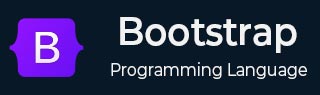
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Popovers
This chapter will discuss about popovers in Bootstrap. A popover typically contains additional information, context, or actions related to the triggering element.
The popover may contain text, images, links, buttons, or other content, depending on its purpose and context. Bootstrap provides built-in popover components that can be easily integrated into web applications.
Things to remember while using the popover plug-in:
- As popovers depend on Popper.js, a third party library, for positioning, you must include popper.min.js before bootstrap.js.
- As a dependency, the popovers require the popover plugin.
- You must initialize the popovers first, as popovers are opt-in for performance reasons.
- A popover will never be shown for a zero-length title and content values.
- Triggering popovers will not work on hidden elements.
- Popovers for .disabled or disabled elements must be triggered using a wrapper element.
- To avoid getting popovers centered between the anchors' overall width, use white-space: nowrap; on the <a>
- Before removing any element from the DOM, you must hide the popovers corresponding to them.
Enable Popovers
Initialize all the popovers on a page, by their data-bs-toggle attribute
const popoverTriggerList = document.querySelectorAll('[data-bs-toggle="popover"]') const popoverList = [...popoverTriggerList].map(popoverTriggerEl => new bootstrap.Popover(popoverTriggerEl))
Creating a Popover
Add the data-bs-toggle="popover" attribute to an element, to create a popover.
The data-bs-toggle attribute defines the popover.
The title attribute defines the title of the popover
The data-content attribute defines the content to be shown in the respective popover.
Example
Let's see an example of creating a popover:
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Popover</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <link href="https://getbootstrap.com/docs/5.3/assets/css/docs.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Bootstrap creation</h4><br><br> <button type="button" class="btn btn-primary" data-bs-toggle="popover" data-bs-placement="top" data-bs-title="Popover" data-bs-content="It is a new popover."> Click to view popover </button> <script> const popoverTriggerList = document.querySelectorAll('[data-bs-toggle="popover"]') const popoverList = [...popoverTriggerList].map(popoverTriggerEl => new bootstrap.Popover(popoverTriggerEl)) </script> </body> </html>
Positioning of a Popover
There are four options available for the positioning of the popover: left, right, top and bottom aligned.
By default, the popover will appear on the right of the element.
The data-bs-placement attribute is used to set the position of the popover.
Example
Let's see an example of setting the position of a popover:
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Popovers</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <link href="https://getbootstrap.com/docs/5.3/assets/css/docs.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Positioning of Popover</h4> <br><br><br> <button type="button" class="btn btn-secondary" data-bs-container="body" data-bs-toggle="popover" data-bs-placement="top" data-bs-content="Top popover"> Popover on top </button> <button type="button" class="btn btn-secondary" data-bs-container="body" data-bs-toggle="popover" data-bs-placement="right" data-bs-content="Right popover"> Popover on right </button> <button type="button" class="btn btn-secondary" data-bs-container="body" data-bs-toggle="popover" data-bs-placement="bottom" data-bs-content="Bottom popover"> Popover on bottom </button> <button type="button" class="btn btn-secondary" data-bs-container="body" data-bs-toggle="popover" data-bs-placement="left" data-bs-content="Left popover"> Popover on left </button> <script> const popoverTriggerList = document.querySelectorAll('[data-bs-toggle="popover"]') const popoverList = [...popoverTriggerList].map(popoverTriggerEl => new bootstrap.Popover(popoverTriggerEl)) </script> </body> </html>
Custom popovers
The appearance of popovers can be customized using a custom class data-bs-custom-class="custom-popover".
Example
Let's see an example of creating a custom popover:
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head&> <title>Bootstrap - Popovers</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Custom Popover</h4><br><br> <!-- Define custom container --> <button type="button" class="btn btn-primary" data-bs-toggle="popover" data-bs-placement="top" data-bs-custom-class="custom-popover" data-bs-title="Custom popover" data-bs-content="It is a custom popover."> Custom popover </button> <script> const popoverTriggerList = document.querySelectorAll('[data-bs-toggle="popover"]') const popoverList = [...popoverTriggerList].map(popoverTriggerEl => new bootstrap.Popover(popoverTriggerEl)) </script> </body> </html>
Dismissible Popover
The popover gets closed when the same element is clicked again, by default. However, the attribute data-bs-trigger="focus" can be used, which will close the popover when clicking outside the element.
For the popover to be dismissed on the next click, it is necessary to use specific HTML code that ensures consistent behavior across different browsers and platforms
Example
Let's see an example of dismissible popover:
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Dismissible Popover</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container mt-3"> <h4>Dismissed on next click - Popover</h4><br> <a href="#" title="Dismissible popover" data-bs-toggle="popover" data-bs-trigger="focus" data-bs-content="Click anywhere in the document to close this popover">Click me</a> </div> <script> const popoverTriggerList = document.querySelectorAll('[data-bs-toggle="popover"]') const popoverList = [...popoverTriggerList].map(popoverTriggerEl => new bootstrap.Popover(popoverTriggerEl)) </script> </body> </html>
Hovering of a Popover
When the mouse pointer is moved over an element and you want a popover to appear on hovering, use the data-bs-trigger="hover" attribute.
Example
Let's see an example of a hovering popover:
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Popover on hover</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container mt-3"> <h4>Hoverable Popover</h4><br> <a href="#" title="Header" data-bs-toggle="popover" data-bs-trigger="hover" data-bs-content="Popover text">Hover over me</a> </div> <script> const popoverTriggerList = document.querySelectorAll('[data-bs-toggle="popover"]') const popoverList = [...popoverTriggerList].map(popoverTriggerEl => new bootstrap.Popover(popoverTriggerEl)) </script> </body> </html>
Popover on disabled elements
For popovers on disabled elements, you may prefer data-bs-trigger="hover focus" so that the popover appears as immediate visual feedback to your users as they may not expect to click on a disabled element.
Example
Let's see an example of a popover on disabled element:
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Popovers</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Popover on Disabled Element</h4><br> <span class="d-inline-block" tabindex="0" data-bs-toggle="popover" data-bs-trigger="hover focus" data-bs-content="Disabled popover"> <button class="btn btn-primary" type="button" disabled>Disabled button</button> </span> <script> const popoverTriggerList = document.querySelectorAll('[data-bs-toggle="popover"]') const popoverList = [...popoverTriggerList].map(popoverTriggerEl => new bootstrap.Popover(popoverTriggerEl)) </script> </body> </html>
Options
An option name can be appended to the class data-bs- and that option can be passed as an attribute, such as data-bs-boundary="{value}".
When passing the options via data attributes, make sure to change the case type to "kebab-case" from "camelCase", such as use data-bs-fallback-placements="[array]" instead of data-bs-fallbackPlacements="[array]".
Example
Here is an example of options added as an attribute to .data-bs- class:
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head&> <title>Bootstrap Popovers - Options</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Popover options</h4><br> <button type="button" class="btn btn-lg btn-success" data-bs-toggle="popover" data-bs-title ="Title added through options and that overrides the title provided" title="Popover description" data-content="Popover desc">Click Me to view</button> <br><br> <script> const popoverTriggerList = document.querySelectorAll('[data-bs-toggle="popover"]') const popoverList = [...popoverTriggerList].map(popoverTriggerEl => new bootstrap.Popover(popoverTriggerEl)) </script> </body> </html>
The table given below shows the various options provided by Bootstrap, to be appended to the class .data-bs- as a data attribute.
Name | Type | Default | Description |
---|---|---|---|
allowList | object | default value | Object that contains allowed attributes and tags. |
animation | boolean | true | A CSS fade transition is applied to the popover. |
boundary | string, element | 'clippingParents' | By default, it’s 'clippingParents' and can accept an HTML Element reference (via JavaScript only). |
container | string, element, false | false | Appends the popover to a specific element. |
customClass | string, function | '' | These classes will be added in addition to any classes specified in the template. To add multiple classes, separate them with spaces: 'class-1 class-2'. |
delay | number, object | 0 | Delay showing and hiding the popover(ms). Object structure is: delay: { "show": 500, "hide": 100 }. |
fallbackPlacements | array | ['top', 'right', 'bottom', 'left'] | Define fallback placements by providing a list of placements in array. |
html | boolean | false | Allow HTML in the popover. |
offset | array, string, function | [0, 0] | Offset of the popover relative to its target. Ex: data-bs-offset="10,20". |
placement | string, function | 'top' | Decides the position of the popover. |
popperConfig | null, object, function | null | To change Bootstrap’s default Popper config. |
sanitize | boolean | true | Enable or disable the sanitization. |
sanitizeFn | null, function | null | You can supply your own sanitize function. |
selector | string, false | false | With a selector, popover objects will be delegated to the specified targets. |
template | string | ' ' |
While creating a popover, use the base HTML. |
title | string, element, function | '' | It refers to the title of the popover. |
trigger | string | 'hover-focus' | Shows how the popover is triggered: click, hover, focus, manual. |