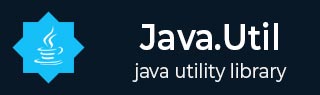
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Arrays Class
Introduction
The Java Arrays class contains a static factory that allows arrays to be viewed as lists. Following are the important points about Arrays −
This class contains various methods for manipulating arrays (such as sorting and searching).
The methods in this class throw a NullPointerException if the specified array reference is null.
Arrays Class Declaration
Following is the declaration for java.util.Arrays class −
public class Arrays extends Object
Arrays Class Methods
Sr.No. |
Method & Description |
---|---|
1 |
static <T> List<T> asList(T... a) This method returns a fixed-size list backed by the specified array. |
2 |
static int binarySearch(byte[] a, byte key) This method searches the specified array of bytes for the specified value using the binary search algorithm. |
3 |
static int binarySearch(char[] a, char key) This method searches the specified array of chars for the specified value using the binary search algorithm. |
4 |
static int binarySearch(double[] a, double key) This method searches the specified array of doubles for the specified value using the binary search algorithm. |
5 |
static int binarySearch(float[] a, float key) This method searches the specified array of floats for the specified value using the binary search algorithm. |
6 |
static int binarySearch(int[] a, int key) This method searches the specified array of ints for the specified value using the binary search algorithm. |
7 |
static int binarySearch(long[] a, int fromIndex, int toIndex, long key) This method searches a range of the specified array of longs for the specified value using the binary search algorithm. |
8 |
static int binarySearch(Object[] a, Object key) This method searches the specified array for the specified object using the binary search algorithm. |
9 |
static int binarySearch(short[] a, short key) This method searches the specified array of shorts for the specified value using the binary search algorithm. |
10 |
static <T> int binarySearch(T[] a, T key, Comparator<? super T> c) This method searches the specified array for the specified object using the binary search algorithm. |
11 |
static int compare(boolean[] a, boolean[] b) This method compares two boolean arrays lexicographically. |
12 |
static int compare(byte[] a, byte[] b) This method compares two byte arrays lexicographically. |
13 |
static int compare(char[] a, char[] b) This method compares two char arrays lexicographically. |
14 |
static int compare(double[] a, double[] b) This method compares two double arrays lexicographically. |
15 |
static int compare(float[] a, float[] b) This method compares two float arrays lexicographically. |
16 |
static int compare(int[] a, int[] b) This method compares two int arrays lexicographically. |
17 |
static int compare(long[] a, long[] b) This method compares two long arrays lexicographically. |
18 |
static int compare(short[] a, short[] b) This method compares two short arrays lexicographically. |
19 |
compareUnsigned(byte[] a, byte[] b) This method compares two byte arrays lexicographically, numerically treating elements as unsigned. |
20 |
compareUnsigned(int[] a, int[] b) This method compares two int arrays lexicographically, numerically treating elements as unsigned. |
21 |
compareUnsigned(long[] a, long[] b) This method compares two long arrays lexicographically, numerically treating elements as unsigned. |
22 |
compareUnsigned(short[] a, short[] b) This method compares two short arrays lexicographically, numerically treating elements as unsigned. |
23 |
static boolean[] copyOf(boolean[] original, int newLength) This method copies the specified array, truncating or padding with false (if necessary) so the copy has the specified length. |
24 |
static byte[] copyOf(byte[] original, int newLength) This method copies the specified array, truncating or padding with false (if necessary) so the copy has the specified length. |
25 |
static char[] copyOf(char[] original, int newLength) This method copies the specified array, truncating or padding with false (if necessary) so the copy has the specified length. |
26 |
static double[] copyOf(double[] original, int newLength) This method copies the specified array, truncating or padding with false (if necessary) so the copy has the specified length. |
27 |
static float[] copyOf(float[] original, int newLength) This method copies the specified array, truncating or padding with zeros (if necessary) so the copy has the specified length. |
28 |
static int[] copyOf(int[] original, int newLength) This method copies the specified array, truncating or padding with zeros (if necessary) so the copy has the specified length. |
29 |
static long[] copyOf(long[] original, int newLength) This method copies the specified array, truncating or padding with zeros (if necessary) so the copy has the specified length. |
30 |
static short[] copyOf(short[] original, int newLength) This method copies the specified array, truncating or padding with zeros (if necessary) so the copy has the specified length. |
31 |
static <T> T[] copyOf(T[] original, int newLength) This method copies the specified array, truncating or padding with nulls (if necessary) so the copy has the specified length. |
32 |
static <T, U> T[] copyOf(U[] original, int newLength,Class<? extends T[]> newType) This method copies the specified array, truncating or padding with nulls (if necessary) so the copy has the specified length. |
33 |
static boolean[] copyOfRange(boolean[] original, int from, int to) This method copies the specified range of the specified array into a new array. |
34 |
static byte[] copyOfRange(byte[] original, int from, int to) This method copies the specified range of the specified array into a new array. |
35 |
static char[] copyOfRange(char[] original, int from, int to) This method copies the specified range of the specified array into a new array. |
36 |
static double[] copyOfRange(double[] original, int from, int to) This method copies the specified range of the specified array into a new array. |
37 |
static int[] copyOfRange(int[] original, int from, int to) This method copies the specified range of the specified array into a new array. |
38 |
static long[] copyOfRange(long[] original, int from, int to) This method copies the specified range of the specified array into a new array. |
39 |
static short[] copyOfRange(short[] original, int from, int to) This method copies the specified range of the specified array into a new array. |
40 |
static <T> T[] copyOfRange(T[] original, int from, int to) This method copies the specified range of the specified array into a new array. |
41 |
static <T,U> T[] copyOfRange(U[] original, int from, int to, Class<? extends T[]> newType) This method copies the specified range of the specified array into a new array. |
42 |
static boolean deepEquals(Object[] a1, Object[] a2) This method returns true if the two specified arrays are deeply equal to one another. |
43 |
static int deepHashCode(Object[] a) This method returns a hash code based on the "deep contents" of the specified array. |
44 |
static String deepToString(Object[] a) This method returns a string representation of the "deep contents" of the specified array. |
45 |
static boolean equals(boolean[] a, boolean[] a2) This method returns true if the two specified arrays of booleans are equal to one another. |
46 |
static boolean equals(byte[] a, byte[] a2) This method returns true if the two specified arrays of bytes are equal to one another. |
47 |
static boolean equals(char[] a, char[] a2) This method returns true if the two specified arrays of chars are equal to one another. |
48 |
static boolean equals(double[] a, double[] a2) This method returns true if the two specified arrays of double are equal to one another. |
49 |
static boolean equals(float[] a, float[] a2) This method returns true if the two specified arrays of floats are equal to one another. |
50 |
static boolean equals(int[] a, int[] a2) This method returns true if the two specified arrays of ints are equal to one another. |
51 |
static boolean equals(long[] a, long[] a2) This method returns true if the two specified arrays of longs are equal to one another. |
52 |
static boolean equals(short[] a, short[] a2) This method returns true if the two specified arrays of shorts are equal to one another. |
53 |
static boolean equals(Object[] a, Object[] a2) This method returns true if the two specified arrays of Objects are equal to one another. |
54 |
static boolean equals(T[] a, T[] a2, Comparator<? super T> cmp) This method returns true if the two specified arrays of Objects are equal to one another. |
55 |
static void fill(boolean[] a, boolean val) This method assigns the specified boolean value to each element of the specified array of booleans. |
56 |
static void fill(byte[] a, byte val) This method assigns the specified byte value to each element of the specified array of bytes. |
57 |
static void fill(char[] a, char val) This method assigns the specified char value to each element of the specified array of chars. |
58 |
static void fill(double[] a, double val) This method assigns the specified double value to each element of the specified array of doubles. |
59 |
static void fill(float[] a, float val) This method assigns the specified float value to each element of the specified array of floats. |
60 |
static void fill(int[] a, int val) This method assigns the specified int value to each element of the specified array of ints. |
61 |
static void fill(long[] a, long val) This method assigns the specified long value to each element of the specified array of longs. |
62 |
static void fill(Object[] a, Object val) This method assigns the specified Object reference to each element of the specified array of Objects. |
63 |
static void fill(short[] a, short val) This method assigns the specified short value to each element of the specified array of shorts. |
64 |
static int hashCode(boolean[] a) This method returns a hash code based on the contents of the specified array. |
65 |
static int mismatch(boolean[] a, boolean[] b) This method finds and returns the index of the first mismatch between two boolean arrays, otherwise return -1 if no mismatch is found. |
66 |
static int mismatch(byte[] a, byte[] b) This method finds and returns the index of the first mismatch between two byte arrays, otherwise return -1 if no mismatch is found. |
67 |
static int mismatch(char[] a, char[] b) This method finds and returns the index of the first mismatch between two char arrays, otherwise return -1 if no mismatch is found. |
68 |
static int mismatch(double[] a, double[] b) This method finds and returns the index of the first mismatch between two double arrays, otherwise return -1 if no mismatch is found. |
69 |
static int mismatch(int[] a, int[] b) This method finds and returns the index of the first mismatch between two int arrays, otherwise return -1 if no mismatch is found. |
70 |
static int mismatch(long[] a, long[] b) This method finds and returns the index of the first mismatch between two long arrays, otherwise return -1 if no mismatch is found. |
71 |
static int mismatch(short[] a, short[] b) This method finds and returns the index of the first mismatch between two short arrays, otherwise return -1 if no mismatch is found. |
72 |
static int mismatch(Object[] a, Object[] b) This method finds and returns the index of the first mismatch between two object arrays, otherwise return -1 if no mismatch is found. |
73 |
static int mismatch(T[] a, T[] b, Comparator<? super T> cmp) This method finds and returns the index of the first mismatch between two object arrays, otherwise return -1 if no mismatch is found. |
74 |
static void parallelPrefix(double[] array, DoubleBinaryOperator op) This method cumulates, in parallel, each element of the given array in place, using the supplied function. |
75 |
static void parallelPrefix(int[] array, IntBinaryOperator op) This method cumulates, in parallel, each element of the given array in place, using the supplied function. |
76 |
static void parallelPrefix(long[] array, LongBinaryOperator op) This method cumulates, in parallel, each element of the given array in place, using the supplied function. |
77 |
static <T> void parallelPrefix(T[] array, BinaryOperator<T> op) This method cumulates, in parallel, each element of the given array in place, using the supplied function. |
78 |
static void parallelSetAll(double[] array, IntToDoubleFunction generator) This method sets all elements of the specified array, in parallel, using the provided generator function to compute each element. |
79 |
static void parallelSetAll(int[] array, IntUnaryOperator generator) This method sets all elements of the specified array, in parallel, using the provided generator function to compute each element. |
80 |
static void parallelSetAll(long[] array, IntToLongFunction generator) This method sets all elements of the specified array, in parallel, using the provided generator function to compute each element. |
81 |
static <T> void parallelSetAll(T[] array, IntFunction<? extends T> generator) This method sets all elements of the specified array, in parallel, using the provided generator function to compute each element. |
82 |
static void parallelSort(byte[] a) This method sorts the specified array of bytes into ascending numerical order. |
83 |
static void parallelSort(char[] a) This method sorts the specified array of chars into ascending numerical order. |
84 |
static void parallelSort(double[] a) This method sorts the specified array of doubles into ascending numerical order. |
85 |
static void parallelSort(float[] a) This method sorts the specified array of floats into ascending numerical order. |
86 |
static void parallelSort(int[] a) This method sorts the specified array of ints into ascending numerical order. |
87 |
static void parallelSort(long[] a) This method sorts the specified array of longs into ascending numerical order. |
88 |
static void parallelSort(short[] a) This method sorts the specified array of shorts into ascending numerical order. |
89 |
static <T extends Comparable<? super T>> void parallelSort(T[] a) This method sorts the specified array of objects into ascending order, according to the natural ordering of its elements. |
90 |
static <T>> void parallelSort(T[] a, Comparator<? super T> cmp) This method sorts the specified array of objects into ascending order, according to the ordering provided by the Comparator instance. |
91 |
static void setAll(double[] array, IntToDoubleFunction generator) This method sets all elements of the specified array, using the provided generator function to compute each element. |
92 |
static void setAll(int[] array, IntUnaryOperator generator) This method sets all elements of the specified array, using the provided generator function to compute each element. |
93 |
static void setAll(long[] array, IntToLongFunction generator) This method sets all elements of the specified array, using the provided generator function to compute each element. |
94 |
static <T> void setAll(T[] array, IntFunction<? extends T> generator) This method sets all elements of the specified array, using the provided generator function to compute each element. |
95 |
static Spliterator.OfDouble spliterator(double[] array) This method returns a Spliterator.OfDouble covering all of the specified array. |
96 |
static Spliterator.OfInt spliterator(int[] array) This method returns a Spliterator.OfInt covering all of the specified array. |
97 |
static Spliterator.OfLong spliterator(long[] array) This method returns a Spliterator.OfLong covering all of the specified array. |
98 |
static <T> Spliterator<T> spliterator(T[] array) This method returns a Spliterator covering all of the specified array. |
99 |
static DoubleStream stream(double[] array) This method returns a sequential DoubleStream with the specified array as its source. |
100 |
static IntStream stream(int[] array) This method returns a sequential IntStream with the specified array as its source. |
101 |
static LongStream stream(long[] array) This method returns a sequential LongStream with the specified array as its source. |
102 |
static <T> Stream<T> stream(T[] array) This method returns a sequential Stream with the specified array as its source. |
103 |
This method sorts the specified array of bytes into ascending numerical order. |
104 |
This method sorts the specified array of chars into ascending numerical order. |
105 |
This method sorts the specified array of doubles into ascending numerical order. |
106 |
This method sorts the specified array of floats into ascending numerical order. |
107 |
This method sorts the specified array of ints into ascending numerical order. |
108 |
This method sorts the specified array of longs into ascending numerical order. |
109 |
This method sorts the specified array of objects into ascending order, according to the natural ordering of its elements. |
110 |
This method sorts the specified array of shorts into ascending numerical order. |
111 |
static String toString(boolean[] a) This method returns a string representation of the contents of the specified array of boolean. |
Methods Inherited
This class inherits methods from the following classes −
java.util.Object
Arrays Class Example
This Java example demonstrates the binarySearch() method of Arrays class.
// Importing the Arrays class import java.util.Arrays; // Public Main Class public class Main { public static void main(String[] args) { // Declaring an array int arr[] = {8, 5, 3, 10, 2, 1, 15, 20}; // Sorting the array Arrays.sort(arr); // Taking an element to search int ele = 15; // Using binarySearch() method to search "ele" System.out.println( ele + " presents at the index = " + Arrays.binarySearch(arr, ele)); } }
Let us compile and run the above program, this will produce the following result −
15 presents at the index = 6