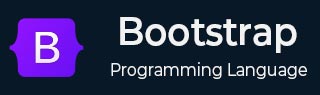
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Text
This chapter will discuss about common Bootstrap text utilities. You can customize text alignment, weight, line height, wrapping, font size, and other formatting options using Bootstrap utility classes.
Text alignment
Text alignment classes provide convenient ways to align text to different components. These classes include responsive options that align the text to the start, end, or center positions, using the same viewport width breakpoints as the grid system.
Note: Kindly resize the browser to see the alignment of text relative to the specific viewport.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <p class="text-start text-info">Text aligned at starting position across all viewports sizes.</p> <p class="text-center text-danger">Text aligned at center position across all viewports sizes.</p> <p class="text-end text-warning">Text aligned at end position across all viewports sizes.</p> <p class="text-sm-start text-primary">Text aligned at start on viewports sized SM (small) or wider.</p> <p class="text-md-start text-primary">Text aligned at start on viewports sized MD (medium) or wider.</p> <p class="text-lg-start text-primary">Text aligned at start on viewports sized LG (large) or wider.</p> <p class="text-xl-start text-primary">Text aligned at start on viewports sized XL (extra-large) or wider.</p> </body> </html>
Bootstrap does not provide utility classes for justified text, which means that while justified text may appear more visually appealing, it can make word spacing more random and consequently more difficult to read.
Text wrapping and overflow
To achieve text wrapping, apply the .text-wrap class to the text.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="badge bg-warning text-wrap" style="width: 8rem;"> This sentence should be wrapped. </div> </body> </html>
You can stop text from wrapping by using the .text-nowrap class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="text-nowrap bg-body-secondary border" style="width: 8rem;"> This sentence should overflow the parent. </div> </body> </html>
Word break
To avoid breaking your components layout with long strings of text, use .text-break to apply word-wrap: break-word and word-break: break-word.
To enhance browser compatibility, use word-wrap instead of overflow-wrap. Additionally, include the deprecated word-break: break-word to prevent problems with flex containers.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <p class="text-break text-primary">TTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTT</p> </body> </html>
Word breaking is not possible in Arabic, this is the most commonly used RTL language. The .text-break feature has been eliminated from our RTL compiled CSS
Text transform
Text capitalization classes can be used to convert text case in components.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <p class="text-lowercase text-danger">This is a lower case text.</p> <p class="text-uppercase text-info"> This is a upper case text.</p> <p class="text-capitalize text-warning">This is a capitalized text.</p> </body> </html>
Note how does the .text-capitalize class modifies the initial letter of every word, without changing the case of other letters.
Font size
You can easily modify the font-size of text. Unlike heading classes (such as .h1–.h6) that include font-weight and line-height, these utilities focus only on font-size.
The size of these utilities matches HTML's heading elements, with a decrease in size as the number increases.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <p class="fs-1 text-info">This is a text of .fs-1 font size.</p> <p class="fs-2 text-info">This is a text of .fs-2 font size.</p> <p class="fs-3 text-info">This is a text of .fs-3 font size.</p> <p class="fs-4 text-info">This is a text of .fs-4 font size.</p> <p class="fs-5 text-info">This is a text of .fs-5 font size.</p> <p class="fs-6 text-info">This is a text of .fs-6 font size.</p> </body> </html>
Modify the $font-sizes Sass map to customize the available font sizes.
Font weight and italics
Use the below utilities to change font-weight or font-style of text:
font-weight utilities are abbreviated as .fw-*
font-style utilities are abbreviated as .fst-*
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <p class="fw-bold text-primary">This is a bold text.</p> <p class="fw-bolder text-primary">This is a bolder weight text (relative to the parent element).</p> <p class="fw-semibold text-primary">This is a semibold weight text.</p> <p class="fw-medium text-secodary">This is a medium weight text.</p> <p class="fw-normal text-secodary">This is a normal weight text.</p> <p class="fw-light text-secodary">This is a light weight text.</p> <p class="fw-lighter text-danger">This is a lighter weight text (relative to the parent element).</p> <p class="fst-italic text-danger">This is a italic text.</p> <p class="fst-normal text-danger">This is a text with normal font style.</p> </body> </html>
Line height
To change the height of the line, use .lh-* utility classes.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <p class="lh-1 text-primary">This is a long text that demonstrates how the utilities affect the line height of an element. Classes can be added to the element directly or occasionally to its parent element. With the help of utility API, these classes can be modified as required.</p> <p class="lh-sm text-secondary">This is a long text that demonstrates how the utilities affect the line height of an element. Classes can be added to the element directly or occasionally to its parent element. With the help of utility API, these classes can be modified as required.</p> <p class="lh-base text-warning">This is a long text that demonstrates how the utilities affect the line height of an element. Classes can be added to the element directly or occasionally to its parent element. With the help of utility API, these classes can be modified as required.</p> <p class="lh-lg text-info">This is a long text that demonstrates how the utilities affect the line height of an element. Classes can be added to the element directly or occasionally to its parent element. With the help of utility API, these classes can be modified as required.</p> </body> </html>
Monospace
Convert the selected text to a monospace font stack using the .font-monospace class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <p class="font-monospace text-primary">Text in monospace font.</p> </body> </html>
Reset color
Use the .text-reset class to restore the color of a text or link and allow it to inherit the color from its parent.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <p class="text-body-danger"> There is a <a href="#" class="text-reset">reset link</a> in this muted text. </p> <p class="text-body-secondary"> There is a <a href="#">reset link without text-reset class</a> in this muted text. </p> </body> </html>
Text decoration
Use text decoration classes to enhance the appearance of the text in components.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Text</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <p class="text-decoration-underline text-danger">There is a line underneath in this paragraph.</p> <p class="text-decoration-line-through text-danger">A line runs through this text.</p> <a href="#" class="text-decoration-none">There is no text decoration on this link.</a> </body> </html>