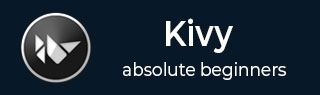
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Windows
The Window class is one of the core classes in the Kivy framework. The application window is constructed with placement of one or more widgets in a certain layout. The build() method normally returns a single root widget, which may be a combination of multiple other widgets arranged in a tree. Instead, the root of the widget tree can be directly added to the application window.
The Window class is defined in the "kivy.core.window" module. It inherits the WindowBase class which is an abstract window widget for any window implementation. A default application window is created with the App object starting its event loop. Note that Kivy supports only one window per application.
Many of the Window properties are read from the Kivy config file ("config.ini" file in KIVY_HOME directory).
The widget dimensions depend upon the size of the default window. The following code in the build() method of App class puts a widget tree in the application window. Note that the root widget is not returned by the build() method. Instead it is added to the Window object's add_widget() method.
box=BoxLayout(orientation='vertical') l=Label(text='Window Properties', font_size=32) box.add_widget(l) b1=ToggleButton(text='Fullscreen') b2=ToggleButton(text='Border') b3=ToggleButton(text='Position') bh=BoxLayout(orientation='horizontal', size_hint=(1, .2)) bh.add_widget(b1) bh.add_widget(b2) bh.add_widget(b3) box.add_widget(bh) Window.add_widget(box)
Events
The Window object can recognize different type of events −
The on_motion event is fired when a new MotionEvent is dispatched.
Window absorbs the touch events on_touch_down, on_touch_move, on_touch_up etc.
The on_close event is fired when the Window is closed.
The on_request_close event occurs when the user wants to end the event loop by pressing the close button on the title bar.
The on_cursor_enter event is fired when the cursor enters the window.
Similarly, the on_cursor_leave event occurs when the cursor leaves the window.
The on_minimize and on_maximize events are fired when the window is minimized and maximized respectively.
The on_restore event Fires when the window is restored.
Similar to touch events, the key events on_key_down and on_key_up events emit the key, scancode, codepoint, modifier when a key is pressed or released respectively.
For the demonstration example in this chapter, let us bind some of the Window events with callback methods.
Window.bind(on_request_close = self.on_close) Window.bind(on_cursor_leave=self.on_leave) Window.bind(on_cursor_enter=self.on_enter)
The on_leave() method is called whenever the mouse pointer leaves the window area.
def on_leave(self, *args): print ("leaving the window")
Similarly, when the mouse enters the window area, the on_enter callback is invoked.
def on_enter(self, *args): print ("Entering the window")
The on_request_close event is raised when the user chooses to close the event loop. If the user presses X button, the following callback asks the user if he wants to quit. You can also make a popup window to appear.
def on_close(self, instance): resp=input("Do you want the window to close?") if resp=='y': Window.close()
Properties
The appearance of the application window is decided by many properties defined in the Window class. Their default values are provided by the config.ini file. However, they can be modified in the application code. Some of the Wiindow properties are as below −
borderless − When set to True, this property removes the window border/decoration.
children − returns the List of the children of this window.
clearcolor − Color used to clear the window. The clear() method uses this property with this color value
from kivy.core.window import Window Window.clearcolor = (1, 0, 0, 1) Window.clear()
custom_titlebar − When set to True, allows the user to set a widget as a titlebar.
fullscreen − This property sets the fullscreen mode of the window. Available options are: True, False, 'auto' and 'fake'.
left , top − Left and Top position of the window. It's an SDL2 property with [0, 0] in the top-left corner.
size − gets/sets the size of the window.
from kivy.core.window import Window Window.size = (720,400)
You can also set the size by modifying the config values −
from kivy.config import Config Config.set('graphics', 'width', '720') Config.set('graphics', 'height', '400') Config.set('graphics', 'resizable', '1')
Let us handle some Window properties. On the application window of this program, we have three toggle buttons. We bind them to certain callbacks.
b1.bind(on_press=self.pressed) b2.bind(on_press=self.bordered) b3.bind(on_press=self.positioned)
The pressed() method toggles the fullscreen state between full screen and normal.
def pressed(self, instance): if instance.state=='down': Window.set_title("Kivy Full screen window") Window.maximize() elif instance.state=='normal': Window.set_title('Kivy Restored Window') Window.restore()
The bordered() method makes the window borderless when the b2 button is pressed, and back to original bordered window when released.
def bordered(self, instance): print (Window.top, Window.left) if instance.state=='down': Window.borderless=True elif instance.state=='normal': Window.borderless=False
The positioned() callback moves the window to (0,0) position and back to its earlier position when b3 is pressed/released.
def positioned(self, instance): print (Window.top, Window.left) if instance.state=='down': self.x, self.y=Window.left, Window.top Window.left, Window.top=(0,0) elif instance.state=='normal': Window.left, Window.top=self.x,self.y
The Application window appears as below in the first place. Generate the events (mouse leave, entered on request_close) and see the callbacks in action. Similarly check the action of togglebuttons.
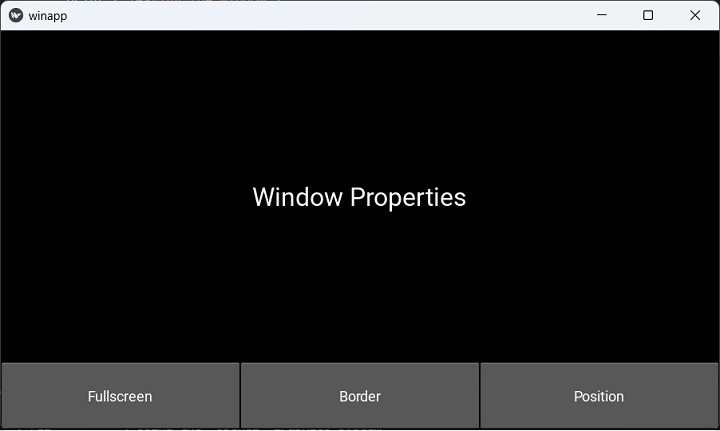
To Continue Learning Please Login