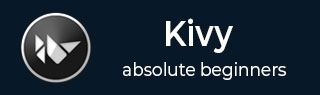
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Camera
With the Camera widget in Kivy, it is possible to display the video stream from a camera device. Kivy may take some time to initialize the camera device, and update the widget texture afterwards.
The Camera class is defined in the "kivy.uix.camera: module.
from kivy.uix.camera import Camera cam = Camera(**kwargs)
If the system finds multiple camera devices, you need to specify the camera to be used by its index.
cam = Camera(index=1)
You can also specify the camera resolution with the resolution argument −
cam = Camera(index=1, resolution=(640, 480))
The kivy.uix.camera.Camera class is a concrete implementation of the core Camera class from the "kivy.core.camera" module, and performs the initialization and frame capture functions.
Kivy needs to find an appropriate camera provider to be able to detect the hardware. For this purpose, install the latest version of opencv-python package, which also installs its dependency packages including NumPy.
pip install opencv-python
To start streaming the feed from your camera on the app window, set the play property of the Camera object to True, and set it to False to stop the feed.
cam.play = True
To capture the snapshot of the camera stream to an image, use the export_to_png() method. Specify the filename to save to.
The Camera class defines following attributes −
index − Index of the used camera, starting from 0. Setting it to -1 to allow auto selection.
play − Boolean indicating whether the camera is playing or not. You can start/stop the camera by setting this property −
# create the camera, and start later (default) cam = Camera() # and later cam.play = True # to sop cam.play = False
resolution − Preferred resolution to use when invoking the camera. If you are using [-1, -1], the resolution will be the default one. To set the desired resolution, provided it is supported by the device −
cam = Camera(resolution=(640, 480))
Example
The following example code adds a Camera widget and a ToggleButton inside a vertical BoxLayout. The callback bound to the toggle button sets the camera object's play property to True when the button is down, and otherwise the video is stopped.
from kivy.app import App from kivy.uix.boxlayout import BoxLayout from kivy.uix.togglebutton import ToggleButton from kivy.uix.camera import Camera from kivy.core.window import Window Window.size = (720,350) class TestCameraApp(App): def build(self): box=BoxLayout(orientation='vertical') self.mycam=Camera(play=False, resolution= (640, 480)) box.add_widget(self.mycam) tb=ToggleButton(text='Play', size_hint_y= None, height= '48dp') tb.bind(on_press=self.play) box.add_widget(tb) return box def play(self, instance): if instance.state=='down': self.mycam.play=True instance.text='Stop' else: self.mycam.play=False instance.text='Play' TestCameraApp().run()
Output
Run the code and check the output −
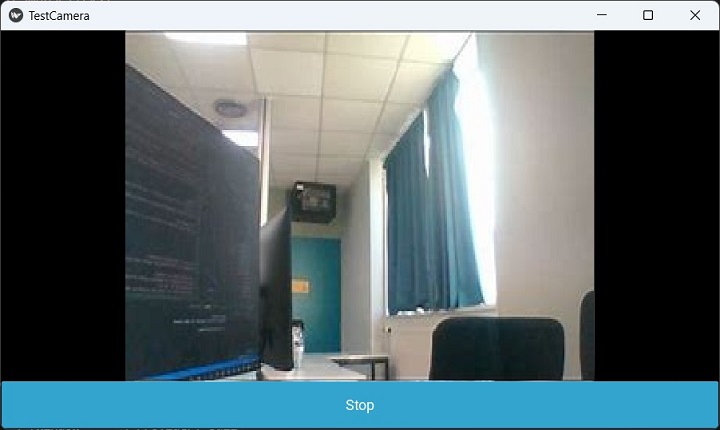
You can also use the "kv" language script to design the application window layout. Save the following script as "TestCamera.kv", comment out the code in the build() method, and just place a "pass" statement in it.
BoxLayout: orientation: 'vertical' Camera: id: camera resolution: (640, 480) play: False ToggleButton: text: 'Play' on_press: camera.play = not camera.play size_hint_y: None height: '48dp'
To Continue Learning Please Login