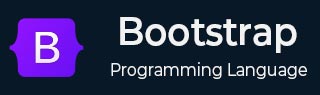
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Navs and Tabs
This chapter will discuss about Bootstrap navs and tabs. Create navigation menu using .nav class and tab navigation menu using .tab class.
Base nav
All types of Bootstrap's navigation components are built with base class .nav (component build with flexbox). The nav components can be used to add a list of links to browse to other pages within the website.
The items can be added within the .nav-item class.
To add links within the item class use .nav-link class.
There is no .active state in the .nav component. The class is used in the following examples, to show that this class does not have unique styling.
To convey active links to assistive technologies like screen readers use an aria-current attribute with value as a page for the current page or true if the current item is set.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav bg-warning mt-2"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoint</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
When item order is important, use <ul>s, <ol>, or create your own with <nav>. The nav links behave similar to the nav items because the .nav uses display: flex without extra markup.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <nav class="nav bg-light mt-2"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> <a class="nav-link" href="#">Home</a> <a class="nav-link disabled">Services</a> <a class="nav-link" href="#">About us</a> </nav> </body> </html>
Available styles
Use modifiers and utilities to change the styles of the .navs component as seen the following sections:
Horizontal alignment
Use flexbox utilities to modify the horizontal alignment of navigation. Navs are left-aligned by default. Navs can be easily centered or right-aligned.
For center-alignment, use the CSS class .justify-content-center.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav justify-content-center bg-warning mt-2"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
To achieve right-alignment, use the CSS class .justify-content-end.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav justify-content-end bg-light mt-2"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
Vertical
Use the .flex-column utility, that changes the flex item direction, in order to stack the navigation items. Use responsive versions to stack them on various viewports (e.g., .flex-sm-column).
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav flex-column bg-light mt-2"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
Even without using <ul>s, vertical navigation is possible.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <nav class="nav flex-column bg-light mt-2"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> <a class="nav-link" href="#">Home</a> <a class="nav-link disabled">Services</a> <a class="nav-link" href="#">About us</a> </nav> </body> </html>
Tabs
Add the .nav-tabs class to .nav to create a tab navigation menu. Use them to create tabbable regions using tab JavaScript plugin.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-tabs mt-2"> <li class="nav-item"> <a class="nav-link active bg-light" aria-current="page" href="#">Tutorialspoints</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
Pills
Create a navigation pills menu by replacing .nav-tab to .nav-pills within .nav class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-pills"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> </li> <li class="nav-item"> <a class="nav-link " href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
Underline
Create an underlined navigation menu, using .nav-underline class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-underline mt-2"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
Fill and justify
Use .nav-fill to evenly fill available space withon .nav-items. The horizontal space is completely occupied, even when the width of each nav item varies.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-pills nav-fill bg-light mt-2"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
With <nav>-based navigation, you can skip .nav-item and use .nav-link to style <a> elements.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <nav class="nav nav-pills nav-fill bg-light mt-2"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> <a class="nav-link" href="#">Home</a> <a class="nav-link disabled">Services</a> <a class="nav-link" href="#">About us</a> </nav> </body> </html>
Use .nav-justified for equal-width elements. This will fill the horizontal space with nav links, with each nav item having equal width, unlike the .nav-fill mentioned earlier.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-pills nav-justified bg-light mt-2"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
An example similar to the .nav-fill demonstration, but using a navigation based on the <nav> element.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <nav class="nav nav-pills nav-justified bg-light mt-2"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> <a class="nav-link" href="#">Home</a> <a class="nav-link disabled">Services</a> <a class="nav-link" href="#">About us</a> </nav> </body> </html>
Working with flex utilities
Use flexbox utilities, in case you require responsive nav variations.
These utilities provide more customization across all the various breakpoints. The navigation will be stacked at the lowest breakpoint and then switch to a horizontal layout that spans the entire width from the small breakpoint onwards.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <nav class="nav nav-pills flex-column flex-sm-row bg-warning mt-2"> <a class="flex-sm-fill text-sm-center nav-link active" aria-current="page" href="#">Tutorialspoints</a> <a class="flex-sm-fill text-sm-center nav-link" href="#">Home</a> <a class="flex-sm-fill text-sm-center nav-link disabled">Services</a> <a class="flex-sm-fill text-sm-center nav-link" href="#">About us</a> </nav> </body> </html>
Regarding accessibility
For navigation bars using navs, add role="navigation" to the parent container of the <ul> or wrap the entire navigation in a <nav> element. Don't add the role to the <ul> to avoid it being announced as a list by assistive technologies.
Navigation bars styled as .nav-tabs should not have the attributes role="tablist", role="tab" or role="tabpanel". This applies specifically to dynamic tabbed interfaces, as explained in the ARIA Authoring Practises Guide's tabs pattern. Check out the JavaScript behavior section for a sample of dynamic tabbed interfaces.
The aria-current attribute is unnecessary on dynamic tabbed interfaces as the JavaScript already manages the selected state by using aria-selected="true" on the active tab.
Using dropdowns
Dropdown menus can be added using HTML and the dropdowns JavaScript plugin.
Tabs with dropdowns
To create a tab with dropdown menu add .nav-tabs class to the <ul> element.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-tabs bg-warning mt-2"> <li class="nav-item"> <a class="nav-link active bg-light" aria-current="page" href="#">Tutorialspoints</a> </li> <li class="nav-item dropdown"> <a class="nav-link dropdown-toggle" data-bs-toggle="dropdown" href="#" role="button" aria-expanded="false">Home</a> <ul class="dropdown-menu"> <li><a class="dropdown-item" href="#">Sign in</a></li> <li><a class="dropdown-item" href="#">Sign out</a></li> </ul> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
Pills with dropdowns
Add .nav-pills class to <ul> to show dropdown menu as pills.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-pills bg-warning mt-2"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Tutorialspoints</a> </li> <li class="nav-item dropdown"> <a class="nav-link dropdown-toggle" data-bs-toggle="dropdown" href="#" role="button" aria-expanded="false">Home</a> <ul class="dropdown-menu"> <li><a class="dropdown-item" href="#">Sign in</a></li> <li><a class="dropdown-item" href="#">Sign out</a></li> </ul> </li> <li class="nav-item"> <a class="nav-link disabled">Services</a> </li> <li class="nav-item"> <a class="nav-link" href="#">About us</a> </li> </ul> </body> </html>
JavaScript behavior
Use navigational tabs and pills to generate tabbable panes of local content using the bootstrap.js file and tab JavaScript plugin.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-tabs bg-warning mt-2" id="myTab" role="list"> <li class="nav-item" role="presentation"> <button class="nav-link active" id="tutTab" data-bs-toggle="tab" data-bs-target="#tutorialspoints" type="button" role="tab" aria-controls="home-tab-pane" aria-selected="true">Tutorialspoints</button> </li> <li class="nav-item" role="presentation"> <button class="nav-link" id="homeTab" data-bs-toggle="tab" data-bs-target="#home" type="button" role="tab" aria-controls="profile-tab-pane" aria-selected="false">Home</button> </li> <li class="nav-item" role="presentation"> <button class="nav-link" id="servicesTab" data-bs-toggle="tab" data-bs-target="#services" type="button" role="tab" aria-controls="disabled-tab-pane" aria-selected="false" disabled>Services-disabled</button> </li> </ul> <div class="tab-content" id="myTabContent"> <div class="tab-pane fade show active bg-light" id="tutorialspoints" role="tabpanel" aria-labelledby="tutTab" tabindex="0">Tutorialspoints Tab Contents</div> <div class="tab-pane fade bg-light" id="home" role="tabpanel" aria-labelledby="homeTab" tabindex="0">Home Tab Contents</div> <div class="tab-pane fade bg-light" id="services" role="tabpanel" aria-labelledby="servicesTab" tabindex="0">Services Tab Content</div> </div> </body> </html>
This works with <ul>-based markup, or with any random "roll your own" markup.
To maintain the native role of <nav> as a navigation landmark, don't add role="tablist" directly to it. Instead, use an alternative element (such as a basic <div>) and enclose the <nav> within it.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <nav> <div class="nav nav-tabs bg-warning" id="nav-tab" role="tablist"> <button class="nav-link active" id="navTutTab" data-bs-toggle="tab" data-bs-target="#navTut" type="button" role="tab" aria-controls="nav-home" aria-selected="true">Tutorialspoints</button> <button class="nav-link" id="navHomeTab" data-bs-toggle="tab" data-bs-target="#navHome" type="button" role="tab" aria-controls="nav-profile" aria-selected="false">Home</button> <button class="nav-link" id="navServicesTab" data-bs-toggle="tab" data-bs-target="#navServices" type="button" role="tab" aria-controls="nav-disabled" aria-selected="false" disabled>Services-disabled</button> </div> </nav> <div class="tab-content bg-light" id="nav-tabContent"> <div class="tab-pane fade show active" id="navTut" role="tabpanel" aria-labelledby="navTutTab" tabindex="0">Tutorialspoints Tab Content</div> <div class="tab-pane fade" id="navHome" role="tabpanel" aria-labelledby="navHomeTab" tabindex="0">Home Tab Content</div> <div class="tab-pane fade" id="navServices" role="tabpanel" aria-labelledby="navServicesTab" tabindex="0">Services Tab Content</div> </div> </body> </html>
Pills can be used with the tabs plugin.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-pills mb-3 bg-warning mt-2" id="pilltablist" role="tablist"> <li class="nav-item" role="presentation"> <button class="nav-link active" id="pillsTutTab" data-bs-toggle="pill" data-bs-target="#pillsTut" type="button" role="tab" aria-controls="pillsTut" aria-selected="true">Tutorialspoints</button> </li> <li class="nav-item" role="presentation"> <button class="nav-link" id="pillsHomeTab" data-bs-toggle="pill" data-bs-target="#pillsHome" type="button" role="tab" aria-controls="pillsHome" aria-selected="false">Home</button> </li> <li class="nav-item" role="presentation"> <button class="nav-link" id="pillsServicesTab" data-bs-toggle="pill" data-bs-target="#pillsServices" type="button" role="tab" aria-controls="pillsServices" aria-selected="false" disabled>Services-disabled</button> </li> </ul> <div class="tab-content bg-light" id="pills-tabContent"> <div class="tab-pane fade show active" id="pillsTut" role="tabpanel" aria-labelledby="pillsTutTab" tabindex="0">Tutorialspoints Tab Contents</div> <div class="tab-pane fade" id="pillsHome" role="tabpanel" aria-labelledby="pillsHomeTab" tabindex="0">Home Tab Contents</div> <div class="tab-pane fade" id="pillsServices" role="tabpanel" aria-labelledby="pillsServicesTab" tabindex="0">Services Tab Contents</div> </div> </body> </html>
For vertical tabs, it is recommended to include aria-orientation="vertical" in the tab list container.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="d-flex align-items-start mt-2"> <div class="nav flex-column nav-pills me-3 bg-warning" id="vTabs" role="tablist" aria-orientation="vertical"> <button class="nav-link active" id="verticalTutTab" data-bs-toggle="pill" data-bs-target="#vTut" type="button" role="tab" aria-controls="vTut" aria-selected="true">Tutorialspoints</button> <button class="nav-link" id="verticalHomeTab" data-bs-toggle="pill" data-bs-target="#vHome" type="button" role="tab" aria-controls="vHome" aria-selected="false">Home</button> <button class="nav-link" id="verticalServicesTab" data-bs-toggle="pill" data-bs-target="#vServices" type="button" role="tab" aria-controls="vServices" aria-selected="false" disabled>Services-disabled</button> </div> <div class="tab-content bg-light" id="v-pills-tabContent"> <div class="tab-pane fade show active" id="vTut" role="tabpanel" aria-labelledby="verticalTutTab" tabindex="0">Tutorialspoints Tabs Contents</div> <div class="tab-pane fade" id="vHome" role="tabpanel" aria-labelledby="verticalHomeTab" tabindex="0">Home Tab Contents</div> <div class="tab-pane fade" id="vServices" role="tabpanel" aria-labelledby="verticalServicesTab" tabindex="0">Services Tab Contents</div> </div> </div> </body> </html>
Accessibility
Use role="tablist", role="tab", role="tabpanel" and aria-attributes to show structure, function, and current state to assistive technology users.
Use <button> elements for tabs as a best practice. These controls cause dynamic changes, instead of taking the user to another page.
According to the ARIA Authoring Practises, only active tab gets keyboard focus. JavaScript sets tabindex = "-1" on inactive tab controls when initialized.
To improve keyboard navigation, it's advisable to make tab panels focusable unless the first meaningful element inside them is already focusable. The JavaScript plugin doesn't handle this, so you need to add tabindex="0" to your tab panels to make them focusable.
The JavaScript plugin for tabs doesn't work well with tabbed interfaces that have dropdown menus due to usability and accessibility concerns. The dropdown menus hide the trigger element of the active tab, causing confusion for users.
Using data attributes
Activate tab or pill navigation by specifying data-bs-toggle="tab" or data-bs-toggle="pill" on an element with .nav-tabs or .nav-pills attributes.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-tabs bg-light" id="myTab" role="tablist"> <li class="nav-item" role="presentation"> <button class="nav-link active" id="tutTab" data-bs-toggle="tab" data-bs-target="#tutorialspoints" type="button" role="tab" aria-controls="tutorialspoints" aria-selected="true">Tutorialspoints</button> </li> <li class="nav-item" role="presentation"> <button class="nav-link" id="homeTab" data-bs-toggle="tab" data-bs-target="#home" type="button" role="tab" aria-controls="home" aria-selected="false">Home</button> </li> <li class="nav-item" role="presentation"> <button class="nav-link" id="servicesTab" data-bs-toggle="tab" data-bs-target="#services" type="button" role="tab" aria-controls="services" aria-selected="false">Services</button> </li> </ul> <div class="tab-content bg-warning"> <div class="tab-pane active" id="tutorialspoints" role="tabpanel" aria-labelledby="tutTab" tabindex="0"> <h4>Tutorialspoints</h4> <p>Tutorialspoints Content</p> </div> <div class="tab-pane" id="home" role="tabpanel" aria-labelledby="homeTab" tabindex="0"> <h4>Home</h4> <p>Home Contents</p></div> <div class="tab-pane" id="services" role="tabpanel" aria-labelledby="servicesTab" tabindex="0"> <h4>Services</h4> <p>Services Contents</p> </div> </div> </body> </html>
Via JavaScript
Use JavaScript to enable tabbable tabs (activate each tab individually).
Example
const triggerTabList = document.querySelectorAll('#tabButton') triggerTabList.forEach(triggerEl => { const tabTrigger = new bootstrap.Tab(triggerEl) triggerEl.addEventListener('click', event => { event.preventDefault() tabTrigger.show() }) })
Activate individual tabs in multiple ways.
Example
const triggerElement = document.querySelector('#myTab button[data-bs-target="#profile"]') bootstrap.Tab.getInstance(triggerElement).show() // Select tab by name const triggerFirstTabElement = document.querySelector('#myTab li:first-child button') bootstrap.Tab.getInstance(triggerFirstTabElement).show() // Select first tab
Fade effect
Use .fade to each .tab-pane to make the tabs fade in. The first tab pane must use .show to make the initial content visible.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Navs and Tabs</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <ul class="nav nav-pills bg-light" id="myTab" role="tablist"> <li class="nav-item" role="presentation"> <button class="nav-link active" id="tutTab" data-bs-toggle="tab" data-bs-target="#tut" type="button" role="tab" aria-controls="tutorialspoints" aria-selected="true">Tutorialspoints</button> </li> <li class="nav-item" role="presentation"> <button class="nav-link" id="homeTab" data-bs-toggle="tab" data-bs-target="#home" type="button" role="tab" aria-controls="home" aria-selected="false">Home</button> </li> <li class="nav-item" role="presentation"> <button class="nav-link" id="servicesTab" data-bs-toggle="tab" data-bs-target="#services" type="button" role="tab" aria-controls="services" aria-selected="false">Services</button> </li> </ul> <div class="tab-content bg-warning mt-2"> <div class="tab-pane fade show active p-2" id="tut" role="tabpanel" aria-labelledby="tutTab" tabindex="0"><h4>Tutorialspoint</h4>Fade effect is used to fade the visible element.</div> <div class="tab-pane fade p-2" id="home" role="tabpanel" aria-labelledby="homeTab" tabindex="0"><h4>Home</h4>Use .fade to each .tab-pane to make the tabs fade in.</div> <div class="tab-pane fade p-2" id="services" role="tabpanel" aria-labelledby="servicesTab" tabindex="0"><h4>Services</h4>The first tab pane must use .show to make the initial content visible.</div> </div> </body> </html>