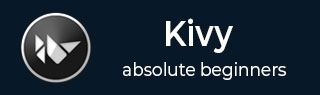
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Tree View
Most of the GUI toolkits, including Kivy, provide a TreeView widget with which a user can navigate and interact with hierarchical data presented as nodes in a tree-like format. The file and directory structure shown in the file explorer of an operating system is a typical example of TreeView.
The "kivy.uix.treeview" module includes the definitions of three important classes: TreeView, TreeViewNode, and TreeViewLabel. Objects of these classes constitute the tree view widget.
A TreeView is populated by the instances of TreeViewNode instances. Any widget from the library such as a label or a button, or a user-defined widget object is combined with TreeViewNode.
The root of the tree view is the TreeView object itself.
from kivy.uix.treeview import TreeView tv = TreeView()
One or more nodes can be directly added below this root. A TreeViewLabel is used as argument for add_node method
from kivy.uix.treeview import TreeViewLabel n1 = tv.add_node(TreeViewLabel(text='node 1'))
Instead of adding a node to the root of the tree, you can add it to a node itself. Provide the instance of the parent node as the second argument to the add_node() method −
n2 = tv.add_node(TreeViewLabel(text='Political Sci'), n1)
The root widget of the tree view is opened by default and its default caption is 'Root'. To change that, you can use the TreeView.root_options property. This will pass options to the root widget −
tv = TreeView(root_options=dict(text='My root'))
The TreeViewLabel itself is a label, and hence cannot generate any event as on_press. For that you should define a class that inherits TreeView and a Button.
class TreeViewButton(Button, TreeViewNode): pass
You can then process different events such as −
on_node_expand − Fired when a node is being expanded
on_node_collapse − Fired when a node is being collapsed
Example
The code given below composes a simple tree view of subjects available in each faculty of a college. A vertical box layout houses a treeview widget and a button. The root of the tree view is open.
To expand a node, click the ">" button to its left. It turns to a downward arrow. If clicked again, the node is collapsed.
from kivy.app import App from kivy.uix.treeview import TreeView, TreeViewLabel from kivy.uix.button import Button from kivy.uix.boxlayout import BoxLayout from kivy.core.window import Window Window.size = (720, 350) class DemoApp(App): def build(self): lo = BoxLayout(orientation='vertical') self.tv = TreeView(root_options={ 'text': 'Faculty-wise Subjects', 'font_size': 20} ) self.n1 = self.tv.add_node(TreeViewLabel(text='Arts')) self.n2 = self.tv.add_node(TreeViewLabel(text='Commerce')) self.n3 = self.tv.add_node(TreeViewLabel(text='Science')) self.n4 = self.tv.add_node( TreeViewLabel(text='Sociology'), self.n1 ) self.n5 = self.tv.add_node( TreeViewLabel(text='History'), self.n1 ) self.n6 = self.tv.add_node( TreeViewLabel(text='Political Sci'), self.n1 ) self.n7 = self.tv.add_node( TreeViewLabel(text='Accountancy'), self.n2 ) self.n8 = self.tv.add_node( TreeViewLabel(text='Secretarial Practice'), self.n2 ) self.n9 = self.tv.add_node( TreeViewLabel(text='Economics'), self.n2 ) self.n10 = self.tv.add_node( TreeViewLabel(text='Physics'), self.n3 ) self.n11 = self.tv.add_node( TreeViewLabel(text='Mathematics'), self.n3 ) self.n12 = self.tv.add_node( TreeViewLabel(text='Chemistry'), self.n3 ) lo.add_widget(self.tv) return lo DemoApp().run()
Output

To Continue Learning Please Login