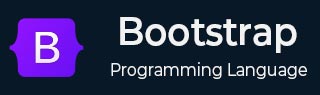
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Button Group
This chapter will discuss about Bootstrap button groups. Bootstrap button group puts multiple buttons together on a single line, horizontally or vertically.
Basic example
You can create a group of buttons using .btn in .btn-group class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-group" role="group" aria-label="Basic Example"> <button type="button" class="btn btn-info">Register</button> <button type="button" class="btn btn-danger">Submit</button> <button type="button" class="btn btn-warning">Cancel</button> </div> </body> </html>
To ensure accessibility for assistive technologies like screen readers, it is important to use the appropriate role attribute and provide explicit labels for button groups. You can use role="group" for button groups or role="toolbar" for button toolbars. To label them, you can use aria-label or aria-labelledby.
As an alternative to the .nav navigation components, these classes can also be applied to groups of links.
You can highlight a link by using .active class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-group my-2"> <a href="#" class="btn btn-success active" aria-current="page">Registration Link</a> <a href="#" class="btn btn-success">Submit</a> <a href="#" class="btn btn-success">Cancel</a> </div> </body> </html>
Mixed styles
In the case of button groups mixed styles, it is possible to form a button group where each button has a distinct background color.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-group mt-2" role="group" aria-label="Mixed styles"> <button type="button" class="btn btn-info">Register</button> <button type="button" class="btn btn-success">Cancel</button> <button type="button" class="btn btn-warning">Submit</button> </div> </body> </html>
Outlined styles
Use button styles such as btn-outline-primary, btn-outline-dark, etc to show the colors only for the border of the buttons element in the group.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-group my-2" role="group" aria-label="Basic outlined"> <button type="button" class="btn btn-outline-primary">Register</button> <button type="button" class="btn btn-outline-dark">Submit</button> <button type="button" class="btn btn-outline-warning">Cancel</button> </div> </body> </html>
Checkbox and radio button groups
Using button like-checkbox toggle buttons, we can create seamless looking button group. To acheive this use .btn-check class and type="checkbox".
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-group my-2" role="group" aria-label="checkbox toggle button group"> <input type="checkbox" class="btn-check" id="btnCheckbox1" autocomplete="off"> <label class="btn btn-outline-primary" for="btnCheckbox1">Register</label> <input type="checkbox" class="btn-check" id="btnCheckbox2" autocomplete="off"> <label class="btn btn-outline-warning" for="btnCheckbox2">Submit</label> <input type="checkbox" class="btn-check" id="btnCheckbox3" autocomplete="off"> <label class="btn btn-outline-info" for="btnCheckbox3">Cancel</label> </div> </body> </html>
Using button like-radio toggle button, we can create a seamless looking button group. To aceive this use .btn-check and type="radio".
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-group" role="group" aria-label="radio toggle button group"> <input type="radio" class="btn-check" name="radioButton" id="buttonRadio1" autocomplete="off" checked> <label class="btn btn-outline-primary" for="buttonRadio1">Register</label> <input type="radio" class="btn-check" name="radioButton" id="buttonRadio2" autocomplete="off"> <label class="btn btn-outline-warning" for="buttonRadio2">Submit</label> <input type="radio" class="btn-check" name="radioButton" id="buttonRadio3" autocomplete="off"> <label class="btn btn-outline-success" for="buttonRadio3">Cancel</label> </div> </body> </html>
Button toolbar
Button toolbars can be created by combining sets of button groups. Use utility classes to add a space between groups, buttons, etc.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-toolbar mt-2" role="toolbar" aria-label="Toolbar button groups"> <div class="btn-group me-2" role="group" aria-label="toolbarButtonGroup1"> <button type="button" class="btn btn-primary">Primary</button> <button type="button" class="btn btn-info">Info</button> <button type="button" class="btn btn-secondary">Secondary</button> </div> <div class="btn-group me-2" role="group" aria-label="toolbarButtonGroup2"> <button type="button" class="btn btn-success">Success</button> <button type="button" class="btn btn-dark">Dark</button> </div> <div class="btn-group" role="group" aria-label="toolbarButtonGroup3"> <button type="button" class="btn btn-warning">Warning</button> </div> </div> </body> </html>
Combine input groups and button groups in toolbars. Space things appropriately using utilities.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-toolbar mb-3" role="toolbar" aria-label="toolbarButtonGroup1"> <div class="btn-group me-2" role="group" aria-label="toolbarButtonGroup2"> <button type="button" class="btn btn-outline-primary">Primary</button> <button type="button" class="btn btn-outline-info">Info</button> <button type="button" class="btn btn-outline-secondary">secondary</button> </div> <div class="input-group"> <div class="input-group-text" id="inputbtnGroup1">@</div> <input type="text" class="form-control" placeholder="Username" aria-label="toolbarButtonGroup3" aria-describedby="inputButtonGroup1"> </div> </div> <div class="btn-toolbar justify-content-between" role="toolbar" aria-label="toolbarButtonGroup4"> <div class="btn-group" role="group" aria-label="toolbarButtonGroup1"> <button type="button" class="btn btn-outline-warning">Warning</button> <button type="button" class="btn btn-outline-success">Success</button> </div> <div class="input-group"> <input type="text" class="form-control" placeholder="tutorialspoint" aria-label="toolbarButtonGroup5" aria-describedby="inputButtonGroup2"> <div class="input-group-text" id="inputbtnGroup2">@example.com</div> </div> </div> </body> </html>
Sizing
When nesting several groups, use .btn-group-* on each .btn-group rather than button sizing classes for each button.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h6 class="mt-4">Default size button group</h6> <div class="btn-group" role="group" aria-label="Default size button group"> <button type="button" class="btn btn-outline-dark">Dark</button> <button type="button" class="btn btn-outline-warning">Warning</button> </div> <h6 class="mt-4">Small size button group</h6> <div class="btn-group btn-group-sm" role="group" aria-label="Small size button group"> <button type="button" class="btn btn-outline-secondary">Secondary</button> <button type="button" class="btn btn-outline-success">Success</button> </div> <h6 class="mt-4">Large size button group</h6> <div class="btn-group btn-group-lg" role="group" aria-label="Large size button group"> <button type="button" class="btn btn-outline-primary">Primary</button> <button type="button" class="btn btn-outline-info">Info</button> </div> </body> </html>
Nesting
To get the dropdown menu mixed with button series, use .btn-group within another .btn-group.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-group mt-2" role="group" aria-label="Nested dropdown"> <button type="button" class="btn btn-primary">Submit</button> <button type="button" class="btn btn-warning">Cancel</button> <div class="btn-group" role="group"> <button type="button" class="btn btn-info dropdown-toggle" data-bs-toggle="dropdown" aria-expanded="false"> Languages </button> <ul class="dropdown-menu"> <li><a class="dropdown-item" href="#">English</a></li> <li><a class="dropdown-item" href="#">Hindi</a></li> </ul> </div> </div> </body> </html>
Vertical variation
To create vertically stacked button group, use .btn-group-vertical class. Bootstrap doesn't support split button dropdowns in this case.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-group-vertical mt-2" role="group" aria-label="Button group with verical variation"> <button type="button" class="btn btn-primary">Primary</button> <button type="button" class="btn btn-warning">Warning</button> <button type="button" class="btn btn-info">Info</button> </div> </body> </html>
Create the vertical button group with the dropdown menu.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-group-vertical mt-2" role="group" aria-label="Vertical button group"> <button type="button" class="btn btn-primary">Primary</button> <button type="button" class="btn btn-info">Secondary</button> <div class="btn-group" role="group"> <button type="button" class="btn btn-warning dropdown-toggle" data-bs-toggle="dropdown" aria-expanded="false"> Languages </button> <ul class="dropdown-menu"> <li><a class="dropdown-item" href="#">English</a></li> <li><a class="dropdown-item" href="#">French</a></li> </ul> </div> <button type="button" class="btn btn-success">Success</button> </div> </div> </body> </html>
Create vertical button group with radio buttons.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Button Groups</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="btn-group-vertical mt-2" role="group" aria-label="Vertical button group with radio"> <input type="radio" class="btn-check" name="vbtn-radio" id="verticalRadioButton1" autocomplete="off" > <label class="btn btn-outline-warning" for="verticalRadioButton1">Unchecked Radio Button</label> <input type="radio" class="btn-check" name="vbtn-radio" id="verticalRadioButton2" autocomplete="off" checked> <label class="btn btn-outline-warning" for="verticalRadioButton2">checked Radio Button</label> <input type="radio" class="btn-check" name="vbtn-radio" id="verticalRadioButton3" autocomplete="off"> <label class="btn btn-outline-warning" for="verticalRadioButton3">Unchecked Radio Button</label> </div> </body> </html>