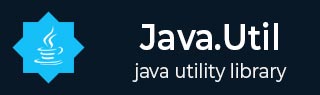
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java HashMap Class
Introduction
The Java HashMap class is the Hash table based implementation of the Map interface.Following are the important points about HashMap −
This class makes no guarantees as to the iteration order of the map; in particular, it does not guarantee that the order will remain constant over time.
This class permits null values and the null key.
Class declaration
Following is the declaration for java.util.HashMap class −
public class HashMap<K,V> extends AbstractMap<K,V> implements Map<K,V>, Cloneable, Serializable
Parameters
Following is the parameter for java.util.HashMap class −
K − This is the type of keys maintained by this map.
V − This is the type of mapped values.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | HashMap() This constructs an empty HashMap with the default initial capacity (16) and the default load factor (0.75). |
2 | HashMap(int initialCapacity) This constructs an empty HashMap with the specified initial capacity and the default load factor (0.75). |
3 | HashMap(int initialCapacity, float loadFactor) This constructs an empty HashMap with the specified initial capacity and load factor. |
4 | HashMap(Map<? extends K,? extends V> m) This constructs a new HashMap with the same mappings as the specified Map. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | void clear()
This method removes all of the mappings from this map. |
2 | Object clone()
This method returns a shallow copy of this HashMap instance, the keys and values themselves are not cloned. |
3 | V compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction)
Attempts to compute a mapping for the specified key and its current mapped value (or null if there is no current mapping). |
4 | V computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction)
If the specified key is not already associated with a value (or is mapped to null), attempts to compute its value using the given mapping function and enters it into this map unless null. |
5 | V computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction)
If the value for the specified key is present and non-null, attempts to compute a new mapping given the key and its current mapped value. |
6 | boolean containsKey(Object key)
This method returns true if this map contains a mapping for the specified key. |
7 | boolean containsValue(Object value)
This method returns true if this map maps one or more keys to the specified value. |
8 | Set<Map.Entry<K,V>> entrySet()
This method returns a Set view of the mappings contained in this map. |
9 | V get(Object key)
This method returns the value to which the specified key is mapped, or null if this map contains no mapping for the key. |
10 | boolean isEmpty()
This method returns true if this map contains no key-value mapping. |
11 | Set<K> keySet()
This method returns a Set view of the keys contained in this map. |
12 | V merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction)
If the specified key is not already associated with a value or is associated with null, associates it with the given non-null value. |
13 | V put(K key, V value)
This method associates the specified value with the specified key in this map. |
14 | void putAll(Map<? extends K,? extends V> m)
This method copies all of the mappings from the specified map to this map. |
15 | V remove(Object key)
This method removes the mapping for the specified key from this map if present. |
16 | int size()
This method returns the number of key-value mappings in this map. |
17 | Collection<V> values()
This method returns a Collection view of the values contained in this map. |
Methods inherited
This class inherits methods from the following classes −
- java.util.AbstractMap
- java.util.Object
- java.util.Map
Example
The following program illustrates several of the methods supported by HashMap collection −
import java.util.*; public class HashMapDemo { public static void main(String args[]) { // Create a hash map HashMap hm = new HashMap(); // Put elements to the map hm.put("Zara", new Double(3434.34)); hm.put("Mahnaz", new Double(123.22)); hm.put("Ayan", new Double(1378.00)); hm.put("Daisy", new Double(99.22)); hm.put("Qadir", new Double(-19.08)); // Get a set of the entries Set set = hm.entrySet(); // Get an iterator Iterator i = set.iterator(); // Display elements while(i.hasNext()) { Map.Entry me = (Map.Entry)i.next(); System.out.print(me.getKey() + ": "); System.out.println(me.getValue()); } System.out.println(); // Deposit 1000 into Zara's account double balance = ((Double)hm.get("Zara")).doubleValue(); hm.put("Zara", new Double(balance + 1000)); System.out.println("Zara's new balance: " + hm.get("Zara")); } }
This will produce the following result −
Output
Daisy: 99.22 Ayan: 1378.0 Zara: 3434.34 Qadir: -19.08 Mahnaz: 123.22 Zara's new balance: 4434.34