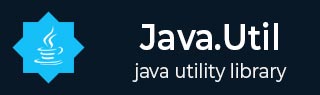
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Arrays deepEquals() Method
Description
The Java Arrays deepEquals(Object[] a1, Object[] a2) method returns true if the two specified arrays are deeply equal to one another.Two array references are considered deeply equal if both are null, or if they refer to arrays that contain the same number of elements and all corresponding pairs of elements in the two arrays are deeply equal.
Two possibly null elements e1 and e2 are deeply equal if any of the following conditions hold −
e1 and e2 are both arrays of object reference types, and Arrays.deepEquals(e1, e2) would return true
e1 and e2 are arrays of the same primitive type, and the appropriate overloading of Arrays.equals(e1,e2) would return true.
e1 == e2
e1.equals(e2) would return true.
Declaration
Following is the declaration for java.util.Arrays.deepEquals() method
public static boolean deepEquals(Object[] a1, Object[] a2)
Parameters
a1 − This is the array to be tested for equality.
a2 − This is the other array to be tested for equality.
Return Value
This method returns true if the two arrays are equal, else false.
Exception
NA
Checking Arrays of chars for Deep Equality Example
The following example shows the usage of Java Arrays deepEquals(Object[], Object[]) method. In this example, we've created three arrays of objects using chars, and compared them using deepEquals() method. As per the result, the comparison is printed.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initializing Object array1 Object[] b1 = new Object[] { 'a', 'b' }; // let us print all the values available in array2 System.out.println("Elements of Array1 is:"); for (Object value : b1) { System.out.println("Value = " + value); } // initializing Object array2 Object[] b2 = new Object[] { 'a', 'b' }; // let us print all the values available in array2 System.out.println("Elements of Array2 is:"); for (Object value : b2) { System.out.println("Value = " + value); } // initializing Object array3 Object[] b3 = new Object[] { 'x', 'y' }; // let us print all the values available in array3 System.out.println("Elements of Array3 is:"); for (Object value : b3) { System.out.println("Value = " + value); } // checking array1 and array2 for equality System.out.println("Array1 and Array2 are equal:" + Arrays.deepEquals(b1,b2)); // checking array1 and array3 for equality System.out.println("Array1 and Array3 are equal:" + Arrays.deepEquals(b1,b3)); } }
Output
Let us compile and run the above program, this will produce the following result −
Elements of Array1 is: Value = a Value = b Elements of Array2 is: Value = a Value = b Elements of Array3 is: Value = x Value = y Array1 and Array2 are equal:true Array1 and Array3 are equal:false
Checking Arrays of Objects for Deep Equality Example
The following example shows the usage of Java Arrays deepEquals(Object[], Object[]) method. In this example, we've created three arrays of objects using Student objects, and compared them using deepEquals() method. As per the result, the comparison is printed.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initializing Object array1 Student[] b1 = new Student[] { new Student(1, "Julie"), new Student(2, "Robert") }; // let us print all the values available in array2 System.out.println("Elements of Array1 is:"); for (Object value : b1) { System.out.println("Value = " + value); } // initializing Object array2 Student[] b2 = new Student[] { new Student(1, "Julie"), new Student(2, "Robert") }; // let us print all the values available in array2 System.out.println("Elements of Array2 is:"); for (Object value : b2) { System.out.println("Value = " + value); } // initializing Object array3 Student[] b3 = new Student[] { new Student(1, "Julie"), new Student(3, "Adam") }; // let us print all the values available in array3 System.out.println("Elements of Array3 is:"); for (Object value : b3) { System.out.println("Value = " + value); } // checking array1 and array2 for equality System.out.println("Array1 and Array2 are equal:" + Arrays.deepEquals(b1,b2)); // checking array1 and array3 for equality System.out.println("Array1 and Array3 are equal:" + Arrays.deepEquals(b1,b3)); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
Output
Let us compile and run the above program, this will produce the following result −
Elements of Array1 is: Value = [ 1, Julie ] Value = [ 2, Robert ] Elements of Array2 is: Value = [ 1, Julie ] Value = [ 2, Robert ] Elements of Array3 is: Value = [ 1, Julie ] Value = [ 3, Adam ] Array1 and Array2 are equal:true Array1 and Array3 are equal:false
Checking Arrays of Integers for Deep Equality Example
The following example shows the usage of Java Arrays deepEquals(Object[], Object[]) method. In this example, we've created three arrays of Integer objects and compared them using deepEquals() method. As per the result, the comparison is printed.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initializing Object array1 Integer[] b1 = new Integer[] { 1, 2 }; // let us print all the values available in array2 System.out.println("Elements of Array1 is:"); for (Object value : b1) { System.out.println("Value = " + value); } // initializing Object array2 Integer[] b2 = new Integer[] { 1, 2 }; // let us print all the values available in array2 System.out.println("Elements of Array2 is:"); for (Object value : b2) { System.out.println("Value = " + value); } // initializing Object array3 Integer[] b3 = new Integer[] { 1, 3 }; // let us print all the values available in array3 System.out.println("Elements of Array3 is:"); for (Object value : b3) { System.out.println("Value = " + value); } // checking array1 and array2 for equality System.out.println("Array1 and Array2 are equal:" + Arrays.deepEquals(b1,b2)); // checking array1 and array3 for equality System.out.println("Array1 and Array3 are equal:" + Arrays.deepEquals(b1,b3)); } }
Output
Let us compile and run the above program, this will produce the following result −
Elements of Array1 is: Value = 1 Value = 2 Elements of Array2 is: Value = 1 Value = 2 Elements of Array3 is: Value = 1 Value = 3 Array1 and Array2 are equal:true Array1 and Array3 are equal:false
To Continue Learning Please Login