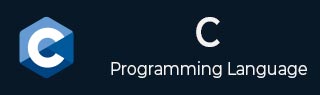
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C Library - sprintf() function
The C Library sprintf() function allows you to create strings with specified formats, similar to printf(), but instead of printing to the standard output, it stores the resulting string in a character array provided by the user.
Syntax
Following is the C library syntax of the sprintf() function −
int sprintf(char *str, const char *format, ...);
Parameters
This function accepts the following parameters −
- str : A pointer to an array of characters where the resulting string will be stored.
- format : A pointer to a null-terminated string that contains the text to be written to the string str. This string may contain format specifiers that dictate how subsequent arguments are converted and formatted into the resulting string.
Return Value
The sprintf() function returns the number of characters written to the string str, excluding the null-terminating character.
Example 1: Formatting Floating Point Numbers
Here, sprintf() formats a floating-point number with two decimal places.
Below is the illustration of the C library sprintf() function.
#include <stdio.h> int main() { char buffer[100]; float pi = 3.14159; sprintf(buffer, "The value of pi is %.2f.", pi); printf("%s\n", buffer); return 0; }
Output
The above code produces following result−
The value of pi is 3.14.
Example 2: Combining Strings and Integers
This example showcases how sprintf() can combine strings and integers into a single formatted string.
#include <stdio.h> int main() { char buffer[100]; int age = 30; char name[] = "John"; sprintf(buffer, "%s is %d years old.", name, age); printf("%s\n", buffer); return 0; }
Output
After execution of above code, we get the following result −
John is 30 years old.