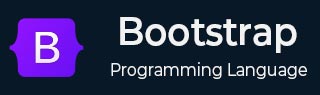
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Placeholders
This chapter discusses about placeholders. A placeholder refers to a temporary text or image that is displayed in an input field or container until the user enters some data or the actual content is loaded.
Placeholders are commonly used to provide hints or examples of the expected input format. They are used for the enhancement and customization of the application.
Some key points to remember:
Placeholders are only displayed when the input field or container is empty.
The placeholders provide visual cues and improve the user experience.
How it works
Placeholders can be created using the class .placeholder and a grid column, such as .col-6 to set the width of the placeholder.
Once some content is been added to the textarea, the placeholder disappears and is replaced by the entered data.
Additional styling can be applied to the .btns via ::before, such that the height is respected.
The same pattern can be extended in other cases as well, or you can add
within an element to show the height, when actual text is added.
Create placeholders
Here's an example of how to create placeholders:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Placeholders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container"> <h4>Placeholders example</h4> <div class="card"> <img src="/bootstrap/images/template.jpg" class="card-img-top" alt="..."> <div class="card-body"> <h5 class="card-title">Card without placeholders</h5> <p class="card-text">An example showing a card without the placeholders.</p> <a href="#" class="btn btn-primary">Go</a> </div> </div> <div class="card" aria-hidden="true"> <img src="/bootstrap/images/template.jpg" class="card-img-top" alt="..."> <div class="card-body"> <h5 class="card-title"> Card with placeholders </h5> <p class="card-text"> <span class="placeholder col-2"></span> <span class="placeholder col-4"></span> <span class="placeholder col-6"></span> <span class="placeholder col-5"></span> <span class="placeholder col-8"></span> </p> <a class="btn btn-primary disabled placeholder col-2"></a> </div> </div> </body> </html>
In order to make the placeholder hidden from the screen readers, use aria-hidden="true". Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Placeholders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container"> <h4>Placeholder hidden</h4> <p><span class="placeholder col-7"></span></p> <p><span class="placeholder col-4"></span></p> <p aria-hidden="true"> <span class="placeholder col-6"></span> </p> <span class="placeholder col-4"></span> </div> </body> </html>
Placeholder - Width
Width of the placeholders can be modified through grid column classes, such as col-6, utilities such as w-50, or inline styles such as style="width: 75%;".
Let us see the example for modifying the width of the placeholders:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Placeholders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container"> <h4>Placeholders example - width</h4> <p><span class="placeholder col-2"></span></p> <p><span class="placeholder col-4 w-25"></span></p> <p><span class="placeholder col-4 w-50"></span></p> <p><span class="placeholder" style="width: 75%;"></span></p> </div> </body> </html>
Placeholder - color
Color can be added to a placeholder using a custom color or utility class. The .placeholder uses currentColor by default.
Let us see an example for setting the color to the placeholder:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Placeholders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container"> <h4>Placeholders example - color</h4> <p><span class="placeholder col-7"></span></p> <p><span class="placeholder col-4 bg-primary"></span></p> <span class="placeholder col-4 bg-info"></span> <p><span class="placeholder col-6 bg-danger"></span></p> <p><span class="placeholder col-8 bg-warning"></span></p> </div> </body> </html>
Placeholder - sizing
Sizing of placeholders can be customized using the modifiers, such as .placeholder-lg, .placeholder-sm, or .placeholder-xs, as the the size of the placeholders depend on the style of the parent element.
Let us see an example of sizing of placeholders:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Placeholders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container"> <h4>Placeholders example - sizing</h4> <p><span class="placeholder col-2 "></span></p> <p><span class="placeholder col-4 placeholder-lg"></span></p> <p><span class="placeholder col-4 placeholder-sm"></span></p> <p><span class="placeholder col-6 placeholder-xs"></span></p> </div> </body> </html>
Placeholder - animation
Placeholders can be animated using the classes .placeholder-glow or .placeholder-wave.
Let us see an example of adding animation to the placeholders:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Placeholders</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container"> <h4>Placeholders example - animation</h4> <!--placeholder-glow--> <p class="placeholder-glow"> <span class="placeholder col-10"></span> </p> <!--no animation--> <p><span class="placeholder col-10 "></span></p> <!--placeholder-wave--> <p class="placeholder-wave"> <span class="placeholder col-10"></span> </p> </div> </body> </html>