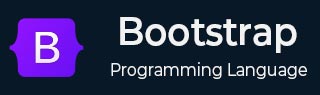
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Carousel
This chapter discusses about the Bootstrap component carousel. The Carousel component in Bootstrap is used to display a rotating set of images or content in a slideshow format.
Overview
The component provides multiple options for customization, including slide transitions, interval timing, and navigation controls.
It allows users to easily navigate through the content and is commonly used for showcasing products or featured content on a website.
To ensure optimal performance, carousels require manual initialization through the carousel constructor method. If not initialized, certain event listeners (specifically those necessary for touch/swipe support) will remain unregistered until a control or indicator is activated by the user.
The carousel with the data-bs-ride="carousel" attribute is initialized automatically on page load. No need to explicitly initialize such carousels.
Bootstrap does not support nested carousels. They can also often cause usability and accessibility challenges.
The animation effect of the carousel component depends on the prefers-reduced-motion media query.
Let us see an example of a basic carousel:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Carousel</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h1 class="text-center">Carousel example</h1> <div id="carouselExample" class="carousel slide"> <center> <div class="carousel-inner bg-secondary"> <div class="carousel-item active"> <p class="text-bg-danger display-6">Slide 1</p> <img src="/bootstrap/images/tutimg.png" alt="GFG" width="600" height="300" class="d-block w-50" alt="..."> </div> <div class="carousel-item"> <p class="text-bg-danger display-6">Slide 2</p> <img src="/bootstrap/images/profile.jpg" alt="GFG" width="300" height="400" class="d-block w-50" alt="..."> </div> <div class="carousel-item"> <p class="text-bg-danger display-6">Slide 3</p> <img src="/bootstrap/images/scenery.jpg" alt="GFG" width="300" height="500" class="d-block w-50" alt="..."> </div> </div> <button class="carousel-control-prev" type="button" data-bs-target="#carouselExample" data-bs-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="visually-hidden">Previous</span> </button> <button class="carousel-control-next" type="button" data-bs-target="#carouselExample" data-bs-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="visually-hidden">Next</span> </button> </center> </div> </body> </html>
Points to remember:
The slide dimensions are not automatically normalized in carousels.
You need to use additional utilities or custom styles to size the content in carousels.
The previous/next controls and indicators are not explicitly required, as carousels support them. Add and customize the controls as per your requirement.
Do not forget to add the .active class to one of the slides, else the carousel will not be visible.
Ensure to set a unique id on the .carousel for optional controls, in case you are using multiple carousels on a single page.
You must add the data-bs-target attribute to the control and indicator elements or href for links, that matches the id of the .carousel element.
Indicators
Indicators can be added along with the previous/next controls, such that the user has the access to jump directly to a specific slide.
Let us see an example for adding indicators:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Carousel</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h1 class="text-center">Carousel Indicators</h1> <div id="carouselExample" class="carousel slide"> <center> <div id="carouselExampleIndicators" class="carousel slide bg-secondary"> <div class="carousel-indicators text-dark"> <button type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide-to="0" class="active" aria-current="true" aria-label="Slide 1"><h3>1</h3></button> <button type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide-to="1" aria-label="Slide 2"><h3>2</h3></button> <button type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide-to="2" aria-label="Slide 3"><h3>3</h3></button> </div> <div class="carousel-inner"> <div class="carousel-item active"> <img src="/bootstrap/images/scenery.jpg" alt="GFG" width="400" height="300" alt="..."> </div> <div class="carousel-item"> <img src="/bootstrap/images/scenery2.jpg" alt="GFG" width="400" height="300" alt="..."> </div> <div class="carousel-item"> <img src="/bootstrap/images/scenery3.jpg" alt="GFG" width="400" height="300" alt="..."> </div> </div> <button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="visually-hidden">Previous</span> </button> <button class="carousel-control-next" type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="visually-hidden">Next</span> </button> </div> </center> </div> </body> </html>
Captions
Captions can be added to the slides with the .carousel-caption element within any .carousel-item. The caption can be hidden using the class .d-none and can be made visible using the classes .d-{breakpoint}-block.
Let us see an example for adding captions:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Carousel</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h1 class="text-center">Carousel Captions</h1> <div id="carouselExampleCaptions" class="carousel slide bg-secondary"> <center> <div id="carouselExampleCaptions" class="carousel slide bg-secondary"> <div class="carousel-indicators text-dark"> <button type="button" data-bs-target="#carouselExampleCaptions" data-bs-slide-to="0" class="active" aria-current="true" aria-label="Slide 1"><h3>1</h3></button> <button type="button" data-bs-target="#carouselExampleCaptions" data-bs-slide-to="1" aria-label="Slide 2"><h3>2</h3></button> <button type="button" data-bs-target="#carouselExampleCaptions" data-bs-slide-to="2" aria-label="Slide 3"><h3>3</h3></button> </div> <div class="carousel-inner"> <div class="carousel-item active"> <img src="/bootstrap/images/template.jpg" alt="GFG" width="400" height="300" alt="..."> <div class="carousel-caption text-white"> <h5>Caption for first slide</h5> <p>This is the first slide of the carousel component.</p> </div> </div> <div class="carousel-item"> <img src="/bootstrap/images/template.jpg" alt="GFG" width="400" height="300" alt="..."> <div class="carousel-caption text-white"> <h5>Caption for second slide</h5> <p>This is the second slide of the carousel component.</p> </div> </div> <div class="carousel-item"> <img src="/bootstrap/images/template.jpg" alt="GFG" width="400" height="300" alt="..."> <div class="carousel-caption text-white"> <h5>Caption for third slide</h5> <p>This is the third slide of the carousel component.</p> </div> </div> </div> <button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleCaptions" data-bs-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="visually-hidden">Previous</span> </button> <button class="carousel-control-next" type="button" data-bs-target="#carouselExampleCaptions" data-bs-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="visually-hidden">Next</span> </button> </div> </center> </div> </body> </html>
Crossfade
To apply a fade transition to your carousel slides instead of a slide, include .carousel-fade. However, if your carousel content comprises solely text slides, it may be necessary to add .bg-body or utilize custom CSS for appropriate crossfading in .carousel-items.
Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Carousel</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h1 class="text-center">Carousel Animation - Fade</h1> <div id="carouselExampleFade" class="carousel slide"> <center> <div id="carouselExampleFade" class="carousel slide carousel-fade bg-secondary"> <div class="carousel-inner"> <div class="carousel-item active"> <img src="/bootstrap/images/template.jpg" alt="GFG" width="600" height="500" alt="..."> <div class="carousel-caption text-white"> <h2>First slide</h2> </div> </div> <div class="carousel-item"> <img src="/bootstrap/images/profile.jpg" alt="GFG" width="600" height="500" alt="..."> <div class="carousel-caption text-white"> <h2>Second slide</h2> </div> </div> <div class="carousel-item"> <img src="/bootstrap/images/tutimg.png" alt="GFG" width="600" height="500" alt="..."> <div class="carousel-caption text-white"> <h2>Third slide</h2> </div> </div> </div> <button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleFade" data-bs-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="visually-hidden">Previous</span> </button> <button class="carousel-control-next" type="button" data-bs-target="#carouselExampleFade" data-bs-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="visually-hidden">Next</span> </button> </div> </center> </div> </body> </html>
Autoplaying carousel
The carousels can be made to autoplay on page load by setting the ride option to carousel.
While you hover with the mouse, the autoplaying carousels pause automatically. You can control this behavior with the option pause.
The carousel will stop cycling when the webpage is not v isible (either the browser window is inactive or minimized). in case of browsers support that support the page visibility API.
To ensure accessibility, it is advised to steer clear of autoplaying carousels. Should your page contain such a feature, we advise adding a separate button or control to enable explicit pausing or stopping of the carousel.
Let us see an example for autoplaying carousel:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Carousel</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h1 class="text-center">Carousel Autoplaying</h1> <center> <div id="carouselExampleRide" class="carousel slide bg-secondary" data-bs-ride="carousel"> <div class="carousel-inner"> <div class="carousel-item active"> <img src="/bootstrap/images/tutimg.png" alt="GFG" width="400" height="300" alt="..."> <div><p><h3>First slide</h3></p></div> </div> <div class="carousel-item"> <img src="/bootstrap/images/profile.jpg" alt="GFG" width="400" height="300" alt="..."> <div><p><h3>Second slide</h3></p></div> </div> <div class="carousel-item"> <img src="/bootstrap/images/template.jpg" alt="GFG" width="400" height="300" alt="..."> <div><p><h3>Third slide</h3></p></div> </div> </div> <button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleRide" data-bs-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="visually-hidden">Previous</span> </button> <button class="carousel-control-next" type="button" data-bs-target="#carouselExampleRide" data-bs-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="visually-hidden">Next</span> </button> </div> </center> </body> </html>
When the ride option is set to true, instead of carousel, the carousel will not automatically start to cycle on page load. It will start only after the user's interaction.
Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <!DOCTYPE html> <html> <head> <title>Bootstrap - Carousel</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h1 class="text-center">Carousel Autoplaying on Ride</h1> <center> <div id="carouselExampleRide" class="carousel slide bg-secondary" data-bs-ride="true"> <div class="carousel-inner"> <div class="carousel-item active"> <img src="/bootstrap/images/scenery.jpg" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>First slide</h3></p></div> </div> <div class="carousel-item"> <img src="/bootstrap/images/scenery2.jpg" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>Second slide</h3></p></div> </div> <div class="carousel-item"> <img src="/bootstrap/images/scenery3.jpg" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>Third slide</h3></p></div> </div> </div> <button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleRide" data-bs-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="visually-hidden">Previous</span> </button> <button class="carousel-control-next" type="button" data-bs-target="#carouselExampleRide" data-bs-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="visually-hidden">Next</span> </button> </div> </center> </body> </html>
Individual .carousel-item interval
To a .carousel-item add data-bs-interval="" to change the amount of time to set between automatically cycling to next item.
Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Carousel</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h1 class="text-center">Carousel Autoplay Time Interval</h1> <center> <div id="carouselExampleInterval" class="carousel slide bg-secondary" data-bs-ride="carousel"> <div class="carousel-inner"> <div class="carousel-item active" data-bs-interval="2000"> <img src="/bootstrap/images/tutimg.png" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>First slide</h3></p></div> </div> <div class="carousel-item" data-bs-interval="2000"> <img src="/bootstrap/images/profile.jpg" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>Second slide</h3></p></div> </div> <div class="carousel-item"> <img src="/bootstrap/images/template.jpg" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>Third slide</h3></p></div> </div> </div> <button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleInterval" data-bs-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="visually-hidden">Previous</span> </button> <button class="carousel-control-next" type="button" data-bs-target="#carouselExampleInterval" data-bs-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="visually-hidden">Next</span> </button> </div> </center> </body> </html>
Autoplaying carousel without controls
Carousel can be played without any controls as well.
Here is an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Carousel</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h1 class="text-center">Carousel Autoplay without controls</h1> <center> <div id="carouselExampleSlideOnly" class="carousel slide bg-secondary" data-bs-ride="carousel"> <div class="carousel-inner"> <div class="carousel-item active" data-bs-interval="2000"> <img src="/bootstrap/images/tutimg.png" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>First slide</h3></p></div> </div> <div class="carousel-item" data-bs-interval="2000"> <img src="/bootstrap/images/profile.jpg" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>Second slide</h3></p></div> </div> <div class="carousel-item"> <img src="/bootstrap/images/template.jpg" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>Third slide</h3></p></div> </div> </div> </div> </center> </body> </html>
Disable touch swiping
Touchscreen devices can swipe left or right to switch between slides on carousels. Turning off the touch option can disable this feature, by setting the value as false.
Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html> <head> <title>Bootstrap - Carousel</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h1 class="text-center">Carousel disable touch swiping</h1> <center> <div id="carouselExampleControlsNoTouching" class="carousel slide bg-secondary" data-bs-touch="false"> <div class="carousel-inner"> <div class="carousel-item active"> <img src="/bootstrap/images/profile.jpg" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>First slide</h3></p></div> </div> <div class="carousel-item"> <img src="/bootstrap/images/template.jpg" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>Second slide</h3></p></div> </div> <div class="carousel-item"> <img src="/bootstrap/images/profile.jpg" alt="GFG" width="600" height="500" alt="..."> <div><p><h3>Third slide</h3></p></div> </div> </div> <button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleControlsNoTouching" data-bs-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="visually-hidden">Previous</span> </button> <button class="carousel-control-next" type="button" data-bs-target="#carouselExampleControlsNoTouching" data-bs-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="visually-hidden">Next</span> </button> </div> </center> </body> </html>
Dark variant
Attention! The use of dark variants for components has been deprecated in v5.3.0 due to the implementation of color modes. Rather than adding .carousel-dark, set data-bs-theme="dark" on the root element, a parent wrapper, or the component itself.