples for anchors</h4> This is a <a href = "#" class = "tooltip-test" data-toggle = "tooltip" title = "Tooltip on left"> Default Tooltip</a>. This is a <a href = "#" class = "tooltip-test" data-toggle = "tooltip" data-placement = "left" title = "Tooltip on left">Tooltip on Left</a>. This is a <a href = "#" data-toggle = "tooltip" data-placement = "top" title = "Tooltip on top">Tooltip on Top</a>. This is a <a href = "#" data-toggle = "tooltip" data-placement = "bottom" title = "Tooltip on bottom">Tooltip on Bottom</a>. This is a <a href = "#" data-toggle = "tooltip" data-placement = "right" title = "Tooltip on right">Tooltip on Right</a> <br> <h4>Tooltip examples for buttons</h4> <button type = "button" class = "btn btn-default" data-toggle = "tooltip" title = "Tooltip on left"> Default Tooltip </button> <button type = "button" class = "btn btn-default" data-toggle = "tooltip" data-placement = "left" title = "Tooltip on left"> Tooltip on left </button> <button type = "button" class = "btn btn-default" data-toggle = "tooltip" data-placement = "top" title = "Tooltip on top"> Tooltip on top </button> <button type = "button" class = "btn btn-default" data-toggle = "tooltip" data-placement = "bottom" title = "Tooltip on bottom"> Tooltip on bottom </button> <button type = " button" class = " btn btn-default" data-toggle = "tooltip" data-placement = "right" title = "Tooltip on right"> Tooltip on right </button> <script> $(function () { $("[data-toggle = 'tooltip']").tooltip(); }); </script>
Options
There are certain options which can be added via the Bootstrap Data API or invoked via JavaScript. Following table lists the options −
Option Name | Type/Default Value | Data attribute name | Description |
---|---|---|---|
animation | boolean Default: true | data-animation | Applies a CSS fade transition to the tooltip. |
html | boolean Default: false | data-html | Inserts HTML into the tooltip. If false, jQuery’s text method will be used to insert content into the dom. Use text if you’re worried about XSS attacks. |
placement | string|function Default: top | data-placement | Specifies how to position the tooltip (i.e., top|bottom|left|right|auto). When auto is specified, it will dynamically reorient the tooltip. For example, if placement is "auto left", the tooltip will display to the left when possible, otherwise it will display right. |
selector | string Default: false | data-selector | If a selector is provided, tooltip objects will be delegated to the specified targets. |
title | string | function Default: " | data-title | The title option is the default title value if the title attribute isn’t present. |
trigger | string Default: 'hover focus' | data-trigger | Defines how the tooltip is triggered: click| hover | focus | manual. You may pass multiple triggers; separate them with a space. |
content | string | function Default: '' | data-content | Default content value if data-content attribute isn't present |
delay | number | object Default: 0 | data-delay | Delays showing and hiding the tooltip in ms — does not apply to manual trigger type. If a number is supplied, delay is applied to both hide/show. Object structure is − delay: { show: 500, hide: 100 } |
container | string | false Default: false | data-container | Appends the tooltip to a specific element. Example: container: 'body' |
Methods
The following are some useful methods for tooltips −
Method | Description | Example |
---|---|---|
Options − .tooltip(options) |
Attaches a tooltip handler to an element collection. |
$().tooltip(options) |
Toggle − .tooltip('toggle') |
Toggles an element's tooltip. |
$('#element').tooltip('toggle') |
Show − .tooltip('show') |
Reveals an element's tooltip. |
$('#element').tooltip('show') |
Hide − .tooltip('hide') |
Hides an element's tooltip. |
$('#element').tooltip('hide') |
Destroy − .tooltip('destroy') |
Hides and destroys an element's tooltip. |
$('#element').tooltip('destroy') |
Example
The following example demonstrates the use of tooltip plugin via data attributes.
<div style = "padding: 100px 100px 10px;"> This is a <a href = "#" class = "tooltip-show" data-toggle = "tooltip" title = "show">Tooltip on method show</a>. This is a <a href = "#" class = "tooltip-hide" data-toggle = "tooltip" data-placement = "left" title = "hide">Tooltip on method hide</a>. This is a <a href = "#" class = "tooltip-destroy" data-toggle = "tooltip" data-placement = "top" title = "destroy">Tooltip on method destroy</a>. This is a <a href = "#" class = "tooltip-toggle" data-toggle = "tooltip" data-placement = "bottom" title = "toggle">Tooltip on method toggle</a>. <br><br><br><br><br><br> <p class = "tooltip-options" > This is a <a href = "#" data-toggle = "tooltip" title = "<h2>'am Header2 </h2>">Tooltip on method options</a>. </p> <script> $(function () { $('.tooltip-show').tooltip('show');}); $(function () { $('.tooltip-hide').tooltip('hide');}); $(function () { $('.tooltip-destroy').tooltip('destroy');}); $(function () { $('.tooltip-toggle').tooltip('toggle');}); $(function () { $(".tooltip-options a").tooltip({html : true });}); </script> </div>
Events
Following table lists the events to work with tooltip plugin. This event may be used to hook into the function.
Event | Description | Example |
---|---|---|
show.bs.tooltip | This event fires immediately when the show instance method is called. |
$('#myTooltip').on('show.bs.tooltip', function () { // do something }) |
shown.bs.tooltip | This event is fired when the tooltip has been made visible to the user (will wait for CSS transitions to complete). |
$('#myTooltip').on('shown.bs.tooltip', function () { // do something }) |
hide.bs.tooltip | This event is fired immediately when the hide instance method has been called. |
$('#myTooltip').on('hide.bs.tooltip', function () { // do something }) |
hidden.bs.tooltip | This event is fired when the tooltip has finished being hidden from the user (will wait for CSS transitions to complete). |
$('#myTooltip').on('hidden.bs.tooltip', function () { // do something }) |
Example
The following example demonstrates the use of tooltip plugin via data attributes.
<h4>Tooltip examples for anchors</h4> This is a <a href = "#" class = "tooltip-show" data-toggle = "tooltip" title = "Default Tooltip">Default Tooltip</a>. <script> $(function () { $('.tooltip-show').tooltip('show');}); $(function () { $('.tooltip-show').on('show.bs.tooltip', function () { alert("Alert message on show"); })}); </script>