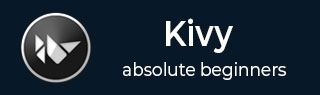
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Spinner
The Spinner control in Kivy framework is a more conventional type of dropdown control, different from Kivy's DropDown widget. Compared to DropDown, it is easier and convenient to construct and use the Spinner widget.
The main difference between Kivy's dropdown and spinner widgets is that the dropdown widget may consist of any other Kivy widget such as Label, Button, Image etc; while a spinner is simply a list of strings.
The Spinner class is defined in the "kivy.uix.spinner" module
from kivy.uix.spinner import Spinner spin = Spinner(**kwargs)
A Spinner widget shows a text caption corresponding to the currently selected value. The Spinner object can be constructed with different properties as keyword arguments. However, these two properties are important −
The text property is a string and shows the default value.
The values property is a ListProperty, consisting of all the values to choose from.
To construct a simple spinner, use the following code snippet −
from kivy.base import runTouchApp from kivy.uix.spinner import Spinner spinner = Spinner( text='English', values=('English', 'French', 'German', 'Chinese') )
The spinner object's text property can be bound to a callback to invoke appropriate action whenever the selection is made.
def value_changed(spinner, text): print(You selected', text, 'language') spinner.bind(text=show_selected_value)
Other properties in the Spinner class are listed below −
dropdown_cls − A class used to display the dropdown list when the Spinner is pressed. It is an ObjectProperty and defaults to DropDown.
is_open − By default, the spinner is not open. Set to True to open it.
option_cls − A class used to display the options within the dropdown list displayed under the Spinner. The text property of the class will be used to represent the value. Its on_release event is used to trigger the option when pressed/touched.
text_autoupdate − This is BooleanProperty. It indicates if the spinner's text should be automatically updated with the first value of the values property. Setting it to True will cause the spinner to update its text property every time values are changed.
values − Values that can be selected by the user. It must be a list of strings. It is a ListProperty and defaults to [].
The code below assembles a Spinner, associated with a label to show the selected value in the horizontal box. The lower horizontal box has a TextInput and a Button. The intention is to provide a callback on this button that adds the string in the textbox to the Spinner values
The program has two callback methods in the App class. One to display the selected value from the spinner, and another to add a new language to the spinner.
The callback to add a new language −
def addvalue(self, instance): self.spin1.values.append(self.t1.text)
We shall bind this method to the "Add" button.
To display the selected language on a label −
def on_spinner_select(self, spinner, text): self.spinnerSelection.text = "Selected Language is: %s" %self.spin1.text
We shall bind this method to the "text" property of the Spinner widget.
Example
Save and run the code below as "spiinerdemo.py"
from kivy.app import App from kivy.uix.label import Label from kivy.uix.spinner import Spinner from kivy.uix.boxlayout import BoxLayout from kivy.uix.textinput import TextInput from kivy.uix.button import Button from kivy.core.window import Window Window.size = (720, 400) class SpinnerExample(App): def addvalue(self, instance): self.spin1.values.append(self.t1.text) def build(self): layout = BoxLayout(orientation='vertical') lo1 = BoxLayout(orientation='horizontal') self.spin1 = Spinner( text="Python", values=("Python", "Java", "C++", "C", "C#", "PHP"), background_color=(0.784, 0.443, 0.216, 1), size_hint=(.5, .4), pos_hint={'top': 1} ) lo1.add_widget(self.spin1) self.spinnerSelection = Label( text="Selected value in spinner is: %s" % self.spin1.text, pos_hint={'top': 1, 'x': .4} ) lo1.add_widget(self.spinnerSelection) layout.add_widget(lo1) lo2 = BoxLayout(orientation='horizontal') lo2.add_widget(Label(text="Add Language")) self.t1 = TextInput() self.b1 = Button(text='add') lo2.add_widget(self.t1) lo2.add_widget(self.b1) layout.add_widget(lo2) self.spin1.bind(text=self.on_spinner_select) self.b1.bind(on_press=self.addvalue) return layout def on_spinner_select(self, spinner, text): self.spinnerSelection.text = "Selected value in spinner is: %s" % self.spin1.text print('The spinner', spinner, 'have text', text) if __name__ == '__main__': SpinnerExample().run()
Output
The button on the top-left is the Spinner. When clicked, the list of languages drops down. You can make a selection. The selected name will be displayed on the label to its right.
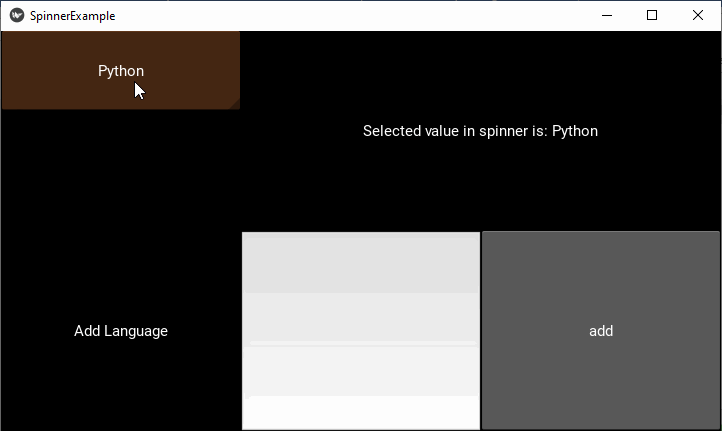
To add a new language to the list, type in the text box, and click the "Add" button. The spinner widget will be appended with the new language name at the bottom. If the list is long enough, you can scroll down with mouse.
To Continue Learning Please Login