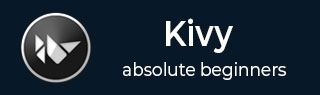
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Bubble
Kivy framework consists of a Bubble widget that acts as a small popup menu with an arrow on any one side of its contents. The direction of arrow can be configured as required. You can place it by setting its relative position of the "arrow_pos" property.
Contents of the bubble are placed in a "BubbleContent" object, a subclass of BoxLayout. One or more BubbleButtons can be placed either horizontally or vertically. Although it is recommended use BubbleButtons, you can add any widget in the bubble's content.

The classes Bubble, BubbleContent, and BubbleButton are defined in kivy.uix.bubble module.
from from kivy.uix.bubble import Bubble
The following properties of the Bubble class help in customizing the appearance and behaviour of the Bubble menu −
arrow_color − Arrow color, in the format (r, g, b, a). To use it you have to set arrow_image first, defaults to [1, 1, 1, 1].
arrow_image − Image of the arrow pointing to the bubble.
arrow_margin − Automatically computed margin that the arrow widget occupies in x and y direction in pixel.
arrow_pos − Specifies the position of the arrow as per one of the predefined values: left_top, left_mid, left_bottom top_left, top_mid, top_right right_top, right_mid, right_bottom bottom_left, bottom_mid, bottom_right. The default value is 'bottom_mid'.
content − This is the object where the main content of the bubble is held.
show_arrow − Indicates whether to show arrow. Default is True.
BubbleButton − A button intended for use in a BubbleContent widget. Instead of this, you can use a "normal" button, but it will may look good unless the background is changed.
BubbleContent − A styled BoxLayout that can be used as the content widget of a Bubble.
The following schematic "kv" language script is used to build a simple Bubble object −
Bubble: BubbleContent: BubbleButton: text: 'Button 1' BubbleButton: text: 'Button 2'
Just as in case of a normal Button, we can bind a BubbleButton to a callback for its "on_press" event.
Example
When the following code is executed, it presents a normal button. When clicked, a Bubble menu pops up with three BubbleButtons. Each of these BubbleButtons invoke a pressed() callback method that reads the button's caption and prints it on the console.
We use the following "kv" language script to assemble the Bubble menu. A class named as "Choices" has been defined that subclasses the "kivy.uix.bubble.Bubble" class.
class Choices(Bubble): def pressed(self, obj): print ("I like ", obj.text) self.clear_widgets()
The class has pressed() instance method, invoked by each BubbleButton.
Here is the "kv" script −
<Choices> size_hint: (None, None) size: (300, 150) pos_hint: {'center_x': .5, 'y': .6} canvas: Color: rgb: (1,0,0) Rectangle: pos:self.pos size:self.size BubbleContent: BubbleButton: text: 'Cricket' size_hint_y: 1 on_press:root.pressed(self) BubbleButton: text: 'Tennis' size_hint_y: 1 on_press:root.pressed(self) BubbleButton: text: 'Hockey' size_hint_y: 1 on_press:root.pressed(self)
BoxLayout widget acts as the root widget for the main application window and consists of a Button and a label. The "on_press" event invokes the "show_bubble()" method that pops up the bubble.
class BubbleTest(FloatLayout): def __init__(self, **temp): super(BubbleTestApp, self).__init__(**temp) self.bubble_button = Button( text ='Your favourite Sport', pos_hint={'center_x':.5, 'center_y':.5}, size_hint=(.3, .1),size=(300, 100) ) self.bubble_button.bind(on_release = self.show_bubble) self.add_widget(self.bubble_button) def show_bubble(self, *arg): self.obj_bub = Choices() self.add_widget(self.obj_bub)
The driver App class code is as below −
from kivy.app import App from kivy.uix.floatlayout import FloatLayout from kivy.uix.button import Button from kivy.uix.label import Label from kivy.uix.bubble import Bubble from kivy.properties import ObjectProperty from kivy.core.window import Window Window.size = (720,400) class MybubbleApp(App): def build(self): return BubbleTest() MybubbleApp().run()
Output
The application shows a button at the center of the main window. When clicked, you should see the bubble menu above it.
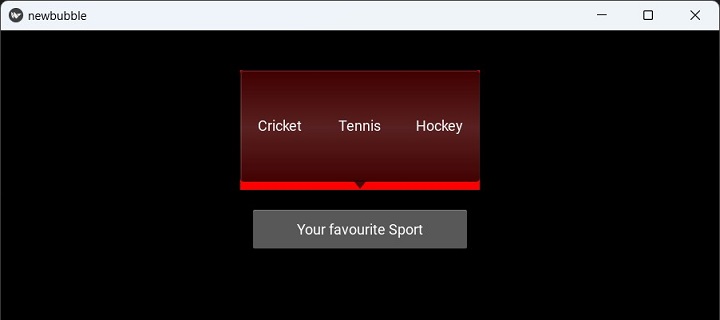
Every time you click any of the options in the bubble, the console shows the result, and hides the bubble.
I like Tennis I like Hockey
To Continue Learning Please Login