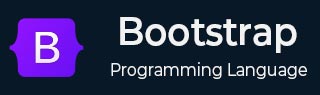
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Grid system
This chapter will discuss about Bootstrap grid system. In Bootstrap, Grid system allows up to 12 columns across the page and is built with flexbox
Basic example
Bootstrap's grid system is a responsive layout tool that uses containers, rows, and columns to align content.
Use a .container class for a responsive fixed width container.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row mt-2"> <div class="col p-2 bg-primary"> First Column </div> <div class="col p-2 bg-warning"> Second Column </div> <div class="col p-2 bg-info"> Third Column </div> </div> </div> </body> </html>
How it works
Bootstrap grid supports six responsive breakpoints. Breakpoints affect all sizes above it (such as sm, md, lg, xl, xxl), allowing you to control container and column sizing and behavior at each breakpoint.
Containers center and horizontally pad your content. Use .container for responsive pixel width or .container-fluid for full width across all viewport and device sizes. For various breakpoints the responsive container classes are used.
Rows are wrappers for columns. Each column contains horizontal padding. This padding is then applied to rows with negative margins. In this manner, all the content in the columns, is left-aligned.
Rows supports modification classes for uniform column sizing column sizing and gutter classes for changing the spacing of your text.
Columns are incredibly flexible. You can create various elements combination with any number of columns using one of the 12 template columns available per row.
Column widths are set in percentages for fluid and relative sizing to parent element.
Gutters are responsive and customizable. They are available for all the viewports and are of same size as margin and padding. You can modify gutters by using the .gx-* (for horizontal gutters), .gy-* (for vertical gutters), .g-* (for all gutters) and .g-0 to remove gutters.
To create more semantic markup, you can use predefined grid's source Sass mixins.
There are six classes in Bootstrap Grid System:
Extra small (.col-xs)
Small (.col-sm-)
Medium (.col-md-)
Large (.col-lg-)
Extra large (.col-xl-)
Extra extra large (.col-xxl-)
How the grid varies over these breakpoints is shown in the below table:
Extra small <576px |
Small ≥576px |
Medium ≥768px |
Large ≥992px |
X-Large ≥1200px |
XX-Large ≥1400px |
|
---|---|---|---|---|---|---|
Container max-width | None (auto) | 540px | 720px | 960px | 1140px | 1320px |
Class prefix | .col- | .col-sm- | .col-md- | .col-lg- | .col-xl- | .col-xxl- |
# of columns | 12 | - | - | - | - | - |
Gutter width | 1.5rem (.75rem margin on both sides.) | - | - | - | - | - |
Custom gutters | Yes | - | - | - | - | - |
Nestable | Yes | - | - | - | - | - |
Column ordering | Yes | - | - | - | - | - |
Auto-layout columns
For easy column sizing without an explicit numbered class, use breakpoint-specific column classes like .col-sm-6.
Equal-width
Use equal-width grid system to create the grid in equal sizes.
Two grid layouts work on any device size from xs to xxl. For each breakpoint, you can add many unit-less classes, and each column/row will have the same width.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <h5>Equal columns</h5> <div class="row mt-2"> <div class="col p-2 bg-primary"> First Column </div> <div class="col p-2 bg-info"> Second Column </div> </div> <h5>Equal rows</h5> <div class="col mt-2"> <div class="row p-2 bg-warning text-white"> First Row </div> <div class="row p-2 bg-secondary text-white"> Second Row </div> <div class="row p-2 bg-success text-white"> Third Row </div> </div> </div> </body> </html>
Setting one column width
You can choose one column width and other columns automatically resize around it.
Use grid classes, mixins, or inline widths. You can resize the other columns no matter the width of the center column.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row mt-2"> <div class="col-6 p-2 bg-primary text-white"> First Column (col-6) </div> <div class="col-3 p-2 bg-secondary text-white"> Second Column (col-3) </div> <div class="col p-2 bg-warning text-white"> Third Column (col) </div> </div> </div> </body> </html>
Variable width content
Use col-{breakpoint}-auto classes to size columns according to the content's natural width.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row justify-content-md-center mt-2"> <div class="col col-lg-2 p-2 bg-primary "> First Column </div> <div class="col-md-auto p-2 bg-info "> Second column with variable width content. </div> <div class="col col-lg-2 p-2 bg-warning"> Third Column </div> </div> </div> </body> </html>
Responsive classes
In Bootstrap, grid has six tiers of predefined classes which is used to create complex responsive layouts. You can customize the columns/row to small, medium, large, or extra-large devices.
All breakpoints
Use the .col and .col-* classes for grids that are consistent across all sizes of devices. If you require a column of a specific size then use a numbered class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <h5>Columns</h5> <div class="row mt-2"> <div class="col p-2 bg-primary">First Column</div> <div class="col p-2 bg-warning">Second Column</div> <div class="col p-2 bg-light">Third Column</div> </div> <h5>Rows</h5> <div class="col mt-2"> <div class="row-9 p-2 bg-info">First Row</div> <div class="row-3 p-2 bg-success">Second Row</div> </div> </div> </body> </html>
Stacked to horizontal
Use .col-sm-* classes to build a simple grid system that is stacked on extra small and small devices and becomes horizontal on larger devices.
Note: Resize the browser to check the effect on various devices.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <h5>Columns</h5> <div class="row mt-2"> <div class="col-sm-6 p-2 bg-primary text-white">First Column</div> <div class="col-sm-3 p-2 bg-success text-white">Second Column</div> <div class="col-sm-2 p-2 bg-dark text-white">Third Column</div> </div> <h5>Rows</h5> <div class="col mt-2"> <div class="row-sm p-2 bg-info">First row</div> <div class="row-sm p-2 bg-warning">Second row</div> </div> </div> </body> </html>
Mix and match
Use a combination of various classes for each tier to easily stack the columns in some grid tiers according to your needs.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row mt-2"> <div class="col-sm-4 p-2 bg-primary text-white">First column of col-sm-4</div> <div class="col-3 p-2 bg-secondary text-white">Second column of col-3</div> </div> <div class="row mt-2"> <div class="col-4 col-md-2 p-2 bg-warning text-white">First column of col-4 col-md-2</div> <div class="col-4 col-md-2 p-2 bg-secondary text-white">Second column of col-4 col-md-2</div> <div class="col-4 col-md-2 p-2 bg-info text-white">Third column of col-4 col-md-2</div> </div> <div class="row mt-2"> <div class="col-sm-3 p-2 bg-dark text-white">First column of col-sm-3</div> <div class="col-md-6 p-2 bg-success text-white">Second column of col-md-6</div> </div> </div> </body> </html>
Row columns
Bootstrap grid provides row column classes to create simple grid layouts.
Individual rows are included in .row* class.
Individual columns are included in .col* class.
Use .row-cols-* class to set the number of columns in a single row.
Use .row-cols-auto to adjust the column's size based on its content.
Create two different columns using .row-cols-2 class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row row-cols-2"> <div class="col bg-info p-2">First Row First Column</div> <div class="col bg-primary p-2">First Row Second Column</div> <div class="col bg-warning p-2">Second Row First Column</div> </div> </div> </body> </html>
Create three different columns using .row-cols-3 class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center mt-2"> <div class="row row-cols-3 mt-2"> <div class="col bg-info p-2">First Row First Column</div> <div class="col bg-light p-2">First Row Second Column</div> <div class="col bg-primary p-2">First Row Third Column</div> <div class="col bg-secondary p-2">Second Row First Column</div> <div class="col bg-success p-2">Second Row Second Column</div> <div class="col bg-warning p-2">Second Row Third Column</div> </div> </div> </body> </html>
Create grids of rows and columns using row-cols-auto class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row row-cols-auto"> <div class="col bg-primary p-2">First Row First Column</div> <div class="col bg-secondary p-2">First Row Second Column</div> <div class="col bg-warning p-2">First Row Third Column</div> <div class="col bg-info p-2">First Row Fourth Column</div> <div class="col bg-success p-2">First Row Fifth Column</div> <div class="col bg-light p-2">First Row sixth Column</div> <div class="col bg-danger p-2">Second Row First Column</div> </div> </div> </body> </html>
Create four different columns using row-cols-4 class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row row-cols-4"> <div class="col bg-primary p-2">First Row First Column</div> <div class="col bg-secondary p-2">First Row Second Column</div> <div class="col bg-warning p-2">First Row Third Column</div> <div class="col bg-info p-2">First Row Fourth Column</div> <div class="col bg-light p-2">Second Row First Column</div> <div class="col bg-danger p-2">Second Row Second Column</div> </div> </div> </body> </html>
Using row-cols-1, row-cols-sm-3 and row-cols-md-6 classes.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row row-cols-1 row-cols-sm-3 row-cols-md-6 mt-2"> <div class="col bg-primary p-2">First Row First Column</div> <div class="col bg-light p-2">First Row Second Column</div> <div class="col bg-warning p-2">First Row Third Column</div> <div class="col bg-info p-2">First Row Fourth Column</div> <div class="col bg-danger p-2">First Row Fifth Column</div> </div> </div> </body> </html>
Using the row-cols() Sass mixin.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> <style> .custom_grid{ @media row-cols(2); @media media-breakpoint-up(lg) { @media row-cols(6); } } </style> </head> <body> <div class="container custom_grid text-center"> <div class="row"> <div class="col bg-primary p-2">First Row First Column</div> <div class="col bg-light p-2">First Row Second Column</div> <div class="col bg-warning p-2">First Row Third Column</div> <div class="col bg-info p-2">First Row Fourth Column</div> <div class="col bg-danger p-2">First Row Fifth Column</div> </div> </div> </body> </html>
Nesting
A nesting grid system adds rows and columns of the grid in single cell of an existing grid. The nested rows should consist of a group of columns whose total does not exceed 12 (it is not necessary to utilize all 12 columns).
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Grid</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row mt-2"> <div class="col-sm bg-primary p-2"> First Column </div> <div class="col-sm bg-info p-2"> <div class="container"> <div class="row"> <div class="col col-sm bg-light"> Second Column </div> <div class="col col-sm bg-light"> Second Column </div> </div> </div> </div> <div class="col-sm bg-primary p-2"> Third Column </div> </div> </div> </body> </html>