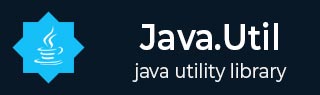
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java HashSet Class
Introduction
The Java HashSet class implements the Set interface, backed by a hash table.Following are the important points about HashSet −
This class makes no guarantees as to the iteration order of the set; in particular, it does not guarantee that the order will remain constant over time.
This class permits the null element.
Class declaration
Following is the declaration for java.util.HashSet class −
public class HashSet<E> extends AbstractSet<E> implements Set<E>, Cloneable, Serializable
Parameters
Following is the parameter for java.util.HashSet class −
E − This is the type of elements maintained by this set.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | HashSet() This constructs a new, empty set; the backing HashMap instance has default initial capacity (16) and load factor (0.75). |
2 | HashSet(Collection<? extends E> c) This constructs a new set containing the elements in the specified collection. |
3 | HashSet(int initialCapacity) This constructs a new, empty set; the backing HashMap instance has the specified initial capacity and default load factor (0.75). |
4 | HashSet(int initialCapacity, float loadFactor) This constructs a new, empty set; the backing HashMap instance has the specified initial capacity and the specified load factor. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | boolean add(E e)
This method adds the specified element to this set if it is not already present. |
2 | void clear()
This method removes all of the elements from this set. |
3 | Object clone()
This method returns a shallow copy of this HashSet instance, the elements themselves are not cloned. |
4 | boolean contains(Object o)
This method returns true if this set contains the specified element. |
5 | boolean isEmpty()
This method returns true if this set contains no elements. |
6 | Iterator<E> iterator()
This method returns an iterator over the elements in this set. |
7 | boolean remove(Object o)
This method removes the specified element from this set if it is present. |
8 | int size()
This method returns returns the number of elements in this set(its cardinality). |
9 | Spliterator<E> spliterator()
This method returns a late-binding and fail-fast Spliterator over the elements in this set. |
Methods inherited
This class inherits methods from the following classes −
- java.util.AbstractSet
- java.util.AbstractCollection
- java.util.Object
- java.util.Set