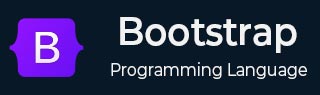
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Position
This chapter discusses about configuration of position of an element.
Bootstrap provides a set of helper classes to set the position of an element on a page. Some of the classes provided by Bootstrap are as follows:.
.fixed-top
.fixed-bottom
.sticky-top
.sticky-bottom
Let us see examples for each set of classes.
Fixed top
The class .fixed-top sets the position of an element at the top of the viewport, from edge to edge.
This is useful for creating navigation bars or headers that remain visible at the top of the screen even as the user scrolls down.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Position - Fixed top</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body style="height:1500px"> <nav class="navbar navbar-expand-lg text-bg-success navbar-dark fixed-top"> <div class="container-fluid"> <a class="navbar-brand" href="#">Use of .fixed-top class</a> </div> </nav> <div class="container-fluid" style="margin-top:80px"> <h4>Position fixed at top</h4> <p>The class .fixed-top of Bootstrap sets the position of the element at top of the screen.</p> <h1>Scroll down the page to see the position</h1> </div> </body> </html>
Fixed bottom
The class .fixed-bottom sets the position of an element at the bottom of the viewport, from edge to edge.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Position - Fixed bottom</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body style="height:1500px"> <nav class="navbar navbar-expand-lg text-bg-primary navbar-dark fixed-bottom"> <div class="container-fluid"> <a class="navbar-brand" href="#">Use of .fixed-bottom class</a> </div> </nav> <div class="container-fluid" style="margin-top:80px"> <h4>Position fixed at bottom</h4> <p>The class .fixed-bottom of Bootstrap sets the position of the element at the bottom of the page.</p> <h1>Scroll down the page to see the position</h1> </div> </body> </html>
Sticky top
The class .sticky-top is used to create a sticky element that remains at the top of the screen, from edge to edge, but only after you scroll past it.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Position - Sticky top</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body style="height:1500px"> <div class="container-fluid mt-3"> <h3>Sticky Navbar</h3> <p>The class <b>.sticky-top</b> is used to create a sticky element that remains at the top of the screen, from edge to edge, but only after you scroll past it.</p> <p>Scroll down the page to see the effect.</p> </div> <nav class="navbar navbar-expand-lg bg-dark navbar-dark sticky-top"> <div class="container-fluid"> <a class="navbar-brand" href="#">Sticky top</a> </div> </nav> <div class="container-fluid"> <p>Example for .sticky-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> </div> </body> </html>
Responsive sticky top
Responsive sticky top classes are used in web design to create a navigation menu or header that sticks to the top of the screen as the user scrolls down the page. This ensures that the navigation menu is always visible and easily accessible, even as the user moves further down the page.
Some of the responsive sticky top classes provided by Bootstrap are as follows:
.sticky-sm-top sticks the position of element(s) to top on small sized viewports
.sticky-md-top sticks the position of element(s) to top on medium sized viewports
.sticky-lg-top sticks the position of element(s) to top on large sized viewports
.sticky-xl-top sticks the position of element(s) to top on extra large sized viewports
.sticky-xxl-top sticks the position of element(s) to top on extra extra large sized viewports
Let us see an example:
Note: Resize your browser to see the change.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Position - Responsive Sticky top</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body style="height:1500px"> <div class="container-fluid mt-3"> <h3>Sticky Navbar</h3> <p>The class <b>.sticky-lg-top</b> is a responsive class, used to create a sticky element that remains at the top of the screen, from edge to edge, but only after you scroll past it.</p> <p>Scroll down the page to see the effect.</p> </div> <nav class="navbar navbar-expand-lg bg-dark navbar-dark sticky-lg-top"> <div class="container-fluid"> <a class="navbar-brand" href="#">Responsive Sticky top</a> </div> </nav> <div class="container-fluid"> <p>Example for .sticky-lg-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-lg-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-lg-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-lg-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-lg-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-lg-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> <p>Example for .sticky-lg-top class of Bootstrap to set the position of an element, like a navbar, stick to the top of the screen.</p> </div> </body> </html>
Sticky bottom
The class .sticky-bottom is used to create a sticky element that remains at the bottom of the screen, from edge to edge, but only after you scroll past it.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Position - sticky bottom</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body style=height:1000px class="d-flex flex-column"> <!-- Navbar --> <nav class="navbar navbar-expand-lg navbar-light bg-light"> <div class="container"> <a class="navbar-brand" href="#">Sticky Bottom Example</a> </div> </nav> <!-- Content --> <div class="container-fluid flex-grow-1"> <h4>sticky bottom</h4> <p>Here is an example for responsive sticky bottom. Its a helper class in bootstrap 5.</p> <p>Here is an example for responsive sticky bottom. Its a helper class in bootstrap 5.</p> <p>Here is an example for responsive sticky bottom. Its a helper class in bootstrap 5.</p> <p>Here is an example for responsive sticky bottom. Its a helper class in bootstrap 5.</p> </div> <!-- Sticky Footer --> <footer class="footer text-bg-info py-3 sticky-bottom"> <div class="container"> <span class="display-6 text-dark">Sticky bottom</span> </div> </footer> </body> </html>
Responsive sticky bottom
Some of the responsive sticky bottom classes provided by Bootstrap are as follows:
.sticky-sm-bottom sticks the position of element(s) to bottom on small sized viewports
.sticky-md-bottom sticks the position of element(s) to bottom on medium sized viewports
.sticky-lg-bottom sticks the position of element(s) to bottom on large sized viewports
.sticky-xl-bottom sticks the position of element(s) to bottom on extra large sized viewports
.sticky-xxl-bottom sticks the position of element(s) to bottom on extra extra large sized viewports
Let us seen an example for a responsive sticky bottom class:
Note: Resize your browser to see the change.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Position - Responsive sticky bottom</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body style=height:1000px class="d-flex flex-column"> <!-- Navbar --> <nav class="navbar navbar-expand-lg navbar-light bg-light"> <div class="container"> <a class="navbar-brand" href="#">Responsive Sticky Bottom Example</a> </div> </nav> <!-- Content --> <div class="container-fluid flex-grow-1"> <h4>Responsive sticky bottom</h4> <p>Here is an example for responsive sticky bottom. Its a helper class in bootstrap 5.</p> <p>Here is an example for responsive sticky bottom. Its a helper class in bootstrap 5.</p> <p>Here is an example for responsive sticky bottom. Its a helper class in bootstrap 5.</p> <p>Here is an example for responsive sticky bottom. Its a helper class in bootstrap 5.</p> </div> <!-- Sticky Footer --> <footer class="footer text-bg-warning py-3 sticky-lg-bottom"> <div class="container"> <span class="display-6 text-dark">Sticky bottom</span> </div> </footer> </body> </html>