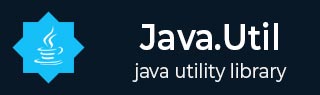
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Dictionary Class
Introduction
The Java Dictionary class is the abstract parent of any class, such as Hashtable, which maps keys to values.Following are the important points about Dictionary −
In this class every key and every value is an object.
In his class object every key is associated with at most one value.
Class declaration
Following is the declaration for java.util.Dictionary class −
public abstract class Dictionary<K,V> extends Object
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | Dictionary() This is the single constructor. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | abstract Enumeration<V> elements()
This method returns an enumeration of the values in this dictionary. |
2 | abstract V get(Object key)
This method returns the value to which the key is mapped in this dictionary. |
3 | abstract boolean isEmpty()
This method tests if this dictionary maps no keys to value. |
4 | abstract Enumeration<K> keys()
This method returns an enumeration of the keys in this dictionary. |
5 | abstract V put(K key, V value)
This method maps the specified key to the specified value in this dictionary. |
6 | abstract V remove(Object key)
This method removes the key (and its corresponding value) from this dictionary. |
7 | abstract int size()
This method returns the number of entries (distinct keys) in this dictionary. |
Methods inherited
This class inherits methods from the following classes −
- java.util.Object
Adding a Mapping to Dictionary of Integer, Integer Example
The following example shows the usage of Java Dictionary put(K,V) method. We're creating a dictionary instance using Hashtable object of Integer, Integer. Then we've added few elements to it using put(K,V) method. An enumeration is retrieved using elements() method and enumeration is then iterated to print the elements of the dictionary.
package com.tutorialspoint; import java.util.Enumeration; import java.util.Dictionary; import java.util.Hashtable; public class DictionaryDemo { public static void main(String[] args) { // create a new hashtable Dictionary<Integer, Integer> dictionary = new Hashtable<>(); // add 2 elements dictionary.put(1, 1); dictionary.put(2, 2); Enumeration<Integer> enumeration = dictionary.elements(); while(enumeration.hasMoreElements()) { System.out.println(enumeration.nextElement()); } } }
Let us compile and run the above program, this will produce the following result −
2 1