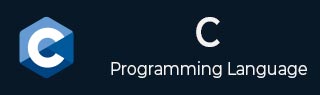
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - system() function
The C stdlib library system() function is used to execute an operating system command specified by the string 'command' from a c/c++ program. It returns an integer status indicating the success or failure of the command execution.
This function is OS-dependent. We use 'system("dir")' on Windows and 'system("ls")' on Unix to list the directory contents.
Note: This function lists the files of the current directory when compiled on our system. If compiled using an online compiler, it will only show 'main main.c'.
Syntax
Following is the syntax of the system() function −
int system(const char *string)
Parameters
This function accepts a single parameter −
string − it represents a pointer to a null-terminated string contains a command we want to execute.
Return Value
This function returns 0 if the command is executed successfully. Otherwise, it returns a non-zero value.
Example 1
In this example, we create a basic c program to demonstrate the use of system() function.
#include <stdio.h> #include <string.h> #include <stdlib.h> int main() { char command[50]; // Set the command to "dir" (list files and directories) strcpy(command, "dir"); //use the system fucntion to execute the command. system(command); return 0; }
Output
Following is the output, which shows the list of current directory −
05/10/2024 05:26 PM <DIR> . 05/10/2024 03:01 PM <DIR> .. 05/09/2024 01:57 PM 238 abort.c 05/09/2024 01:57 PM 131,176 abort.exe 05/09/2024 02:10 PM 412 abort2.c 05/09/2024 02:13 PM 131,175 abort2.exe 05/09/2024 02:23 PM 354 abort3.c 05/09/2024 02:23 PM 131,861 abort3.exe 05/09/2024 04:56 PM 399 atexit1.c 05/09/2024 04:56 PM 132,057 atexit1.exe 05/09/2024 05:30 PM 603 atexit2.c 05/09/2024 05:09 PM 132,078 atexit2.exe 05/09/2024 05:35 PM 612 atexit3.c 05/09/2024 05:35 PM 132,235 atexit3.exe 05/07/2024 11:01 AM 182 atof.cpp 05/07/2024 11:01 AM 131,175 atof.exe . . .
Example 2
Let's create another c program and use the system() function to run an external command (the echo command).
#include <stdio.h> #include <string.h> int main() { char command[50]; // Set the command to print a message strcpy(command, "echo Hello, World!"); // Execute the command system(command); return 0; }
Output
Following is the output −
Hello, World!