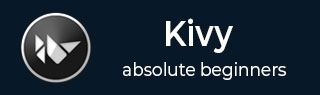
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Checkbox
In any GUI toolkit, a checkbox is used to enable the user to select one or choices from the available options. In Kivy, the CheckBox can be configured to make the choice mutually exclusive (only one of the available options is selectable), or let the user mark any number of choices.
If two or more checkboxes have the same value for group property, they appear as a circular radiobutton; user can choose only one option, as the active property of only one checkbox can be True, for others active property will be automatically False.
For the checkboxes not having the group property, it appears as a rectangular box which when pressed, shows a checkmark with active property becoming True. Click it again and the check marks is removed, the active property becomes False.
The CheckBox class is defined in kivy.uix.checkbox module
from kivy.uix.checkbox import CheckBox cb = CheckBox(**kwargs)
If the checkbox object is be bound to its active property, a callback can be invoked every time the active property changes.
checkbox = CheckBox() checkbox.bind(active=callback)
Example
The following Python code shows how to use checkboxes that are mutually exclusive, as well as multi-selectable.
The code uses a vertical BoxLayout with two horizontal layouts and two labels in it. The upper horizontal layout holds two checkboxes with group property of both as 'sex'
self.m = CheckBox(group='sex', color=[1,0,1,1]) self.m.bind(active=self.on_male) gendergrp.add_widget(self.m) gendergrp.add_widget(Label(text='Female')) self.f = CheckBox(active=False, group='sex') self.f.bind(active=self.on_female) gendergrp.add_widget(self.f)
Both the checkboxes invoke a callback method that identifies the active property.
The lower horizontal box holds three independent checkboxes −
interests.add_widget(Label(text='Sports')) self.cb1 = CheckBox() self.cb1.bind(active=self.on_interest) interests.add_widget(self.cb1) self.cb2 = CheckBox() self.cb2.bind(active=self.on_interest) interests.add_widget(Label(text='Music')) interests.add_widget(self.cb2) self.cb3 = CheckBox() self.cb3.bind(active=self.on_interest) interests.add_widget(Label(text='Travel')) interests.add_widget(self.cb3)
When each of these checkboxes are clicked, a list of selected interests is built and displayed on the label below the horizontal box.
Here is the complete code −
from kivy.app import App from kivy.uix.label import Label from kivy.uix.checkbox import CheckBox from kivy.uix.boxlayout import BoxLayout from kivy.core.window import Window Window.size = (720,400) class CheckBoxApp(App): gender='' intrst=[] def on_male(self, instance, value): if value: CheckBoxApp.gender='Male' self.lbl.text = "Gender selected: "+CheckBoxApp.gender else: self.lbl.text = "Gender selected: " def on_female(self, instance, value): if value: CheckBoxApp.gender='Female' self.lbl.text = "Gender selected: "+CheckBoxApp.gender else: self.lbl.text = "Gender selected: " def on_interest(self, instance, value): CheckBoxApp.intrst=[] if self.cb1.active: CheckBoxApp.intrst.append("Sports") if self.cb2.active: CheckBoxApp.intrst.append("Music") if self.cb3.active: CheckBoxApp.intrst.append("Travel") self.lbl1.text="Interests Selected: "+" ".join(CheckBoxApp.intrst) def build(self): main=BoxLayout(orientation='vertical') gendergrp=BoxLayout(orientation='horizontal') interests = BoxLayout(orientation='horizontal') gendergrp.add_widget(Label(text='Gender:')) gendergrp.add_widget(Label(text='Male')) self.m = CheckBox(group='sex', color=[1,0,1,1]) self.m.bind(active=self.on_male) gendergrp.add_widget(self.m) gendergrp.add_widget(Label(text='Female')) self.f = CheckBox(active=False, group='sex') self.f.bind(active=self.on_female) gendergrp.add_widget(self.f) main.add_widget(gendergrp) self.lbl = Label(text="Gender selected: ", font_size=32) main.add_widget(self.lbl) interests.add_widget(Label(text='Interests:')) interests.add_widget(Label(text='Sports')) self.cb1 = CheckBox() self.cb1.bind(active=self.on_interest) interests.add_widget(self.cb1) self.cb2 = CheckBox() self.cb2.bind(active=self.on_interest) interests.add_widget(Label(text='Music')) interests.add_widget(self.cb2) self.cb3 = CheckBox() self.cb3.bind(active=self.on_interest) interests.add_widget(Label(text='Travel')) interests.add_widget(self.cb3) self.lbl1 = Label(text="Interests selected: ", font_size=32) main.add_widget(interests) main.add_widget(self.lbl1) return main if __name__ == '__main__': CheckBoxApp().run()
Output
When you run this code, it will produce a GUI like the one shown here −
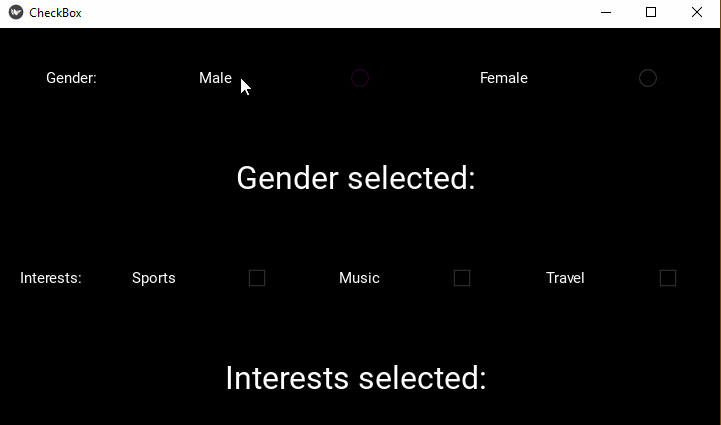
To Continue Learning Please Login