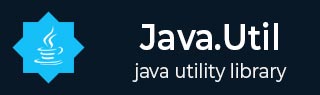
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java WeakHashMap Class
Introduction
The Java WeakHashMap class is a hashtable-based Map implementation with weak keys. An entry in a WeakHashMap will automatically be removed by the garbage collector, when its key is no longer in use. Following are the important points about WeakHashMap −
Both null values and the null key are supported.
Like most collection classes, this class is also not synchronized.
This class is intended primarily for use with key objects whose equals methods test for object identity using the == operator.
Each key object in a WeakHashMap is stored indirectly as the referent of a weak reference.
This class is a member of the Java Collections Framework.
Class declaration
Following is the declaration for java.util.WeakHashMap class −
public class WeakHashMap<K,V> extends AbstractMap<K,V> implements Map<K,V>
Here <K> is the type of keys maintained by this map and <V> is the type of mapped values.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | WeakHashMap() This constructor is used to create an empty WeakHashMap with the default initial capacity (16) and load factor (0.75). |
2 | WeakHashMap(int initialCapacity) This constructor is used to create an empty WeakHashMap with the given initial capacity and the default load factor (0.75). |
3 | WeakHashMap(int initialCapacity, float loadFactor) This constructor is used to create an empty WeakHashMap with the given initial capacity and the given load factor. |
4 | WeakHashMap(Map<? extends K,? extends V> m) This constructor is used to create a new WeakHashMap with the same mappings as the specified map. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | void clear()
This method removes all of the mappings from this map. |
2 | boolean containsKey(Object key)
This method returns true if this map contains a mapping for the specified key. |
3 | boolean containsValue(Object value)
This method returns true if this map maps one or more keys to the specified value. |
4 | Set<Map.Entry>K,V>> entrySet()
This method returns a Set view of the mappings contained in this map. |
5 | v get(Object key)
This method returns the value to which the specified key is mapped, or null if this map contains no mapping for the key. |
6 | boolean isEmpty()
This method returns true if this map contains no key-value mappings. |
7 | Set<K> keySet()
This method returns a Set view of the keys contained in this map. |
8 | v put(K key, V value)
This method associates the specified value with the specified key in this map. |
9 | void putAll(Map<? extends K,? extends V> m)
This method copies all of the mappings from the specified map to this map. |
10 | v remove(Object key)
This method removes the mapping for a key from this weak hash map if it is present. |
11 | int size()
This method returns the number of key-value mappings in this map. |
12 | Collection<V> values()
This method returns a Collection view of the values contained in this map. |
Methods inherited
This class inherits methods from the following classes −
- java.util.AbstractMap
- java.lang.Object
- java.util.Map
Adding a Key-Value Pair to a WeakHashMap of Integer, Integer Pairs Example
The following example shows the usage of Java WeakHashMap put() method to put few values in a Map. We've created a Map object of Integer,Integer pairs. Then few entries are added using put() method and then map is printed.
package com.tutorialspoint; import java.util.WeakHashMap; public class WeakHashMapDemo { public static void main(String args[]) { // create hash map WeakHashMap<Integer,Integer> newmap = new WeakHashMap<>(); // populate hash map newmap.put(1, 1); newmap.put(2, 2); newmap.put(3, 3); System.out.println("Map elements: " + newmap); } }
Output
Let us compile and run the above program, this will produce the following result.
Map elements: {3=3, 2=2, 1=1}