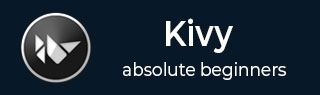
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Vector
In Euclidean geometry, a vector is an object that represents a physical quantity that has both the magnitude and direction. Kivy library includes Vector class and provides functionality to perform 2D vector operations.
The Vector class is defined in kivy.vector module. Kivy's Vector class inherits Python's builtin list class. A Vector object is instantiated by passing x and y coordinate values in a cartesian coordinate system.
from kivy.vector import Vector v=vector(10,10)
The two parameters are accessible either by the subscript operator. First parameter is v[0], and second parameter is v[1].
print (v[0], v[1])
They are also identified as x and y properties of a Vector object.
print (v.x, v.y)
You can also initialize a vector by passing a two value list or a tuple to the constructor.
vals = [10,10] v = Vector(vals)
Example
The Vector class in Kivy supports vector operations represented by the usual arithmetic operations +, −, /
The addition of two vectors (a,b)+(c,d) results in a vector (a+c, b+d). Similarly, "(a,b) - (c,d)" is equal to "(a − c, b − d)".
from kivy.vector import Vector a = (10, 10) b = (87, 34) print ("addition:",Vector(1, 1) + Vector(9, 5)) print ("Subtraction:",Vector(9, 5) - Vector(5, 5)) print ("Division:",Vector(10, 10) / Vector(2., 4.)) print ("division:",Vector(10, 10) / 5.)
Output
addition: [10, 6] Subtraction: [4, 0] Division: [5.0, 2.5] division: [2.0, 2.0]
Methods in Vector Class
Following methods are defined in Kivy's Vector class −
angle()
It computes the angle between a vector and an argument vector, and returns the angle in degrees.
Mathematically, the angle between vectors is calculated by the formula −
$$\theta =cos^{-1}\left [ \frac{x\cdot y}{\left| x\right|\left|y \right|} \right ]$$
The Kivy code to find angle is −
Example
a=Vector(100, 0) b=(0, 100) print ("angle:",a.angle(b))
Output
angle: -90.0
distance()
It returns the distance between two points. The Euclidean distance between two vectors is computed by the formula −
$$d\left ( p,q \right )=\sqrt{\left ( q_{1}-p_{1} \right )^{2}+\left ( q_{2}-p_{2} \right )^{2}}$$
The distance() method is easier to use.
Example
a = Vector(90, 33) b = Vector(76, 34) print ("Distance:",a.distance(b))
Output
Distance: 14.035668847618199
distance2()
It returns the distance between two points squared. The squared distance between two vectors x = [ x1, x2 ] and y = [ y1, y2 ] is the sum of squared differences in their coordinates.
Example
a = (10, 10) b = (5,10) print ("Squared distance:",Vector(a).distance2(b))
Output
Squared distance: 25
dot(a)
Computes the dot product of "a" and "b". The dot product (also called the scalar product) is the magnitude of vector b multiplied by the size of the projection of "a" onto "b". The size of the projection is $cos\theta $(where $\theta$ is the angle between the 2 vectors).
Example
print ("dot product:",Vector(2, 4).dot((2, 2)))
Output
dot product: 12
length()
It returns the length of a vector. length2() method Returns the length of a vector squared.
Example
pos = (10, 10) print ("length:",Vector(pos).length()) print ("length2:",Vector(pos).length2())
Output
length: 14.142135623730951 length2: 200
rotate(angle)
Rotate the vector with an angle in degrees.
Example
v = Vector(100, 0) print ("rotate:",v.rotate(45))
Output
rotate: [70.71067811865476, 70.71067811865476]
To Continue Learning Please Login