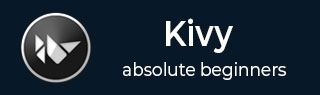
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Text Input
You often see a rectangular box being used in desktop and web applications, meant for the user to enter some text. A textbox is an essential widget in any GUI toolkit. In Kivy, the TextInput provides a control in which the user can enter and edit text.
The TextInput control can be customized to receive a single line or multiline text. A certain part of the text can be selected with mouse. One can also perform full screen editing inside it with the cursor movement.
The TextInput class is defined in kivy.uix.textinput module.
from kivy.uix.textinput import TextInput textbox = TextInput(**kwargs)
Following properties are defined in the TextInput class −
allow_copy − Decides whether to allow copying the text. allow_copy is a BooleanProperty and defaults to True.
background_color − Current color of the background, in (r, g, b, a) format.It is a ColorProperty and defaults to [1, 1, 1, 1] (white).
border − Border used for BorderImage graphics instruction. Used with background_normal and background_active. Can be used for a custom background. It must be a list of four values: (bottom, right, top, left). border is a ListProperty and defaults to (4, 4, 4, 4).
cursor − Tuple of (col, row) values indicating the current cursor position. You can set a new (col, row) if you want to move the cursor. The scrolling area will be automatically updated to ensure that the cursor is visible inside the viewport. cursor is an AliasProperty.
cursor_color − Current color of the cursor, in (r, g, b, a) format. cursor_color is a ColorProperty and defaults to [1, 0, 0, 1].
cut() − Copy current selection to clipboard then delete it from TextInput.
delete_selection(from_undo=False) − Delete the current text selection (if any).
disabled_foreground_color − Current color of the foreground when disabled, in (r, g, b, a) format. disabled_foreground_color is a ColorProperty and defaults to [0, 0, 0, 5] (50% transparent black).
font_name − Filename of the font to use. The path can be absolute or relative. Relative paths are resolved by the resource_find() function.
font_name − is a StringProperty and defaults to 'Roboto'. This value is taken from Config.
font_size − Font size of the text in pixels. font_size is a NumericProperty and defaults to 15 sp.
foreground_color − Current color of the foreground, in (r, g, b, a) format. oreground_color is a ColorProperty and defaults to [0, 0, 0, 1] (black).
halign − Horizontal alignment of the text. halign is an OptionProperty and defaults to 'auto'. Available options are : auto, left, center and right.
hint_text − Hint text of the widget, shown if text is ''. hint_text a AliasProperty and defaults to ''.
hint_text_color − Current color of the hint_text text, in (r, g, b, a) format, ColorProperty and defaults to [0.5, 0.5, 0.5, 1.0] (grey).
input_filter − Filters the input according to the specified mode, if not None. If None, no filtering is applied. It is an ObjectProperty and defaults to None. Can be one of None, 'int' (string), or 'float' (string), or a callable.
insert_text(substring, from_undo=False) − Insert new text at the current cursor position. Override this function in order to pre-process text for input validation.
line_height − Height of a line. This property is automatically computed from the font_name, font_size. Changing the line_height will have no impact. line_height is a NumericProperty, read-only.
line_spacing − Space taken up between the lines. line_spacing is a NumericProperty and defaults to 0.
minimum_height − Minimum height of the content inside the TextInput. minimum_height is a readonly AliasProperty.
multiline − If True, the widget will be able show multiple lines of text. If False, the "enter" keypress will defocus the textinput instead of adding a new line
on_touch_down(touch) − Receive a touch down event. The touch parameter is object of MotionEvent class. It returns bool If True, the dispatching of the touch event will stop. If False, the event will continue to be dispatched to the rest of the widget tree.
on_touch_move(touch) − Receive a touch move event. The touch is in parent coordinates.
on_touch_up(touch) − Receive a touch up event. The touch is in parent coordinates.
padding − Padding of the text: [padding_left, padding_top, padding_right, padding_bottom]. Padding also accepts a two argument form [padding_horizontal, padding_vertical] and a one argument form [padding]. Padding is a VariableListProperty and defaults to [6, 6, 6, 6].
password − If True, the widget will display its characters as the character set in password_mask.
password_mask − Sets the character used to mask the text when password is True. password_mask is a StringProperty and defaults to '*'.
paste() − Insert text from system Clipboard into the TextInput at current cursor position.
readonly − If True, the user will not be able to change the content of a textinput.
select_all() − Select all of the text displayed in this TextInput.
select_text(start, end) − Select a portion of text displayed in this TextInput. Parameters are start - Index of textinput.text from where to start selection and end - Index of textinput.text till which the selection should be displayed
selection_color − Current color of the selection, in (r, g, b, a) format.
selection_from − If a selection is in progress or complete, this property will represent the cursor index where the selection started.
selection_text − Current content selection. selection_text is a StringProperty and defaults to '', readonly.
tab_width − By default, each tab will be replaced by four spaces on the text input widget. You can set a lower or higher value. tab_width is a NumericProperty and defaults to 4.
text − Text of the widget. It is an AliasProperty.
Usage
To create a simple hello world −
widget = TextInput(text='Hello world')
If you want to create the widget with an unicode string, use −
widget = TextInput(text=u'My unicode string')
When the user enters data inside the TextInput widget, it becomes the value of text property.
You can invoke a callback when the text property of TextInput object changes.
def callback(instance, value): print('The widget', instance, 'have:', value) textinput = TextInput() textinput.bind(text=callback)
When the multiline property is False, the TextInput accepts a single line input. When the user presses Enter, an on_text_validate event is generated −
def callback(instance, value): print('The widget', instance, 'have:', value) textinput = TextInput(multiline=False) textinput.bind(on_text_validate=callback)
Example
Let us use some of the properties and methods of TextInput class explained above. In the following example, we have two multiline textboxes and two buttons arranged in a BoxLayout.
The COPY button calls gettext() method which stores the selected text from the upper text box.
def gettext(self, instance): mydemoapp.text = self.text1.selection_text
The PASTE button invokes a callback insert() which pastes the selected text at the cursor position.
def insert(self, instance): self.text2.insert_text(mydemoapp.text)
These two functions are bound to two buttons −
self.b1=Button(text='COPY') self.b1.bind(on_press=self.gettext) self.b2=Button(text='PASTE') self.b2.bind(on_press=self.insert)
The build() method assembles the text boxes and buttons.
Here is the complete code −
from kivy.app import App from kivy.uix.boxlayout import BoxLayout from kivy.uix.textinput import TextInput from kivy.uix.button import Button from kivy.config import Config Config.set('graphics', 'width', '720') Config.set('graphics', 'height', '300') Config.set('graphics', 'resizable', '1') class mydemoapp(App): text='' def gettext(self, instance): mydemoapp.text = self.text1.selection_text def insert(self, instance): self.text2.insert_text(mydemoapp.text) def build(self): main= BoxLayout(orientation= 'vertical') self.text1 = TextInput(multiline=True, font_size=20) btns = BoxLayout(orientation='horizontal') self.b1=Button(text='COPY') self.b1.bind(on_press=self.gettext) self.b2=Button(text='PASTE') self.b2.bind(on_press=self.insert) self.text2 = TextInput( multiline=True, font_size=20, foreground_color=[0,0,1,1] ) btns.add_widget(self.b1) btns.add_widget(self.b2) main.add_widget(self.text1) main.add_widget(btns) main.add_widget(self.text2) return main mydemoapp().run()
Output
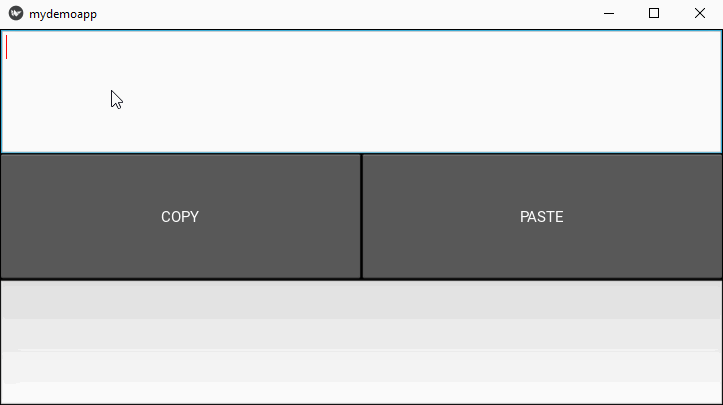
To Continue Learning Please Login