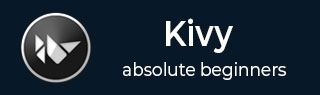
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - ScrollView
The ScrollView widget in Kivy framework encloses any other widget having dimensions larger than the size allocated to it, and provides a scrollable panel to it. This enables the enclosed widget to be panned/scrolled vertically or horizontally.
The ScrollView class is defined in kivy.uix.scrollview module. You normally compose one or more widgets in a layout and add the layout to the ScrollView.
from kivy.uix.scrollview import ScrollView view = ScrollView() view.add_widget(layout)
The "scroll_x" and "scroll_y" properties of a ScrollView object control the scrolling behavior of the scroll panel. The ScollView enables scrolling in both directions. You can disable on an axis by setting "do_scroll_x" or "do_scroll_y" to False.
Further, the "scroll_distance" property sets the minimum distance to travel, defaults to 20 pixels. Additionally, the scroll_timeout property specifies the maximum time period which is by default 55 milliseconds.
The significance of "scroll_distance" and "scroll_timeout" is this: If the number of pixels scrolled by the touch gesture is more than or equal to scroll_distance, and it is within the scroll_timeout period, it is recognized as a scrolling gesture and translation (scroll/pan) will begin. If the timeout occurs, the touch down event is propagated to the child instead.
Other properties of ScrollView class are given below −
do_scroll − Allow scroll on X or Y axis.
do_scroll_x − Allow scroll on X axis. This is a BooleanProperty and defaults to True.
do_scroll_y − Allow scroll on Y axis. This is a BooleanProperty and defaults to True.
scroll_distance − Distance to move before scrolling the ScrollView, in pixels. It is a NumericProperty and defaults to 20 pixels.
scroll_timeout − Timeout allowed to trigger the scroll_distance, in milliseconds, default being 55 ms
scroll_to() − Scrolls the viewport to ensure that the given widget is visible, optionally with padding and animation.
scroll_type − Sets the type of scrolling to use for the content of the scrollview. Available options are: ['content'], ['bars'], ['bars', 'content'].
The ScrollView object emits following events −
on_scroll_start − Generic event fired when scrolling starts from touch.
on_scroll_move − Generic event fired when scrolling move from touch.
on_scroll_stop − Generic event fired when scrolling stops from touch.
Example
To be able to understand the working of ScrollView, we need a layout large enough, so that it overflows the dimensions of the main application window. For this purpose, we add 1oo buttons to a one-column gridlayout, and apply scrollview to it.
The operative code in the following example is given below −
layout = GridLayout(cols=1) for i in range(100): btn = Button(text='Button ' + str(i), size_hint_y=None, height=40) layout.add_widget(btn) root = ScrollView() root.add_widget(layout)
To make sure the height is such that there is something to scroll, bind the layout object's minimum_height property to its setter.
layout.bind(minimum_height=layout.setter('height'))
Here is the complete code for ScrollView demo code −
from kivy.uix.gridlayout import GridLayout from kivy.uix.button import Button from kivy.uix.scrollview import ScrollView from kivy.app import App from kivy.core.window import Window Window.size = (720, 350) class scrollableapp(App): def build(self): layout = GridLayout(cols=1, spacing=10, size_hint_y=None) layout.bind(minimum_height=layout.setter('height')) for i in range(100): btn = Button(text='Button ' + str(i), size_hint_y=None, height=40) layout.add_widget(btn) root = ScrollView( size_hint=(1, None), size=(Window.width, Window.height) ) root.add_widget(layout) return root scrollableapp().run()
Output
Run the above code. To go beyond the buttons visible in the view, scroll vertically with mouse, or fingers if you are working on a touch-enabled device.
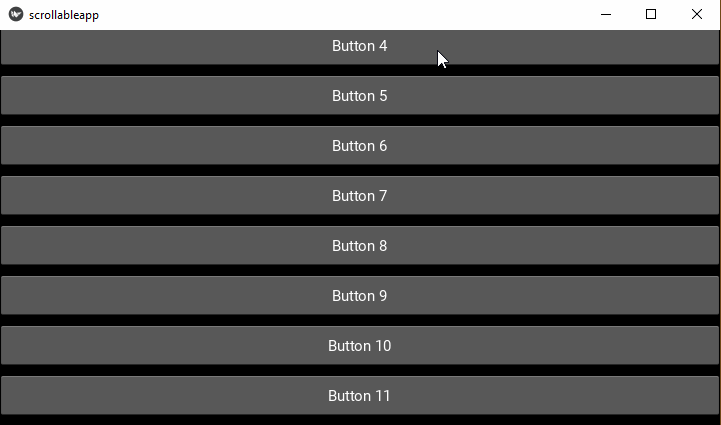
To Continue Learning Please Login