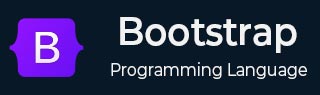
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Gutters
This chapter will discuss about Bootstrap gutters. Gutter provides padding between your columns. Gutters are used to responsively space and align content.
How it works
Gutters are generated by horizontal padding, and are spaces between the column content. Align the content using padding-right and padding-left on each column.
Gutters begin at a width of 1.5 rem (24px), hence allows us to align grid to the scale of padding and margin spacers.
Adjust gutters with breakpoint-specific classes to change horizontal, vertical, and all other gutters.
Horizontal gutters
.gx-* classes manage the widths of horizontal gutters, and if larger gutters are used, the parent .container or .container-fluid may require adjustment to prevent overflow. This can be done using a padding utility, such as .px-4 as demonstarted in below example.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Gutters</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container px-4 text-center mt-2"> <div class="row gx-5"> <div class="col"> <div class="p-2 bg-info">First Column</div> </div> <div class="col"> <div class="p-2 bg-warning">Second Column</div> </div> </div> </div> </body> </html>
Using overflow functionality
Adding a wrapper with the .overflow-hidden class to .row is another option.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Gutters</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container overflow-hidden text-center"> <div class="row gx-5 mt-2"> <div class="col"> <div class="p-2 bg-info">First Column</div> </div> <div class="col"> <div class="p-2 bg-warning">Second Column</div> </div> </div> </div> </body> </html>
Vertical gutters
The vertical gutter is used for responsive spacing, padding between columns, and aligning content with the grid.
Use .gy-* classes to control vertical gutter widths in a row when column wrapping occurs.
They can cause some overflow below the .row at the end of a page, like the horizontal gutters. To fix this, add a wrapper with .overflow-hidden class around a .row.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Gutters</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container overflow-hidden text-center mt-2"> <div class="row gy-5"> <div class="col-6"> <div class="p-2 bg-info">First Column</div> </div> <div class="col-6"> <div class="p-2 bg-warning">Second Column</div> </div> <div class="col-6"> <div class="p-2 bg-info">Third Column</div> </div> <div class="col-6"> <div class="p-2 bg-warning">Fourth Column</div> </div> </div> </div> </body> </html>
Horizontal and vertical gutters
To control the horizontal and vertical grid gutters, use .g-* classes. Use smaller gutter width. So, we won't need of .overflow-hidden wrapper class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Gutters</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row g-2 mt-2"> <div class="col-6"> <div class="p-2 bg-info">First Column</div> </div> <div class="col-6"> <div class="p-2 bg-warning">Second Column</div> </div> <div class="col-6 "> <div class="p-2 bg-info">Third Column</div> </div> <div class="col-6"> <div class="p-2 bg-warning">Fourth Column</div> </div> </div> </div> </body> </html>
Row columns gutters
Gutter classes can be added to row columns with responsive design. Responsive row columns and responsive gutter classes are used in the example below:
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Gutters</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container text-center"> <div class="row row-cols-2 row-cols-lg-3 g-2 g-lg-3 mt-2"> <div class="col"> <div class="p-3 bg-info">First Row column</div> </div> <div class="col"> <div class="p-3 bg-warning">Second Row column</div> </div> <div class="col"> <div class="p-3 bg-info">Third Row column</div> </div> <div class="col"> <div class="p-3 bg-warning">Fourth Row column</div> </div> <div class="col"> <div class="p-3 bg-info">Fifth Row column</div> </div> <div class="col"> <div class="p-3 bg-warning">Sixth Row column</div> </div> </div> </div> </body> </html>
No gutters
Remove gutters between columns with .g-0 in grid classes. This removes negative margins from .row and horizontal padding from immediate children columns.
Remove the parent .container or .container-fluid to create an edge-to-edge design and add .mx-0 to the .row to prevent overflow.
No gutters eliminates margin and padding for both row and columns.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Gutters</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="row g-0 text-center mt-2"> <div class="col-sm-4 col-md-6 p-2 bg-info">First Column</div> <div class="col-4 col-md-3 p-2 bg-warning">Second Column</div> </div> </body> </html>