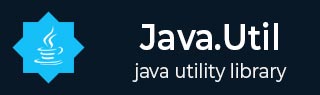
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Arrays toString(boolean[])Method
Description
The Java Arrays toString(boolean[]) method returns a string representation of the contents of the specified array. It consists of a list of the array's elements, enclosed by square brackets ("[]"). Adjacent elements are separated by the characters ", ". String.valueOf(boolean) is used to convert elements to String. Returns "null" if a is null.
Declaration
Following is the declaration for java.util.Arrays.toString() method
public static String toString(boolean[] a)
Parameters
a − This is the array whose string representation is to be returned.
Return Value
This method returns a string representation for a.
Exception
NA
Java Arrays toString(byte[]) Method
Description
The Java Arrays toString(byte[]) method returns a string representation of the contents of the specified array. It consists of a list of the array's elements, enclosed by square brackets ("[]"). Adjacent elements are separated by the characters ", ". String.valueOf(byte) is used to convert elements to String. Returns "null" if a is null.
Declaration
Following is the declaration for java.util.Arrays.toString() method
public static String toString(byte[] a)
Parameters
a − This is the array whose string representation is to be returned.
Return Value
This method returns a string representation for a.
Exception
NA
Java Arrays toString(char[]) Method
Description
The Java Arrays toString(char[]) method returns a string representation of the contents of the specified array. It consists of a list of the array's elements, enclosed by square brackets ("[]"). Adjacent elements are separated by the characters ", ". String.valueOf(char) is used to convert elements to String. Returns "null" if a is null.
Declaration
Following is the declaration for java.util.Arrays.toString() method
public static String toString(char[] a)
Parameters
a − This is the array whose string representation is to be returned.
Return Value
This method returns a string representation for a.
Exception
NA
Java Arrays toString(double[]) Method
Description
The Java Arrays toString(double[]) method returns a string representation of the contents of the specified array. It consists of a list of the array's elements, enclosed by square brackets ("[]"). Adjacent elements are separated by the characters ", ". String.valueOf(double) is used to convert elements to String. Returns "null" if a is null.
Declaration
Following is the declaration for java.util.Arrays.toString() method
public static String toString(double[] a)
Parameters
a − This is the array whose string representation is to be returned.
Return Value
This method returns a string representation for a.
Exception
NA
Java Arrays toString(float[]) Method
Description
The Java Arrays toString(float[]) method returns a string representation of the contents of the specified array. It consists of a list of the array's elements, enclosed by square brackets ("[]"). Adjacent elements are separated by the characters ", ". String.valueOf(float) is used to convert elements to String. Returns "null" if a is null.
Declaration
Following is the declaration for java.util.Arrays.toString() method
public static String toString(float[] a)
Parameters
a − This is the array whose string representation is to be returned.
Return Value
This method returns a string representation for a.
Exception
NA
Java Arrays toString(int[]) Method
Description
The Java Arrays toString(int[]) method returns a string representation of the contents of the specified array. It consists of a list of the array's elements, enclosed by square brackets ("[]"). Adjacent elements are separated by the characters ", ". String.valueOf(int) is used to convert elements to String. Returns "null" if a is null.
Declaration
Following is the declaration for java.util.Arrays.toString() method
public static String toString(int[] a)
Parameters
a − This is the array whose string representation is to be returned.
Return Value
This method returns a string representation for a.
Exception
NA
Java Arrays toString(long[]) Method
Description
The Java Arrays toString(long[]) method returns a string representation of the contents of the specified array. It consists of a list of the array's elements, enclosed by square brackets ("[]"). Adjacent elements are separated by the characters ", ". String.valueOf(long) is used to convert elements to String. Returns "null" if a is null.
Declaration
Following is the declaration for java.util.Arrays.toString() method
public static String toString(long[] a)
Parameters
a − This is the array whose string representation is to be returned.
Return Value
This method returns a string representation for a.
Exception
NA
Java Arrays toString(short[]) Method
Description
The Java Arrays toString(short[]) method returns a string representation of the contents of the specified array. It consists of a list of the array's elements, enclosed by square brackets ("[]"). Adjacent elements are separated by the characters ", ". String.valueOf(short) is used to convert elements to String. Returns "null" if a is null.
Declaration
Following is the declaration for java.util.Arrays.toString() method
public static String toString(short[] a)
Parameters
a − This is the array whose string representation is to be returned.
Return Value
This method returns a string representation for a.
Exception
NA
Java Arrays toString(Object[]) Method
Description
The Java Arrays toString(Object[]) method returns a string representation of the contents of the specified array. It consists of a list of the array's elements, enclosed by square brackets ("[]"). Adjacent elements are separated by the characters ", ". Arrays.asList(a).toString() is used to convert elements to String. Returns "null" if a is null.
Declaration
Following is the declaration for java.util.Arrays.toString() method
public static String toString(Object[] a)
Parameters
a − This is the array whose string representation is to be returned.
Return Value
This method returns a string representation for a.
Exception
NA
Getting String Representation of Arrays of boolean, byte, char and double Example
The following example shows the usage of Java Arrays toString() methods for boolean[], byte[], char[] and double[]. First, we've created four arrays of boolean, byte, char and double and then their string representation is printed using Arrays toString() method.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initializing arrays boolean[] boolArr = new boolean[] { true, true }; byte[] byteArr = new byte[] {10, 20 }; char[] charArr = new char[] {'A', 'B' }; double[] doubleArr = new double[] {10.0, 20.0 }; // printing hash code value System.out.println("The string representation of boolean array is: " + Arrays.toString(boolArr)); System.out.println("The string representation of byte array is: " + Arrays.toString(byteArr)); System.out.println("The string representation of char array is: " + Arrays.toString(charArr)); System.out.println("The string representation of double array is: " + Arrays.toString(doubleArr)); } }
Output
Let us compile and run the above program, this will produce the following result −
The string representation of boolean array is: [true, true] The string representation of byte array is: [10, 20] The string representation of char array is: [A, B] The string representation of double array is: [10.0, 20.0]
Getting String Representation of Arrays of float, int, long and short Example
The following example shows the usage of Java Arrays toString() methods for float[], int[], long[] and short[]. First, we've created four arrays of float, int, long and short and then their string representation is printed using Arrays toString() method.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initializing arrays float[] floatArr = new float[] { 10.0f, 20.f }; int[] intArr = new int[] {10, 20 }; long[] longArr = new long[] { 10L, 20L }; short[] shortArr = new short[] {10, 20 }; // printing hash code value System.out.println("The string representation of float array is: " + Arrays.toString(floatArr)); System.out.println("The string representation of int array is: " + Arrays.toString(intArr)); System.out.println("The string representation of long array is: " + Arrays.toString(longArr)); System.out.println("The string representation of short array is: " + Arrays.toString(shortArr)); } }
Output
Let us compile and run the above program, this will produce the following result −
The string representation of float array is: [10.0, 20.0] The string representation of int array is: [10, 20] The string representation of long array is: [10, 20] The string representation of short array is: [10, 20]
Getting String Representation of Arrays of Object Example
The following example shows the usage of Java Arrays toString() methods for Object[]. First, we've created an array of Student object and then their string representation is printed using Arrays toString() method.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initializing array Student[] students = { new Student(1, "Julie"), new Student(3, "Adam"), new Student(2, "Robert") }; // printing hash code value System.out.println("The string representation of Student array is: " + Arrays.toString(students)); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result −
The string representation of Student array is: [[ 1, Julie ], [ 3, Adam ], [ 2, Robert ]]