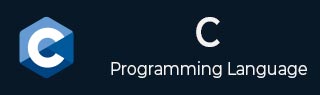
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <float.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdarg.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <time.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library function - feof()
Description
The C library function int feof(FILE *stream) tests the end-of-file indicator for the given stream.
Declaration
Following is the declaration for feof() function.
int feof(FILE *stream)
Parameters
stream − This is the pointer to a FILE object that identifies the stream.
Return Value
This function returns a non-zero value when End-of-File indicator associated with the stream is set, else zero is returned.
Example
The following example shows the usage of feof() function.
#include <stdio.h> int main () { FILE *fp; int c; fp = fopen("file.txt","r"); if(fp == NULL) { perror("Error in opening file"); return(-1); } while(1) { c = fgetc(fp); if( feof(fp) ) { break ; } printf("%c", c); } fclose(fp); return(0); }
Assuming we have a text file file.txt, which has the following content. This file will be used as an input for our example program −
This is tutorialspoint.com
Let us compile and run the above program, this will produce the following result −
This is tutorialspoint.com
stdio_h.htm
Advertisements