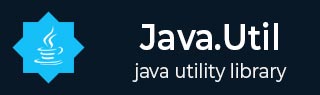
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java.util.Arrays.sort(Object[ ]) Method
Description
The java.util.Arrays.sort(Object[]) method sorts the specified array of Objects into ascending order according to the natural ordering of its elements.
Declaration
Following is the declaration for java.util.Arrays.sort() method
public static void sort(Object[] a)
Parameters
a − This is the array to be sorted.
Return Value
This method does not return any value.
Exception
ClassCastException − if the array contains elements that are not mutually comparable (for example, strings and integers).
Example
The following example shows the usage of java.util.Arrays.sort() method.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initializing unsorted Object array Object ob[] = {27, 11, 44}; // let us print all the elements available in list for (Object number : ob) { System.out.println("Number = " + number); } // sorting array Arrays.sort(ob); // let us print all the elements available in list System.out.println("The sorted Object array is:"); for (Object number : ob) { System.out.println("Number = " + number); } } }
Let us compile and run the above program, this will produce the following result −
Number = 27 Number = 11 Number = 44 The sorted Object array is: Number = 11 Number = 27 Number = 44
java_util_arrays.htm
Advertisements