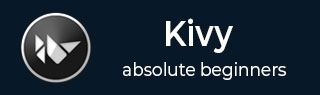
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Effects
The Kivy library provides the "kivy.effects" subpackage to control the overscroll effects when using the ScrollView widget in a Kivy app. The Effect classes can perform actions like bouncing back, changing opacity, or prevent scrolling beyond the normal boundaries.
There are three Effect classes −
ScrollEffect − Base class used for implementing an effect. It only calculates the scrolling and the overscroll. This class is defined in kivy.effects.scroll module
DampedScrollEffect − Uses the overscroll information to allow the user to drag more than expected. Once the user stops the drag, the position is returned to one of the bounds. The definition of this class is in kivy.effects.dampedscroll module.
OpacityScrollEffect − Uses the overscroll information to reduce the opacity of the scrollview widget. When the user stops the drag, the opacity is set back to 1. The class definition is available in kivy.effects.opacityscroll module.
These classes use the KineticEffect as the base class for computing velocity out of a movement.
To apply the effect of any of these classes on the scrolling behavior of ScrollView, set one of these classes as the value of effect_cls property of ScrollView widget.
scr = ScrollView(size=Window.size) scr.eefect_cls=ScrollEffect
Example
The following "kv" language script constructs a ScrollView with a hundred buttons added to a GridLayout. The "effect_cls" property is set to ScrollEffect class.
#:import ScrollEffect kivy.effects.scroll.ScrollEffect #:import Button kivy.uix.button.Button <RootWidget> effect_cls: ScrollEffect GridLayout: size_hint_y: None height: self.minimum_height cols: 1 on_parent: for i in range(100): self.add_widget(Button(text=str(i), size_hint_y=None))
The above "kv" code uses a class rule by the name RootWidget. The build() method of the App class in the following Python code returns an object of RootWidget class.
from kivy.uix.gridlayout import GridLayout from kivy.uix.button import Button from kivy.uix.scrollview import ScrollView from kivy.effects.dampedscroll import DampedScrollEffect from kivy.core.window import Window from kivy.app import App from kivy.core.window import Window Window.size = (720,350) class RootWidget(ScrollView): pass class scrollableapp(App): def build(self): return RootWidget() scrollableapp().run()
Output
Execute the above Python program from command line. You get the app window with a scrollview, showing a snapshot of buttons. You can scroll up or down with the ScrollEffect activated.
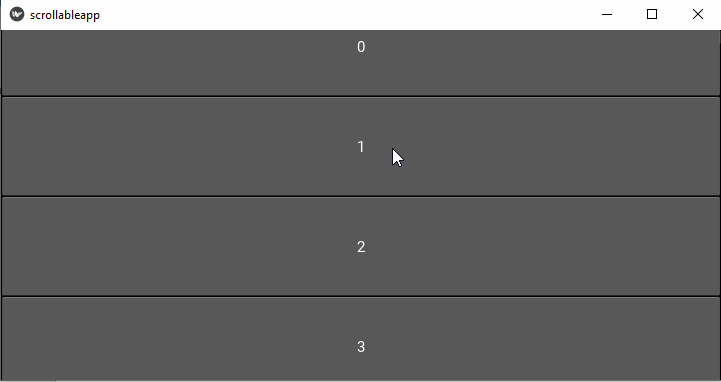
You can customize the Effect class by specifying the attributes in the RootWidget class and use it as the "effect_cls" property.
For example, you can set the max and min boundaries to be used for scrolling. The overscroll property is the computed value when the user overscrolls, i.e., goes out of the bounds.
To Continue Learning Please Login