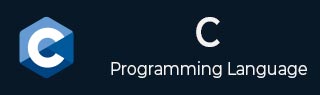
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <float.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdarg.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <time.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C Standard Library - Quick Guide
C Library - <assert.h>
The assert.h header file of the C Standard Library provides a macro called assert which can be used to verify assumptions made by the program and print a diagnostic message if this assumption is false.
The defined macro assert refers to another macro NDEBUG which is not a part of <assert.h>. If NDEBUG is defined as a macro name in the source file, at the point where <assert.h> is included, the assert macro is defined as follows −
#define assert(ignore) ((void)0)
Library Macros
Following is the only function defined in the header assert.h −
Sr.No. | Function & Description |
---|---|
1 |
void assert(int expression)
This is actually a macro and not a function, which can be used to add diagnostics in your C program. |
C Library - <ctype.h>
The ctype.h header file of the C Standard Library declares several functions that are useful for testing and mapping characters.
All the functions accepts int as a parameter, whose value must be EOF or representable as an unsigned char.
All the functions return non-zero (true) if the argument c satisfies the condition described, and zero(false) if not.
Library Functions
Following are the functions defined in the header ctype.h −
Sr.No. | Function & Description |
---|---|
1 |
int isalnum(int c)
This function checks whether the passed character is alphanumeric. |
2 |
int isalpha(int c)
This function checks whether the passed character is alphabetic. |
3 |
int iscntrl(int c)
This function checks whether the passed character is control character. |
4 |
int isdigit(int c)
This function checks whether the passed character is decimal digit. |
5 |
int isgraph(int c)
This function checks whether the passed character has graphical representation using locale. |
6 |
int islower(int c)
This function checks whether the passed character is lowercase letter. |
7 |
int isprint(int c)
This function checks whether the passed character is printable. |
8 |
int ispunct(int c)
This function checks whether the passed character is a punctuation character. |
9 |
int isspace(int c)
This function checks whether the passed character is white-space. |
10 |
int isupper(int c)
This function checks whether the passed character is an uppercase letter. |
11 |
int isxdigit(int c)
This function checks whether the passed character is a hexadecimal digit. |
The library also contains two conversion functions that accepts and returns an "int".
Sr.No. | Function & Description |
---|---|
1 |
int tolower(int c)
This function converts uppercase letters to lowercase. |
2 |
int toupper(int c)
This function converts lowercase letters to uppercase. |
Character Classes
Sr.No. | Character Class & Description |
---|---|
1 |
Digits This is a set of whole numbers { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 }. |
2 |
Hexadecimal digits This is the set of { 0 1 2 3 4 5 6 7 8 9 A B C D E F a b c d e f }. |
3 |
Lowercase letters This is a set of lowercase letters { a b c d e f g h i j k l m n o p q r s t u v w x y z }. |
4 |
Uppercase letters This is a set of uppercase letters {A B C D E F G H I J K L M N O P Q R S T U V W X Y Z }. |
5 |
Letters This is a set of lowercase and uppercase letters. |
6 |
Alphanumeric characters This is a set of Digits, Lowercase letters and Uppercase letters. |
7 |
Punctuation characters This is a set of ! " # $ % & ' ( ) * + , - . / : ; < = > ? @ [ \ ] ^ _ ` { | } ~ |
8 |
Graphical characters This is a set of Alphanumeric characters and Punctuation characters. |
9 |
Space characters This is a set of tab, newline, vertical tab, form feed, carriage return, and space. |
10 |
Printable characters This is a set of Alphanumeric characters, Punctuation characters and Space characters. |
11 |
Control characters In ASCII, these characters have octal codes 000 through 037, and 177 (DEL). |
12 |
Blank characters These are spaces and tabs. |
13 |
Alphabetic characters This is a set of Lowercase letters and Uppercase letters. |
C Library - <errno.h>
The errno.h header file of the C Standard Library defines the integer variable errno, which is set by system calls and some library functions in the event of an error to indicate what went wrong. This macro expands to a modifiable lvalue of type int, therefore it can be both read and modified by a program.
The errno is set to zero at program startup. Certain functions of the standard C library modify its value to other than zero to signal some types of error. You can also modify its value or reset to zero at your convenience.
The errno.h header file also defines a list of macros indicating different error codes, which will expand to integer constant expressions with type int.
Library Macros
Following are the macros defined in the header errno.h −
Sr.No. | Macro & Description |
---|---|
1 |
extern int errno
This is the macro set by system calls and some library functions in the event of an error to indicate what went wrong. |
2 |
EDOM Domain Error
This macro represents a domain error, which occurs if an input argument is outside the domain, over which the mathematical function is defined and errno is set to EDOM. |
3 |
ERANGE Range Error
This macro represents a range error, which occurs if an input argument is outside the range, over which the mathematical function is defined and errno is set to ERANGE. |
C Library - <float.h>
The float.h header file of the C Standard Library contains a set of various platform-dependent constants related to floating point values. These constants are proposed by ANSI C. They allow making more portable programs. Before checking all the constants, it is good to understand that floating-point number is composed of following four elements −
Sr.No. | Component & Component Description |
---|---|
1 |
S sign ( +/- ) |
2 |
b base or radix of the exponent representation, 2 for binary, 10 for decimal, 16 for hexadecimal, and so on... |
3 |
e exponent, an integer between a minimum emin and a maximum emax. |
4 |
p precision, the number of base-b digits in the significand. |
Based on the above 4 components, a floating point will have its value as follows −
floating-point = ( S ) p x be or floating-point = (+/-) precision x baseexponent
Library Macros
The following values are implementation-specific and defined with the #define directive, but these values may not be any lower than what is given here. Note that in all instances FLT refers to type float, DBL refers to double, and LDBL refers to long double.
Sr.No. | Macro & Description |
---|---|
1 | FLT_ROUNDS Defines the rounding mode for floating point addition and it can have any of the following values −
|
2 | FLT_RADIX 2 This defines the base radix representation of the exponent. A base-2 is binary, base-10 is the normal decimal representation, base-16 is Hex. |
3 | FLT_MANT_DIG DBL_MANT_DIG LDBL_MANT_DIG These macros define the number of digits in the number (in the FLT_RADIX base). |
4 | FLT_DIG 6 DBL_DIG 10 LDBL_DIG 10 These macros define the maximum number decimal digits (base-10) that can be represented without change after rounding. |
5 | FLT_MIN_EXP DBL_MIN_EXP LDBL_MIN_EXP These macros define the minimum negative integer value for an exponent in base FLT_RADIX. |
6 | FLT_MIN_10_EXP -37 DBL_MIN_10_EXP -37 LDBL_MIN_10_EXP -37 These macros define the minimum negative integer value for an exponent in base 10. |
7 | FLT_MAX_EXP DBL_MAX_EXP LDBL_MAX_EXP These macros define the maximum integer value for an exponent in base FLT_RADIX. |
8 | FLT_MAX_10_EXP +37 DBL_MAX_10_EXP +37 LDBL_MAX_10_EXP +37 These macros define the maximum integer value for an exponent in base 10. |
9 | FLT_MAX 1E+37 DBL_MAX 1E+37 LDBL_MAX 1E+37 These macros define the maximum finite floating-point value. |
10 | FLT_EPSILON 1E-5 DBL_EPSILON 1E-9 LDBL_EPSILON 1E-9 These macros define the least significant digit representable. |
11 | FLT_MIN 1E-37 DBL_MIN 1E-37 LDBL_MIN 1E-37 These macros define the minimum floating-point values. |
Example
The following example shows the usage of few of the constants defined in float.h file.
Live Demo#include <stdio.h> #include <float.h> int main () { printf("The maximum value of float = %.10e\n", FLT_MAX); printf("The minimum value of float = %.10e\n", FLT_MIN); printf("The number of digits in the number = %.10e\n", FLT_MANT_DIG); }
Let us compile and run the above program that will produce the following result −
The maximum value of float = 3.4028234664e+38 The minimum value of float = 1.1754943508e-38 The number of digits in the number = 7.2996655210e-312
C Library - <limits.h>
The limits.h header determines various properties of the various variable types. The macros defined in this header, limits the values of various variable types like char, int and long.
These limits specify that a variable cannot store any value beyond these limits, for example an unsigned character can store up to a maximum value of 255.
Library Macros
The following values are implementation-specific and defined with the #define directive, but these values may not be any lower than what is given here.
Macro | Value | Description |
---|---|---|
CHAR_BIT | 8 | Defines the number of bits in a byte. |
SCHAR_MIN | -128 | Defines the minimum value for a signed char. |
SCHAR_MAX | +127 | Defines the maximum value for a signed char. |
UCHAR_MAX | 255 | Defines the maximum value for an unsigned char. |
CHAR_MIN | -128 | Defines the minimum value for type char and its value will be equal to SCHAR_MIN if char represents negative values, otherwise zero. |
CHAR_MAX | +127 | Defines the value for type char and its value will be equal to SCHAR_MAX if char represents negative values, otherwise UCHAR_MAX. |
MB_LEN_MAX | 16 | Defines the maximum number of bytes in a multi-byte character. |
SHRT_MIN | -32768 | Defines the minimum value for a short int. |
SHRT_MAX | +32767 | Defines the maximum value for a short int. |
USHRT_MAX | 65535 | Defines the maximum value for an unsigned short int. |
INT_MIN | -2147483648 | Defines the minimum value for an int. |
INT_MAX | +2147483647 | Defines the maximum value for an int. |
UINT_MAX | 4294967295 | Defines the maximum value for an unsigned int. |
LONG_MIN | -9223372036854775808 | Defines the minimum value for a long int. |
LONG_MAX | +9223372036854775807 | Defines the maximum value for a long int. |
ULONG_MAX | 18446744073709551615 | Defines the maximum value for an unsigned long int. |
Example
The following example shows the usage of few of the constants defined in limits.h file.
Live Demo#include <stdio.h> #include <limits.h> int main() { printf("The number of bits in a byte %d\n", CHAR_BIT); printf("The minimum value of SIGNED CHAR = %d\n", SCHAR_MIN); printf("The maximum value of SIGNED CHAR = %d\n", SCHAR_MAX); printf("The maximum value of UNSIGNED CHAR = %d\n", UCHAR_MAX); printf("The minimum value of SHORT INT = %d\n", SHRT_MIN); printf("The maximum value of SHORT INT = %d\n", SHRT_MAX); printf("The minimum value of INT = %d\n", INT_MIN); printf("The maximum value of INT = %d\n", INT_MAX); printf("The minimum value of CHAR = %d\n", CHAR_MIN); printf("The maximum value of CHAR = %d\n", CHAR_MAX); printf("The minimum value of LONG = %ld\n", LONG_MIN); printf("The maximum value of LONG = %ld\n", LONG_MAX); return(0); }
Let us compile and run the above program that will produce the following result −
The maximum value of UNSIGNED CHAR = 255 The minimum value of SHORT INT = -32768 The maximum value of SHORT INT = 32767 The minimum value of INT = -2147483648 The maximum value of INT = 2147483647 The minimum value of CHAR = -128 The maximum value of CHAR = 127 The minimum value of LONG = -9223372036854775808 The maximum value of LONG = 9223372036854775807
C Library - <locale.h>
The locale.h header defines the location specific settings, such as date formats and currency symbols. You will find several macros defined along with an important structure struct lconv and two important functions listed below.
Library Macros
Following are the macros defined in the header and these macros will be used in two functions listed below −
Sr.No. | Macro & Description |
---|---|
1 |
LC_ALL Sets everything. |
2 |
LC_COLLATE Affects strcoll and strxfrm functions. |
3 |
LC_CTYPE Affects all character functions. |
4 |
LC_MONETARY Affects the monetary information provided by localeconv function. |
5 |
LC_NUMERIC Affects decimal-point formatting and the information provided by localeconv function. |
6 |
LC_TIME Affects the strftime function. |
Library Functions
Following are the functions defined in the header locale.h −
Sr.No. | Function & Description |
---|---|
1 |
char *setlocale(int category, const char *locale)
Sets or reads location dependent information. |
2 |
struct lconv *localeconv(void)
Sets or reads location dependent information. |
Library Structure
typedef struct { char *decimal_point; char *thousands_sep; char *grouping; char *int_curr_symbol; char *currency_symbol; char *mon_decimal_point; char *mon_thousands_sep; char *mon_grouping; char *positive_sign; char *negative_sign; char int_frac_digits; char frac_digits; char p_cs_precedes; char p_sep_by_space; char n_cs_precedes; char n_sep_by_space; char p_sign_posn; char n_sign_posn; } lconv
Following is the description of each of the fields −
Sr.No. | Field & Description |
---|---|
1 |
decimal_point Decimal point character used for non-monetary values. |
2 |
thousands_sep Thousands place separator character used for non-monetary values. |
3 |
grouping A string that indicates the size of each group of digits in non-monetary quantities. Each character represents an integer value, which designates the number of digits in the current group. A value of 0 means that the previous value is to be used for the rest of the groups. |
4 |
int_curr_symbol It is a string of the international currency symbols used. The first three characters are those specified by ISO 4217:1987 and the fourth is the character, which separates the currency symbol from the monetary quantity. |
5 |
currency_symbol The local symbol used for currency. |
6 |
mon_decimal_point The decimal point character used for monetary values. |
7 |
mon_thousands_sep The thousands place grouping character used for monetary values. |
8 |
mon_grouping A string whose elements defines the size of the grouping of digits in monetary values. Each character represents an integer value which designates the number of digits in the current group. A value of 0 means that the previous value is to be used for the rest of the groups. |
9 |
positive_sign The character used for positive monetary values. |
10 |
negative_sign The character used for negative monetary values. |
11 |
int_frac_digits Number of digits to show after the decimal point in international monetary values. |
12 |
frac_digits Number of digits to show after the decimal point in monetary values. |
13 |
p_cs_precedes If equals to 1, then the currency_symbol appears before a positive monetary value. If equals to 0, then the currency_symbol appears after a positive monetary value. |
14 |
p_sep_by_space If equals to 1, then the currency_symbol is separated by a space from a positive monetary value. If equals to 0, then there is no space between the currency_symbol and a positive monetary value. |
15 |
n_cs_precedes If equals to 1, then the currency_symbol precedes a negative monetary value. If equals to 0, then the currency_symbol succeeds a negative monetary value. |
16 |
n_sep_by_space If equals to 1, then the currency_symbol is separated by a space from a negative monetary value. If equals to 0, then there is no space between the currency_symbol and a negative monetary value. |
17 |
p_sign_posn Represents the position of the positive_sign in a positive monetary value. |
18 |
n_sign_posn Represents the position of the negative_sign in a negative monetary value. |
The following values are used for p_sign_posn and n_sign_posn −
Value | Description |
---|---|
0 | Parentheses encapsulates the value and the currency_symbol. |
1 | The sign precedes the value and currency_symbol. |
2 | The sign succeeds the value and currency_symbol. |
3 | The sign immediately precedes the value and currency_symbol. |
4 | The sign immediately succeeds the value and currency_symbol. |
C Library - <math.h>
The math.h header defines various mathematical functions and one macro. All the functions available in this library take double as an argument and return double as the result.
Library Macros
There is only one macro defined in this library −
Sr.No. | Macro & Description |
---|---|
1 |
HUGE_VAL This macro is used when the result of a function may not be representable as a floating point number. If magnitude of the correct result is too large to be represented, the function sets errno to ERANGE to indicate a range error, and returns a particular, very large value named by the macro HUGE_VAL or its negation (- HUGE_VAL). If the magnitude of the result is too small, a value of zero is returned instead. In this case, errno might or might not be set to ERANGE. |
Library Functions
Following are the functions defined in the header math.h −
Sr.No. | Function & Description |
---|---|
1 |
double acos(double x)
Returns the arc cosine of x in radians. |
2 |
double asin(double x)
Returns the arc sine of x in radians. |
3 |
double atan(double x)
Returns the arc tangent of x in radians. |
4 |
double atan2(double y, double x)
Returns the arc tangent in radians of y/x based on the signs of both values to determine the correct quadrant. |
5 |
double cos(double x)
Returns the cosine of a radian angle x. |
6 |
double cosh(double x)
Returns the hyperbolic cosine of x. |
7 |
double sin(double x)
Returns the sine of a radian angle x. |
8 |
double sinh(double x)
Returns the hyperbolic sine of x. |
9 |
double tanh(double x)
Returns the hyperbolic tangent of x. |
10 |
double exp(double x)
Returns the value of e raised to the xth power. |
11 |
double frexp(double x, int *exponent)
The returned value is the mantissa and the integer pointed to by exponent is the exponent. The resultant value is x = mantissa * 2 ^ exponent. |
12 |
double ldexp(double x, int exponent)
Returns x multiplied by 2 raised to the power of exponent. |
13 |
double log(double x)
Returns the natural logarithm (base-e logarithm) of x. |
14 |
double log10(double x)
Returns the common logarithm (base-10 logarithm) of x. |
15 |
double modf(double x, double *integer)
The returned value is the fraction component (part after the decimal), and sets integer to the integer component. |
16 |
double pow(double x, double y)
Returns x raised to the power of y. |
17 |
double sqrt(double x)
Returns the square root of x. |
18 |
double ceil(double x)
Returns the smallest integer value greater than or equal to x. |
19 |
double fabs(double x)
Returns the absolute value of x. |
20 |
double floor(double x)
Returns the largest integer value less than or equal to x. |
21 |
double fmod(double x, double y)
Returns the remainder of x divided by y. |
C Library - <setjmp.h>
The setjmp.h header defines the macro setjmp(), one function longjmp(), and one variable type jmp_buf, for bypassing the normal function call and return discipline.
Library Variables
Following is the variable type defined in the header setjmp.h −
Sr.No. | Variable & Description |
---|---|
1 |
jmp_buf This is an array type used for holding information for macro setjmp() and function longjmp(). |
Library Macros
There is only one macro defined in this library −
Sr.No. | Macro & Description |
---|---|
1 |
int setjmp(jmp_buf environment)
This macro saves the current environment into the variable environment for later use by the function longjmp(). If this macro returns directly from the macro invocation, it returns zero but if it returns from a longjmp() function call, then a non-zero value is returned. |
Library Functions
Following is the only one function defined in the header setjmp.h −
Sr.No. | Function & Description |
---|---|
1 |
void longjmp(jmp_buf environment, int value)
This function restores the environment saved by the most recent call to setjmp() macro in the same invocation of the program with the corresponding jmp_buf argument. |
C Library - <signal.h>
The signal.h header defines a variable type sig_atomic_t, two function calls, and several macros to handle different signals reported during a program's execution.
Library Variables
Following is the variable type defined in the header signal.h −
Sr.No. | Variable & Description |
---|---|
1 |
sig_atomic_t This is of int type and is used as a variable in a signal handler. This is an integral type of an object that can be accessed as an atomic entity, even in the presence of asynchronous signals. |
Library Macros
Following are the macros defined in the header signal.h and these macros will be used in two functions listed below. The SIG_ macros are used with the signal function to define signal functions.
Sr.No. | Macro & Description |
---|---|
1 |
SIG_DFL Default signal handler. |
2 |
SIG_ERR Represents a signal error. |
3 |
SIG_IGN Signal ignore. |
The SIG macros are used to represent a signal number in the following conditions −
Sr.No. | Macro & Description |
---|---|
1 |
SIGABRT Abnormal program termination. |
2 |
SIGFPE Floating-point error like division by zero. |
3 |
SIGILL Illegal operation. |
4 |
SIGINT Interrupt signal such as ctrl-C. |
5 |
SIGSEGV Invalid access to storage like segment violation. |
6 |
SIGTERM Termination request. |
Library Functions
Following are the functions defined in the header signal.h −
Sr.No. | Function & Description |
---|---|
1 |
void (*signal(int sig, void (*func)(int)))(int)
This function sets a function to handle signal i.e. a signal handler. |
2 |
int raise(int sig)
This function causes signal sig to be generated. The sig argument is compatible with the SIG macros. |
C Library - <stdarg.h>
The stdarg.h header defines a variable type va_list and three macros which can be used to get the arguments in a function when the number of arguments are not known i.e. variable number of arguments.
A function of variable arguments is defined with the ellipsis (,...) at the end of the parameter list.
Library Variables
Following is the variable type defined in the header stdarg.h −
Sr.No. | Variable & Description |
---|---|
1 |
va_list This is a type suitable for holding information needed by the three macros va_start(), va_arg() and va_end(). |
Library Macros
Following are the macros defined in the header stdarg.h −
Sr.No. | Macro & Description |
---|---|
1 |
void va_start(va_list ap, last_arg)
This macro initializes ap variable to be used with the va_arg and va_end macros. The last_arg is the last known fixed argument being passed to the function i.e. the argument before the ellipsis. |
2 |
type va_arg(va_list ap, type)
This macro retrieves the next argument in the parameter list of the function with type type. |
3 |
void va_end(va_list ap)
This macro allows a function with variable arguments which used the va_start macro to return. If va_end is not called before returning from the function, the result is undefined. |
C Library - <stddef.h>
The stddef.h header defines various variable types and macros. Many of these definitions also appear in other headers.
Library Variables
Following are the variable types defined in the header stddef.h −
Sr.No. | Variable & Description |
---|---|
1 |
ptrdiff_t This is the signed integral type and is the result of subtracting two pointers. |
2 |
size_t This is the unsigned integral type and is the result of the sizeof keyword. |
3 |
wchar_t This is an integral type of the size of a wide character constant. |
Library Macros
Following are the macros defined in the header stddef.h −
Sr.No. | Macro & Description |
---|---|
1 |
NULL
This macro is the value of a null pointer constant. |
2 |
offsetof(type, member-designator)
This results in a constant integer of type size_t which is the offset in bytes of a structure member from the beginning of the structure. The member is given by member-designator, and the name of the structure is given in type. |
C Library - <stdio.h>
The stdio.h header defines three variable types, several macros, and various functions for performing input and output.
Library Variables
Following are the variable types defined in the header stdio.h −
Sr.No. | Variable & Description |
---|---|
1 |
size_t This is the unsigned integral type and is the result of the sizeof keyword. |
2 |
FILE This is an object type suitable for storing information for a file stream. |
3 |
fpos_t This is an object type suitable for storing any position in a file. |
Library Macros
Following are the macros defined in the header stdio.h −
Sr.No. | Macro & Description |
---|---|
1 |
NULL This macro is the value of a null pointer constant. |
2 |
_IOFBF, _IOLBF and _IONBF These are the macros which expand to integral constant expressions with distinct values and suitable for the use as third argument to the setvbuf function. |
3 |
BUFSIZ This macro is an integer, which represents the size of the buffer used by the setbuf function. |
4 |
EOF This macro is a negative integer, which indicates that the end-of-file has been reached. |
5 |
FOPEN_MAX This macro is an integer, which represents the maximum number of files that the system can guarantee to be opened simultaneously. |
6 |
FILENAME_MAX This macro is an integer, which represents the longest length of a char array suitable for holding the longest possible filename. If the implementation imposes no limit, then this value should be the recommended maximum value. |
7 |
L_tmpnam This macro is an integer, which represents the longest length of a char array suitable for holding the longest possible temporary filename created by the tmpnam function. |
8 |
SEEK_CUR, SEEK_END, and SEEK_SET These macros are used in the fseek function to locate different positions in a file. |
9 |
TMP_MAX This macro is the maximum number of unique filenames that the function tmpnam can generate. |
10 |
stderr, stdin, and stdout These macros are pointers to FILE types which correspond to the standard error, standard input, and standard output streams. |
Library Functions
Following are the functions defined in the header stdio.h −
Sr.No. | Function & Description |
---|---|
1 |
int fclose(FILE *stream)
Closes the stream. All buffers are flushed. |
2 |
void clearerr(FILE *stream)
Clears the end-of-file and error indicators for the given stream. |
3 |
int feof(FILE *stream)
Tests the end-of-file indicator for the given stream. |
4 |
int ferror(FILE *stream)
Tests the error indicator for the given stream. |
5 |
int fflush(FILE *stream)
Flushes the output buffer of a stream. |
6 |
int fgetpos(FILE *stream, fpos_t *pos)
Gets the current file position of the stream and writes it to pos. |
7 |
FILE *fopen(const char *filename, const char *mode)
Opens the filename pointed to by filename using the given mode. |
8 |
size_t fread(void *ptr, size_t size, size_t nmemb, FILE *stream)
Reads data from the given stream into the array pointed to by ptr. |
9 |
FILE *freopen(const char *filename, const char *mode, FILE *stream)
Associates a new filename with the given open stream and same time closing the old file in stream. |
10 |
int fseek(FILE *stream, long int offset, int whence)
Sets the file position of the stream to the given offset. The argument offset signifies the number of bytes to seek from the given whence position. |
11 |
int fsetpos(FILE *stream, const fpos_t *pos)
Sets the file position of the given stream to the given position. The argument pos is a position given by the function fgetpos. |
12 |
long int ftell(FILE *stream)
Returns the current file position of the given stream. |
13 |
size_t fwrite(const void *ptr, size_t size, size_t nmemb, FILE *stream)
Writes data from the array pointed to by ptr to the given stream. |
14 |
int remove(const char *filename)
Deletes the given filename so that it is no longer accessible. |
15 |
int rename(const char *old_filename, const char *new_filename)
Causes the filename referred to, by old_filename to be changed to new_filename. |
16 |
void rewind(FILE *stream)
Sets the file position to the beginning of the file of the given stream. |
17 |
void setbuf(FILE *stream, char *buffer)
Defines how a stream should be buffered. |
18 |
int setvbuf(FILE *stream, char *buffer, int mode, size_t size)
Another function to define how a stream should be buffered. |
19 |
FILE *tmpfile(void)
Creates a temporary file in binary update mode (wb+). |
20 |
char *tmpnam(char *str)
Generates and returns a valid temporary filename which does not exist. |
21 |
int fprintf(FILE *stream, const char *format, ...)
Sends formatted output to a stream. |
22 |
int printf(const char *format, ...)
Sends formatted output to stdout. |
23 |
int sprintf(char *str, const char *format, ...)
Sends formatted output to a string. |
24 |
int vfprintf(FILE *stream, const char *format, va_list arg)
Sends formatted output to a stream using an argument list. |
25 |
int vprintf(const char *format, va_list arg)
Sends formatted output to stdout using an argument list. |
26 |
int vsprintf(char *str, const char *format, va_list arg)
Sends formatted output to a string using an argument list. |
27 |
int fscanf(FILE *stream, const char *format, ...)
Reads formatted input from a stream. |
28 |
int scanf(const char *format, ...)
Reads formatted input from stdin. |
29 |
int sscanf(const char *str, const char *format, ...)
Reads formatted input from a string. |
30 |
int fgetc(FILE *stream)
Gets the next character (an unsigned char) from the specified stream and advances the position indicator for the stream. |
31 |
char *fgets(char *str, int n, FILE *stream)
Reads a line from the specified stream and stores it into the string pointed to by str. It stops when either (n-1) characters are read, the newline character is read, or the end-of-file is reached, whichever comes first. |
32 |
int fputc(int char, FILE *stream)
Writes a character (an unsigned char) specified by the argument char to the specified stream and advances the position indicator for the stream. |
33 |
int fputs(const char *str, FILE *stream)
Writes a string to the specified stream up to but not including the null character. |
34 |
int getc(FILE *stream)
Gets the next character (an unsigned char) from the specified stream and advances the position indicator for the stream. |
35 |
int getchar(void)
Gets a character (an unsigned char) from stdin. |
36 |
char *gets(char *str)
Reads a line from stdin and stores it into the string pointed to by, str. It stops when either the newline character is read or when the end-of-file is reached, whichever comes first. |
37 |
int putc(int char, FILE *stream)
Writes a character (an unsigned char) specified by the argument char to the specified stream and advances the position indicator for the stream. |
38 |
int putchar(int char)
Writes a character (an unsigned char) specified by the argument char to stdout. |
39 |
int puts(const char *str)
Writes a string to stdout up to but not including the null character. A newline character is appended to the output. |
40 |
int ungetc(int char, FILE *stream)
Pushes the character char (an unsigned char) onto the specified stream so that the next character is read. |
41 |
void perror(const char *str)
Prints a descriptive error message to stderr. First the string str is printed followed by a colon and then a space. |
C Library - <stdlib.h>
The stdlib.h header defines four variable types, several macros, and various functions for performing general functions.
Library Variables
Following are the variable types defined in the header stdlib.h −
Sr.No. | Variable & Description |
---|---|
1 |
size_t This is the unsigned integral type and is the result of the sizeof keyword. |
2 |
wchar_t This is an integer type of the size of a wide character constant. |
3 |
div_t This is the structure returned by the div function. |
4 |
ldiv_t This is the structure returned by the ldiv function. |
Library Macros
Following are the macros defined in the header stdlib.h −
Sr.No. | Macro & Description |
---|---|
1 |
NULL This macro is the value of a null pointer constant. |
2 |
EXIT_FAILURE This is the value for the exit function to return in case of failure. |
3 |
EXIT_SUCCESS This is the value for the exit function to return in case of success. |
4 |
RAND_MAX This macro is the maximum value returned by the rand function. |
5 |
MB_CUR_MAX This macro is the maximum number of bytes in a multi-byte character set which cannot be larger than MB_LEN_MAX. |
Library Functions
Following are the functions defined in the header stlib.h −
Sr.No. | Function & Description |
---|---|
1 |
double atof(const char *str)
Converts the string pointed to, by the argument str to a floating-point number (type double). |
2 |
int atoi(const char *str)
Converts the string pointed to, by the argument str to an integer (type int). |
3 |
long int atol(const char *str)
Converts the string pointed to, by the argument str to a long integer (type long int). |
4 |
double strtod(const char *str, char **endptr)
Converts the string pointed to, by the argument str to a floating-point number (type double). |
5 |
long int strtol(const char *str, char **endptr, int base)
Converts the string pointed to, by the argument str to a long integer (type long int). |
6 |
unsigned long int strtoul(const char *str, char **endptr, int base)
Converts the string pointed to, by the argument str to an unsigned long integer (type unsigned long int). |
7 |
void *calloc(size_t nitems, size_t size)
Allocates the requested memory and returns a pointer to it. |
8 |
void free(void *ptr
Deallocates the memory previously allocated by a call to calloc, malloc, or realloc. |
9 |
void *malloc(size_t size)
Allocates the requested memory and returns a pointer to it. |
10 |
void *realloc(void *ptr, size_t size)
Attempts to resize the memory block pointed to by ptr that was previously allocated with a call to malloc or calloc. |
11 |
void abort(void)
Causes an abnormal program termination. |
12 |
int atexit(void (*func)(void))
Causes the specified function func to be called when the program terminates normally. |
13 |
void exit(int status)
Causes the program to terminate normally. |
14 |
char *getenv(const char *name)
Searches for the environment string pointed to by name and returns the associated value to the string. |
15 |
int system(const char *string)
The command specified by string is passed to the host environment to be executed by the command processor. |
16 |
void *bsearch(const void *key, const void *base, size_t nitems, size_t size, int (*compar)(const void *, const void *))
Performs a binary search. |
17 |
void qsort(void *base, size_t nitems, size_t size, int (*compar)(const void *, const void*))
Sorts an array. |
18 |
int abs(int x)
Returns the absolute value of x. |
19 |
div_t div(int numer, int denom)
Divides numer (numerator) by denom (denominator). |
20 |
long int labs(long int x)
Returns the absolute value of x. |
21 |
ldiv_t ldiv(long int numer, long int denom)
Divides numer (numerator) by denom (denominator). |
22 |
int rand(void)
Returns a pseudo-random number in the range of 0 to RAND_MAX. |
23 |
void srand(unsigned int seed)
This function seeds the random number generator used by the function rand. |
24 |
int mblen(const char *str, size_t n)
Returns the length of a multibyte character pointed to by the argument str. |
25 |
size_t mbstowcs(schar_t *pwcs, const char *str, size_t n)
Converts the string of multibyte characters pointed to by the argument str to the array pointed to by pwcs. |
26 |
int mbtowc(whcar_t *pwc, const char *str, size_t n)
Examines the multibyte character pointed to by the argument str. |
27 |
size_t wcstombs(char *str, const wchar_t *pwcs, size_t n)
Converts the codes stored in the array pwcs to multibyte characters and stores them in the string str. |
28 |
int wctomb(char *str, wchar_t wchar)
Examines the code which corresponds to a multibyte character given by the argument wchar. |
C Library - <string.h>
The string.h header defines one variable type, one macro, and various functions for manipulating arrays of characters.
Library Variables
Following is the variable type defined in the header string.h −
Sr.No. | Variable & Description |
---|---|
1 |
size_t This is the unsigned integral type and is the result of the sizeof keyword. |
Library Macros
Following is the macro defined in the header string.h −
Sr.No. | Macro & Description |
---|---|
1 |
NULL This macro is the value of a null pointer constant. |
Library Functions
Following are the functions defined in the header string.h −
Sr.No. | Function & Description |
---|---|
1 |
void *memchr(const void *str, int c, size_t n)
Searches for the first occurrence of the character c (an unsigned char) in the first n bytes of the string pointed to, by the argument str. |
2 |
int memcmp(const void *str1, const void *str2, size_t n)
Compares the first n bytes of str1 and str2. |
3 |
void *memcpy(void *dest, const void *src, size_t n)
Copies n characters from src to dest. |
4 |
void *memmove(void *dest, const void *src, size_t n)
Another function to copy n characters from str2 to str1. |
5 |
void *memset(void *str, int c, size_t n)
Copies the character c (an unsigned char) to the first n characters of the string pointed to, by the argument str. |
6 |
char *strcat(char *dest, const char *src)
Appends the string pointed to, by src to the end of the string pointed to by dest. |
7 |
char *strncat(char *dest, const char *src, size_t n)
Appends the string pointed to, by src to the end of the string pointed to, by dest up to n characters long. |
8 |
char *strchr(const char *str, int c)
Searches for the first occurrence of the character c (an unsigned char) in the string pointed to, by the argument str. |
9 |
int strcmp(const char *str1, const char *str2)
Compares the string pointed to, by str1 to the string pointed to by str2. |
10 |
int strncmp(const char *str1, const char *str2, size_t n)
Compares at most the first n bytes of str1 and str2. |
11 |
int strcoll(const char *str1, const char *str2)
Compares string str1 to str2. The result is dependent on the LC_COLLATE setting of the location. |
12 |
char *strcpy(char *dest, const char *src)
Copies the string pointed to, by src to dest. |
13 |
char *strncpy(char *dest, const char *src, size_t n)
Copies up to n characters from the string pointed to, by src to dest. |
14 |
size_t strcspn(const char *str1, const char *str2)
Calculates the length of the initial segment of str1 which consists entirely of characters not in str2. |
15 |
char *strerror(int errnum)
Searches an internal array for the error number errnum and returns a pointer to an error message string. |
16 |
size_t strlen(const char *str)
Computes the length of the string str up to but not including the terminating null character. |
17 |
char *strpbrk(const char *str1, const char *str2)
Finds the first character in the string str1 that matches any character specified in str2. |
18 |
char *strrchr(const char *str, int c)
Searches for the last occurrence of the character c (an unsigned char) in the string pointed to by the argument str. |
19 |
size_t strspn(const char *str1, const char *str2)
Calculates the length of the initial segment of str1 which consists entirely of characters in str2. |
20 |
char *strstr(const char *haystack, const char *needle)
Finds the first occurrence of the entire string needle (not including the terminating null character) which appears in the string haystack. |
21 |
char *strtok(char *str, const char *delim)
Breaks string str into a series of tokens separated by delim. |
22 |
size_t strxfrm(char *dest, const char *src, size_t n)
Transforms the first n characters of the string src into current locale and places them in the string dest. |
C Library - <time.h>
The time.h header defines four variable types, two macro and various functions for manipulating date and time.
Library Variables
Following are the variable types defined in the header time.h −
Sr.No. | Variable & Description |
---|---|
1 |
size_t This is the unsigned integral type and is the result of the sizeof keyword. |
2 |
clock_t This is a type suitable for storing the processor time. |
3 |
time_t is This is a type suitable for storing the calendar time. |
4 |
struct tm This is a structure used to hold the time and date. |
The tm structure has the following definition −
struct tm { int tm_sec; /* seconds, range 0 to 59 */ int tm_min; /* minutes, range 0 to 59 */ int tm_hour; /* hours, range 0 to 23 */ int tm_mday; /* day of the month, range 1 to 31 */ int tm_mon; /* month, range 0 to 11 */ int tm_year; /* The number of years since 1900 */ int tm_wday; /* day of the week, range 0 to 6 */ int tm_yday; /* day in the year, range 0 to 365 */ int tm_isdst; /* daylight saving time */ };
Library Macros
Following are the macros defined in the header time.h −
Sr.No. | Macro & Description |
---|---|
1 |
NULL This macro is the value of a null pointer constant. |
2 |
CLOCKS_PER_SEC This macro represents the number of processor clocks per second. |
Library Functions
Following are the functions defined in the header time.h −
Sr.No. | Function & Description |
---|---|
1 |
char *asctime(const struct tm *timeptr)
Returns a pointer to a string which represents the day and time of the structure timeptr. |
2 |
clock_t clock(void)
Returns the processor clock time used since the beginning of an implementation defined era (normally the beginning of the program). |
3 |
char *ctime(const time_t *timer)
Returns a string representing the localtime based on the argument timer. |
4 |
double difftime(time_t time1, time_t time2)
Returns the difference of seconds between time1 and time2 (time1-time2). |
5 |
struct tm *gmtime(const time_t *timer)
The value of timer is broken up into the structure tm and expressed in Coordinated Universal Time (UTC) also known as Greenwich Mean Time (GMT). |
6 |
struct tm *localtime(const time_t *timer)
The value of timer is broken up into the structure tm and expressed in the local time zone. |
7 |
time_t mktime(struct tm *timeptr)
Converts the structure pointed to by timeptr into a time_t value according to the local time zone. |
8 |
size_t strftime(char *str, size_t maxsize, const char *format, const struct tm *timeptr)
Formats the time represented in the structure timeptr according to the formatting rules defined in format and stored into str. |
9 |
time_t time(time_t *timer)
Calculates the current calender time and encodes it into time_t format. |