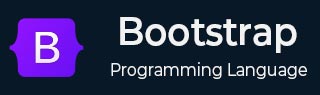
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Pagination
This chapter will discuss about the pagination. Pagination is a component that allows you to display a list of items across multiple pages. It provides a simple way to navigate through a large set of data by splitting it into smaller, more manageable chunks. In order to identify it as a navigation section to screen readers and other assistive technologies, use the wrapping <nav> element.
There could be more than one navigation section on pages, so it is advised to provide a descriptive aria-label for the <nav> element, so that the right purpose is served.
Like, if the pagination component is used by an online store selling shoes for navigating between a set of products (shoes) available, an appropriate label could be aria-label="Search shoes".
Simple Pagination
In order to create a basic pagination, add the .pagination class to an <ul> element. To each <li> element add the .page-item and a .page-link class to the link inside it.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Pagination</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="container mt-4"> <nav aria-label="Navigation page"> <ul class="pagination"> <li class="page-item"><a class="page-link" href="#">Previous</a></li> <li class="page-item"><a class="page-link" href="#">1</a></li> <li class="page-item"><a class="page-link" href="#">2</a></li> <li class="page-item"><a class="page-link" href="#">3</a></li> <li class="page-item"><a class="page-link" href="#">Next</a></li> </ul> </nav> </div> </body> </html>
Working with icons
Let's look at how to insert a symbol or icon in place of some pagination link text. For screen readers to operate effectively, users must use correct aria attributes.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Pagination</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="container mt-4"> <nav aria-label="Navigation page"> <ul class="pagination"> <li class="page-item"> <a class="page-link" href="#" aria-label="Previous"> <span aria-hidden="true">«</span> </a> </li> <li class="page-item"><a class="page-link" href="#">1</a></li> <li class="page-item"><a class="page-link" href="#">2</a></li> <li class="page-item"><a class="page-link" href="#">3</a></li> <li class="page-item"> <a class="page-link" href="#" aria-label="Next"> <span aria-hidden="true">»</span> </a> </li> </ul> </nav> </div> </body> </html>
Pagination state - disabled and active
Pagination links can be modified based on the circumstances. For links that don't appear to be clickable, use .disabled class, and use .active class to show the current page that is now being viewed.
-
Users can use .disabled class for un-clickable links
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Pagination</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="container mt-4"> <nav aria-label="Disabled link"> <ul class="pagination"> <li class="page-item disabled"> <a class="page-link">Previous</a> </li> <li class="page-item"><a class="page-link" href="#">1</a></li> <li class="page-item active" aria-current="page"> <a class="page-link" href="#">2</a> </li> <li class="page-item"><a class="page-link" href="#">3</a></li> <li class="page-item"> <a class="page-link" href="#">Next</a> </li> </ul> </nav> </div> </body> </html>
-
Users can use the .active class in order to "highlight" the current page
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Pagination</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="container mt-4"> <nav aria-label="Active link"> <ul class="pagination"> <li class="page-item"><a class="page-link" href="#">Previous</a></li> <li class="page-item"><a class="page-link" href="#">1</a></li> <li class="page-item"><a class="page-link" href="#">2</a></li> <li class="page-item active"><a class="page-link" href="#">3</a></li> <li class="page-item"><a class="page-link" href="#">Next</a></li> </ul> </nav> </div> </body> </html>
Sizing
Users can select between stylish larger and smaller pagination.
Following are the classes to be used for different sizes:
- .pagination-lg for larger size
- .pagination-sm for smaller size.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Pagination</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="container mt-4"> <nav aria-label="Sizing large"> <ul class="pagination pagination-lg"> <li class="page-item active" aria-current="page"> <span class="page-link">1</span> </li> <li class="page-item"><a class="page-link" href="#">2</a></li> <li class="page-item"><a class="page-link" href="#">3</a></li> <li class="page-item"><a class="page-link" href="#">4</a></li> </ul> </nav> <nav aria-label="Sizing small"> <ul class="pagination pagination-sm"> <li class="page-item active" aria-current="page"> <span class="page-link">1</span> </li> <li class="page-item"><a class="page-link" href="#">2</a></li> <li class="page-item"><a class="page-link" href="#">3</a></li> <li class="page-item"><a class="page-link" href="#">4</a></li> </ul> </nav> </div> </body> </html>
Alignment
The alignment options can be used to control the positioning of pagination components on a web page.
Horizontally left alignment is the default for pagination. As demonstrated below, add the class .justify-content-center to the .pagination base class to centre it on the page.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Pagination</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="container mt-4"> <nav aria-label="Pagination center alignment"> <ul class="pagination justify-content-center"> <li class="page-item"><a href="#" class="page-link">Previous</a></li> <li class="page-item"><a href="#" class="page-link">1</a></li> <li class="page-item"><a href="#" class="page-link">2</a></li> <li class="page-item"><a href="#" class="page-link">3</a></li> <li class="page-item"><a href="#" class="page-link">4</a></li> <li class="page-item"><a href="#" class="page-link">Next</a></li> </ul> </nav> </div> </body> </html>
Alignment using a flex utility
Refer the various classes provided under flex utilities to set the alignment of the pagination component. Alignment can be set as left, center, right, and many more using flexbox utilities.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Pagination</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="container mt-4"> <nav aria-label="Pagination right aligned using flex"> <ul class="pagination justify-content-end d-flex"> <li class="page-item"><a href="#" class="page-link">Previous</a></li> <li class="page-item"><a href="#" class="page-link">1</a></li> <li class="page-item"><a href="#" class="page-link">2</a></li> <li class="page-item"><a href="#" class="page-link">3</a></li> <li class="page-item"><a href="#" class="page-link">4</a></li> <li class="page-item"><a href="#" class="page-link">Next</a></li> </ul> </nav> </div> </body> </html>