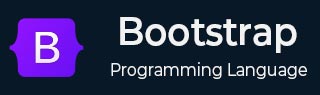
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Borders
This chapter discusses about the borders that can be applied to elements. Utilize border utilities to promptly apply styling to an element's border and border-radius, making it suitable for images, buttons, or any other element.
Borders can be either added or removed from an element. Bootstrap provides a range of border styles that can be chosen all at a time or one by one.
Add a border
Borders can be added to a custom element. Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Borders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> <style> span { display: inline-block; width: 100px; height: 100px; margin: 10px; background-color: #f1f1f1 } </style> </head> <body> <div class="container mt-3"> <h4>Borders</h4> <p>Use the border classes to add borders to an element:</p><br> <span class="border border-2"></span> <span class="border-top border-2"></span> <span class="border-end border-2"></span> <span class="border-bottom border-2"></span> <span class="border-start border-2"></span> </div> </body> </html>
Remove a border
Borders can be removed from a custom element. Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Borders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> <style> span { display: inline-block; width: 100px; height: 100px; margin: 10px; background-color: #f1f1f1; } </style> </head> <body> <div class="container mt-3"> <h4>Borders</h4> <p>Use the border classes to remove borders from an element:</p><br> <span class="border border-2"></span> <span class="border border-0 border-2"></span> <span class="border border-top-0 border-2"></span> <span class="border border-end-0 border-2"></span> <span class="border border-bottom-0 border-2"></span> <span class="border border-start-0 border-2"></span> </div> </body> </html>
Border colors
The border utilities, such as .border-* generated from the original $theme-colors Sass map, currently do not adapt to color modes. However, any .border-*-subtle utility will respond accordingly. This issue will be addressed in version 6.
The border color can be modified using the utilities built on theme colors. Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Borders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> <style> span { display: inline-block; width: 100px; height: 100px; margin: 10px; background-color: #faf8f8; } </style> </head> <body> <div class="container mt-3"> <h4>Borders color</h4><br> <p>Use the .border-* classes to add colors to the borders:</p><br> <span class="border border-success"></span> <span class="border border-success-subtle"></span> <span class="border border-danger"></span> <span class="border border-danger-subtle"></span> <span class="border border-light"></span> <span class="border border-light-subtle"></span> <span class="border border-secondary"></span> <span class="border border-secondary-subtle"></span> <span class="border border-primary"></span> <span class="border border-primary-subtle"></span> <span class="border border-black"></span> <span class="border border-white"></span> <span class="border border-info"></span> <span class="border border-info-subtle"></span> <span class="border border-warning"></span> <span class="border border-warning-subtle"></span> <span class="border border-dark"></span> <span class="border border-dark-subtle"></span> </div> </body> </html>
The default border of an element can also be modified using the .border-* classes.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Borders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container mt-3"> <h4>Border colors of elements</h4><br> <p>Use the .border-* classes to add colors to the borders of different elements:</p><br> <div class="h4 pb-4 mb-2 text-center text-success border border-success"> Border for success </div> <div class="p-2 bg-warning bg-opacity-25 border border-warning border-start-0"> Adding color to a warning text, with warning border. </div><br> <button class="btn btn-light text-dark border">Click</button> </div> </body> </html>
Border opacity
In order to change the opacity of the border, you need to override the --bs-border-opacity through the custom styles or inline styles.
Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Borders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container mt-3"> <h4>Border opacity of borders</h4><br> <p>Use the .border-* classes to change the opacity of the borders of different elements:</p><br> <div class="border border-primary p-2 mb-2">Primary border default</div> <div class="border border-danger p-2 mb-2 border-opacity-75">75% opacity of danger border</div> <div class="border border-warning p-2 mb-2 border-opacity-50">50% opacity of warning border</div> <div class="border border-success p-2 mb-2 border-opacity-25">25% opacity of success border</div> <div class="border border-secondary p-2 border-opacity-10">10% opacity of secondary border</div> </body> </html>
Border width
In order to change the width of the border of an element, use the classes such as .border-1, .border-2, .border-3, .border-4 and .border-5
Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Borders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> <style> span { display: inline-block; width: 100px; height: 100px; margin: 10px; background-color: #f9f6f6; } </style> </head> <body> <div class="container mt-3"> <h4>Borders - width</h4><br> <p>Set the border width with .border-* classes:</p><br> <span class="border border-5"></span> <span class="border border-4"></span> <span class="border border-3"></span> <span class="border border-2"></span> <span class="border border-1"></span> </div> </body> </html>
Border radius
In order to add rounded corners to any element, use the .rounded-* classes.
Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Borders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> <style> span { display: inline-block; width: 100px; height: 100px; margin: 10px; background-color: #440d0d; } </style> </head> <body> <div class="container mt-3"> <h4>Borders - Radius</h4> <p>Set the radius of the border with .rounded-* classes:</p><br> <span class="rounded"></span> <span class="rounded-top"></span> <span class="rounded-bottom"></span> <span class="rounded-start"></span> <span class="rounded-end"></span> <span class="rounded-circle"></span> <span class="rounded-pill" style="width:130px"></span> </div> </body> </html>
Borders size
Set the size of the rounded corners with the range of .rounded-* classes, starting from 0 to 5.
Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Borders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> <style> span { display: inline-block; width: 100px; height: 100px; margin: 10px; background-color: #440d0d; } </style> </head> <body> <div class="container mt-3"> <h4>Borders - Size</h4> <p>Set the size of the rounded corners with .rounded-* classes:</p><br> <span class="rounded-5"></span> <span class="rounded-4"></span> <span class="rounded-3"></span> <span class="rounded-2"></span> <span class="rounded-1"></span> <span class="rounded-0"></span> </div> </body> </html>
Another set of .rounded-* classes can be used to set one of the sides of the element as rounded. Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Borders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> <style> span { display: inline-block; width: 100px; height: 100px; margin: 10px; background-color: #440d0d; } </style> </head> <body> <div class="container mt-3"> <h4>Borders - Rounded side</h4><br> <p>Set the side to be with rounded corners</p><br> <span class="rounded-start-pill"></span> <span class="rounded-start-2"></span> <span class="rounded-bottom-1"></span> <span class="rounded-5 rounded-top-0"></span> <span class="rounded-end-circle"></span> </div> </body> </html>