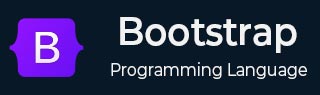
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Floating Labels
This chapter will discuss about Bootstrap floating labels. Floating labels refer to form labels that float over the input fields.
Basic example
To allow floating labels with Bootstrap's textual form fields, include a pair of <input class="form-control"> and <label> elements in .form-floating.
Each <input> must have a placeholder since the technique for CSS-only floating labels employs the :placeholder-shown pseudo-element. The <input> needs to be placed first to make use of a sibling selector like (~).
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-floating mb-3 mt-2"> <input type="text" class="form-control" id="floatingUsername" placeholder="tutorialspoint@example.com"> <label for="floatingUsername">Username</label> </div> <div class="form-floating"> <input type="password" class="form-control" id="floatingPassword" placeholder="Password"> <label for="floatingPassword">Password</label> </div> </body> </html>
When a value is already assigned, <label> elements will automatically align their position to float over the input field.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <form class="form-floating mt-2"> <input type="email" class="form-control" id="floatingInputValue" placeholder="tutorialspoint@example.com" value="tutorialspoint@example.com"> <label for="floatingInputValue">Username</label> </form> </body> </html>
By using form validation styles like is-invalid, you can provide visual feedback to users when they submit incorrect data.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <form class="form-floating"> <input type="text" class="form-control is-invalid" id="floatingInvalidInput" placeholder="Password" value="Password"> <label for="floatingInvalidInput">Invalid Password</label> </form> </body> </html>
Textareas
The height of <textarea> with the class .form-control is automatically set to have the same height as <input>.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-floating"> <textarea class="form-control" placeholder="Text Here" id="floatingTextarea"></textarea> <label for="floatingTextarea">Text Here</label> </div> </body> </html>
Don't use the rows attribute if you want to customize the height of your <textarea>. Instead, specify a height explicitly (either inline or using customized CSS). In the below example we have used inline styling.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-floating"> <textarea class="form-control" placeholder="Add a comment" id="floatingTextarea" style="height: 100px"></textarea> <label for="floatingTextarea">Add a comment</label> </div> </body> </html>
Selects
Floating labels are only accessible on .form-selects, in addition to .form-control.
The same concepts apply to them, except unlike <input>s, they always display the <label> in its floated state. Size-based and multiple selects are not supported.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-floating"> <select class="form-select" id="floatingSelect" aria-label="Floating label select menu"> <option selected>Language</option> <option value="1">English</option> <option value="2">Hindi</option> <option value="3">Marathi</option> </select> <label for="floatingSelect">Others</label> </div> </body> </html>
Disabled
Add the disabled boolean attribute on an input. This gives grayed-out appearance to an input, textarea, or select. It also removes pointer events, and prevents focusing.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-floating mb-3"> <input type="email" class="form-control" id="floatingInputDisabled" placeholder="name" disabled> <label for="floatingInputDisabled">Name</label> </div> <div class="form-floating mb-3"> <textarea class="form-control" placeholder="tutorialspoint@example.com" id="floatingEmailDisabled" disabled></textarea> <label for="floatingEmailDisabled">Email Id</label> </div> <div class="form-floating mb-3"> <textarea class="form-control" placeholder="Add a comment" id="floatingTextareaDisabled" style="height: 120px" disabled></textarea> <label for="floatingTextareaDisabled">Add a comment</label> </div> <div class="form-floating"> <select class="form-select" id="floatingSelectDisabled" aria-label="Floating label disabled select example" disabled> <option selected>Select Language</option> <option value="1">English</option> <option value="2">Hindi</option> <option value="3">Marathi</option> </select> <label for="floatingSelectDisabled">Others</label> </div> </body> </html>
Readonly plaintext
When switching from an editable <input> to a plaintext value without changing the page layout, .form-control-plaintext can be useful.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-floating mb-3 mt-2"> <input type="text" readonly class="form-control" id="floatingEmptyPlaintextInput" style="height: 80px" placeholder="Block the comment" value="Block the comment"> <label for="floatingEmptyPlaintextInput">Block the comment</label> </div> <div class="form-floating mb-3"> <input type="text" readonly class="form-control" id="floatingPlaintextInput" style="height: 80px" placeholder="Add a comment" value="Add a comment"> <label for="floatingPlaintextInput">Add a comment</label> </div> </body> </html>
Input groups
Similarly, floating labels support .input-group.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="input-group mb-3"> <div class="form-floating"> <input type="email" class="form-control" id="floatingInputGroup" placeholder="email"> <label for="floatingInputGroup">Email Id</label> </div> <span class="input-group-text">tutorialspoint@example.com</span> </div> </body> </html>
The -feedback (it is a validation class to provide valuable feedback to users before submitting the form.) should be positioned outside of the .form-floating but inside of the .input-group when using .input-group and .form-floating along with form validation.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="input-group has-validation"> <div class="form-floating is-invalid"> <input type="text" class="form-control is-invalid" id="floatingInputGroup" placeholder="Password" required> <label for="floatingInputGroup">Password</label> </div> <div class="invalid-feedback"> Wrong Password </div> </div> </body> </html>
Layout
When using a grid system, the implementation of floating labels layout becomes beneficial as it enables the placement of form elements within column classes.
There is no pre-defined classes in bootstrap, so we must utilize form classes and position them according to our requirement.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Floating Labels</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="row g-2"> <div class="col-md"> <div class="form-floating"> <input type="email" class="form-control" id="floatingGrid" placeholder="tutorialspoint@example.com" value="tutorialspoint@example.com"> <label for="floatingGrid">Email Id</label> </div> </div> <div class="col-md"> <div class="form-floating"> <select class="form-select" id="floatingSelectGrid"> <option selected>Language</option> <option value="1">English</option> <option value="2">Hindi</option> <option value="3">Marathi</option> </select> <label for="floatingSelectGrid">Others</label> </div> </div> </div> </html>